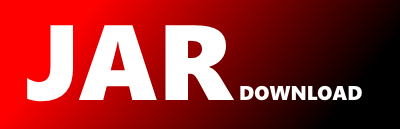
net.israfil.service.mail.api.smtp.SMTPAddress Maven / Gradle / Ivy
The newest version!
package net.israfil.service.mail.api.smtp;
import net.israfil.service.mail.api.MailAddress;
/**
* A MailAddress that represents an immutable well-formed SMTP
* e-mail address in compliance witha subset of RFC2822 Paragraph
* 3.4 specifying addresses. In other words, this class will
* render to a compliant address, but not all compliant addresses
* may be represented by this class. For example, this class
* imposes an 800character limit on the address to allow for
* at least one address to be on a <1000 line per the RFC2822
* spec, though that is a line limit for headers, not addresses
* per-se. Additionally, only ASCII or ASCII-subsets of other
* encodings are permitted.
*
* @author cgruber
*
*/
public class SMTPAddress implements MailAddress{
private final String displayName;
private final String local;
private final String domain;
public SMTPAddress(String address) {
this(null,address);
}
public SMTPAddress(String displayName, String address) {
this(displayName,SMTPUtils.getLocal(address),SMTPUtils.getDomain(address));
}
public SMTPAddress(String displayName, String local, String domain) {
this.displayName = (displayName == null || "".equals(displayName)) ?
null :
SMTPUtils.assertASCII(displayName);
if (local == null ||"".equals(local))
throw new IllegalArgumentException("SMTP must be constructed with at least a local address.");
this.local = SMTPUtils.assertASCII(local);
this.domain = (domain == null || "".equals(domain)) ?
null :
SMTPUtils.assertASCII(domain);
}
public String getDisplayName() {
return displayName;
}
public String getAddress() {
if (domain == null) return local;
else return String.format("%s@%s", local, domain);
}
public String getCanonicalAddress() {
if (displayName == null) {
if (domain == null) {
return String.format("<%s>", local);
} else {
return String.format("<%s@%s>", local, domain);
}
} else {
if (domain == null) {
return String.format("\"%s\" <%s>", displayName, local);
} else {
return String.format("\"%s\" <%s@%s>", displayName, local, domain);
}
}
}
@Override
public String toString() {
return String.format("%s[%s]",this.getClass().getSimpleName(),getCanonicalAddress());
}
public String getType() { return SMTPTransport.TRANSPORT_TYPE; }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy