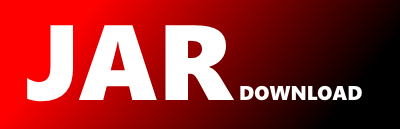
net.isger.brick.plugin.service.Services Maven / Gradle / Ivy
The newest version!
package net.isger.brick.plugin.service;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import net.isger.util.Helpers;
import net.isger.util.Strings;
public class Services {
private static final Logger LOG;
private Map services;
static {
LOG = LoggerFactory.getLogger(Services.class);
}
public Services() {
this(null);
}
@SuppressWarnings("unchecked")
public Services(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy