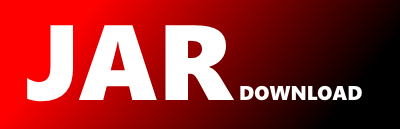
net.israfil.service.mail.api.smtp.SMTPUtils Maven / Gradle / Ivy
The newest version!
package net.israfil.service.mail.api.smtp;
import java.util.Collection;
import net.israfil.service.mail.api.MailHeader;
public abstract class SMTPUtils {
/**
* Takes a string and asserts that all characters are ASCII.
*/
public static String assertASCII(final String string) {
int length;
if (string == null || (length = string.length()) == 0) return string;
char[] chars = new char[length];
string.getChars(0, length-1, chars,0);
for (int i = 0; i < length; i++) {
if (chars[i] > 127)
throw new IllegalArgumentException("String contains non-ASCII characters: " + string);
}
return string;
}
public static String getLocal(String address) {
if (address == null) throw new IllegalArgumentException("SMTP must be constructed with at least a local address.");
if (!address.contains("@")) return address;
else {
String local = address.split("@")[0];
return (local == null || local.equals("")) ? null : local;
}
}
public static String getDomain(String address) {
if (address == null) throw new IllegalArgumentException("SMTP must be constructed with at least a local address.");
if (!address.contains("@")) return null;
else {
String[] parts = address.split("@");
return (parts.length > 1) ? parts[1] : null;
}
}
@SuppressWarnings("unchecked")
public static void validateSpecialHeader(SMTPHeaderTypes type,MailHeader> header) {
if (type == null) return;
if (header == null) throw new IllegalArgumentException("Error attempting to set a null value as a header object.");
switch(type) {
case Bcc:
case To:
case Cc:
try {
Collection contents = (Collection)header.getContent();
for (@SuppressWarnings("unused") SMTPAddress address : contents);
} catch (ClassCastException cce) {
throw new IllegalArgumentException("Header " + type + " does not contain a valid collection of SMTPAddress instances");
}
break;
case Subject:
try {
@SuppressWarnings("unused")
String contents = (String)header.getContent();
} catch (ClassCastException cce) {
throw new IllegalArgumentException("Header " + type + " does not contain a valid collection of SMTPAddress instances");
}
break;
case From:
try {
@SuppressWarnings("unused")
SMTPAddress contents = (SMTPAddress)header.getContent();
} catch (ClassCastException cce) {
throw new IllegalArgumentException("Header " + type + " does not contain a valid collection of SMTPAddress instances");
}
break;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy