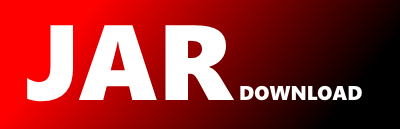
net.jacobpeterson.domain.alpaca.order.Order Maven / Gradle / Ivy
Show all versions of alpaca-java Show documentation
package net.jacobpeterson.domain.alpaca.order;
import java.io.Serializable;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
/**
* See https://docs.alpaca.markets/api-documentation/api-v2/orders/
*
*
*
*/
public class Order implements Serializable
{
/**
* Order ID
*
*
*
*/
@SerializedName("id")
@Expose
private String id;
/**
* Client unique order ID
*
*
*
*/
@SerializedName("client_order_id")
@Expose
private String clientOrderId;
/**
* Created at
*
*
*
*/
@SerializedName("created_at")
@Expose
private ZonedDateTime createdAt;
/**
* Updated at
*
*
*
*/
@SerializedName("updated_at")
@Expose
private ZonedDateTime updatedAt;
/**
* Submitted at
*
*
*
*/
@SerializedName("submitted_at")
@Expose
private ZonedDateTime submittedAt;
/**
* Filled at
*
*
*
*/
@SerializedName("filled_at")
@Expose
private ZonedDateTime filledAt;
/**
* Expired at
*
*
*
*/
@SerializedName("expired_at")
@Expose
private ZonedDateTime expiredAt;
/**
* Canceled at
*
*
*
*/
@SerializedName("canceled_at")
@Expose
private ZonedDateTime canceledAt;
/**
* Failed at
*
*
*
*/
@SerializedName("failed_at")
@Expose
private ZonedDateTime failedAt;
/**
* Replaced at
*
*
*
*/
@SerializedName("replaced_at")
@Expose
private ZonedDateTime replacedAt;
/**
* The order ID that this order was replaced by
*
*
*
*/
@SerializedName("replaced_by")
@Expose
private String replacedBy;
/**
* The order ID that this order replaces
*
*
*
*/
@SerializedName("replaces")
@Expose
private String replaces;
/**
* Asset ID
*
*
*
*/
@SerializedName("asset_id")
@Expose
private String assetId;
/**
* Asset symbol
*
*
*
*/
@SerializedName("symbol")
@Expose
private String symbol;
/**
* Asset class
*
*
*
*/
@SerializedName("asset_class")
@Expose
private String assetClass;
/**
* Ordered quantity
*
*
*
*/
@SerializedName("qty")
@Expose
private String qty;
/**
* Filled quantity
*
*
*
*/
@SerializedName("filled_qty")
@Expose
private String filledQty;
/**
* Valid values: market, limit, stop, stop_limit
*
*
*
*/
@SerializedName("type")
@Expose
private String type;
/**
* Valid values: buy, sell
*
*
*
*/
@SerializedName("side")
@Expose
private String side;
/**
* See Orders page
*
*
*
*/
@SerializedName("time_in_force")
@Expose
private String timeInForce;
/**
* Limit price
*
*
*
*/
@SerializedName("limit_price")
@Expose
private String limitPrice;
/**
* Stop price
*
*
*
*/
@SerializedName("stop_price")
@Expose
private String stopPrice;
/**
* Stop price
*
*
*
*/
@SerializedName("filled_avg_price")
@Expose
private String filledAvgPrice;
/**
* See Orders page
*
*
*
*/
@SerializedName("status")
@Expose
private String status;
/**
* If true, eligible for execution outside regular trading hours.
*
*
*
*/
@SerializedName("extended_hours")
@Expose
private Boolean extendedHours;
/**
* When querying non-simple order_class orders in a nested style, an array of Order entities associated with this order is returned. Otherwise, null.
*
*
*
*/
@SerializedName("legs")
@Expose
private ArrayList legs;
private final static long serialVersionUID = 929662062072421910L;
/**
* No args constructor for use in serialization
*
*/
public Order() {
}
/**
*
* @param symbol
* @param replacedAt
* @param extendedHours
* @param assetClass
* @param type
* @param createdAt
* @param expiredAt
* @param failedAt
* @param assetId
* @param legs
* @param id
* @param submittedAt
* @param timeInForce
* @param updatedAt
* @param side
* @param limitPrice
* @param replacedBy
* @param replaces
* @param clientOrderId
* @param filledAt
* @param filledAvgPrice
* @param stopPrice
* @param canceledAt
* @param qty
* @param filledQty
* @param status
*/
public Order(String id, String clientOrderId, ZonedDateTime createdAt, ZonedDateTime updatedAt, ZonedDateTime submittedAt, ZonedDateTime filledAt, ZonedDateTime expiredAt, ZonedDateTime canceledAt, ZonedDateTime failedAt, ZonedDateTime replacedAt, String replacedBy, String replaces, String assetId, String symbol, String assetClass, String qty, String filledQty, String type, String side, String timeInForce, String limitPrice, String stopPrice, String filledAvgPrice, String status, Boolean extendedHours, ArrayList legs) {
super();
this.id = id;
this.clientOrderId = clientOrderId;
this.createdAt = createdAt;
this.updatedAt = updatedAt;
this.submittedAt = submittedAt;
this.filledAt = filledAt;
this.expiredAt = expiredAt;
this.canceledAt = canceledAt;
this.failedAt = failedAt;
this.replacedAt = replacedAt;
this.replacedBy = replacedBy;
this.replaces = replaces;
this.assetId = assetId;
this.symbol = symbol;
this.assetClass = assetClass;
this.qty = qty;
this.filledQty = filledQty;
this.type = type;
this.side = side;
this.timeInForce = timeInForce;
this.limitPrice = limitPrice;
this.stopPrice = stopPrice;
this.filledAvgPrice = filledAvgPrice;
this.status = status;
this.extendedHours = extendedHours;
this.legs = legs;
}
/**
* Order ID
*
*
*
*/
public String getId() {
return id;
}
/**
* Order ID
*
*
*
*/
public void setId(String id) {
this.id = id;
}
/**
* Client unique order ID
*
*
*
*/
public String getClientOrderId() {
return clientOrderId;
}
/**
* Client unique order ID
*
*
*
*/
public void setClientOrderId(String clientOrderId) {
this.clientOrderId = clientOrderId;
}
/**
* Created at
*
*
*
*/
public ZonedDateTime getCreatedAt() {
return createdAt;
}
/**
* Created at
*
*
*
*/
public void setCreatedAt(ZonedDateTime createdAt) {
this.createdAt = createdAt;
}
/**
* Updated at
*
*
*
*/
public ZonedDateTime getUpdatedAt() {
return updatedAt;
}
/**
* Updated at
*
*
*
*/
public void setUpdatedAt(ZonedDateTime updatedAt) {
this.updatedAt = updatedAt;
}
/**
* Submitted at
*
*
*
*/
public ZonedDateTime getSubmittedAt() {
return submittedAt;
}
/**
* Submitted at
*
*
*
*/
public void setSubmittedAt(ZonedDateTime submittedAt) {
this.submittedAt = submittedAt;
}
/**
* Filled at
*
*
*
*/
public ZonedDateTime getFilledAt() {
return filledAt;
}
/**
* Filled at
*
*
*
*/
public void setFilledAt(ZonedDateTime filledAt) {
this.filledAt = filledAt;
}
/**
* Expired at
*
*
*
*/
public ZonedDateTime getExpiredAt() {
return expiredAt;
}
/**
* Expired at
*
*
*
*/
public void setExpiredAt(ZonedDateTime expiredAt) {
this.expiredAt = expiredAt;
}
/**
* Canceled at
*
*
*
*/
public ZonedDateTime getCanceledAt() {
return canceledAt;
}
/**
* Canceled at
*
*
*
*/
public void setCanceledAt(ZonedDateTime canceledAt) {
this.canceledAt = canceledAt;
}
/**
* Failed at
*
*
*
*/
public ZonedDateTime getFailedAt() {
return failedAt;
}
/**
* Failed at
*
*
*
*/
public void setFailedAt(ZonedDateTime failedAt) {
this.failedAt = failedAt;
}
/**
* Replaced at
*
*
*
*/
public ZonedDateTime getReplacedAt() {
return replacedAt;
}
/**
* Replaced at
*
*
*
*/
public void setReplacedAt(ZonedDateTime replacedAt) {
this.replacedAt = replacedAt;
}
/**
* The order ID that this order was replaced by
*
*
*
*/
public String getReplacedBy() {
return replacedBy;
}
/**
* The order ID that this order was replaced by
*
*
*
*/
public void setReplacedBy(String replacedBy) {
this.replacedBy = replacedBy;
}
/**
* The order ID that this order replaces
*
*
*
*/
public String getReplaces() {
return replaces;
}
/**
* The order ID that this order replaces
*
*
*
*/
public void setReplaces(String replaces) {
this.replaces = replaces;
}
/**
* Asset ID
*
*
*
*/
public String getAssetId() {
return assetId;
}
/**
* Asset ID
*
*
*
*/
public void setAssetId(String assetId) {
this.assetId = assetId;
}
/**
* Asset symbol
*
*
*
*/
public String getSymbol() {
return symbol;
}
/**
* Asset symbol
*
*
*
*/
public void setSymbol(String symbol) {
this.symbol = symbol;
}
/**
* Asset class
*
*
*
*/
public String getAssetClass() {
return assetClass;
}
/**
* Asset class
*
*
*
*/
public void setAssetClass(String assetClass) {
this.assetClass = assetClass;
}
/**
* Ordered quantity
*
*
*
*/
public String getQty() {
return qty;
}
/**
* Ordered quantity
*
*
*
*/
public void setQty(String qty) {
this.qty = qty;
}
/**
* Filled quantity
*
*
*
*/
public String getFilledQty() {
return filledQty;
}
/**
* Filled quantity
*
*
*
*/
public void setFilledQty(String filledQty) {
this.filledQty = filledQty;
}
/**
* Valid values: market, limit, stop, stop_limit
*
*
*
*/
public String getType() {
return type;
}
/**
* Valid values: market, limit, stop, stop_limit
*
*
*
*/
public void setType(String type) {
this.type = type;
}
/**
* Valid values: buy, sell
*
*
*
*/
public String getSide() {
return side;
}
/**
* Valid values: buy, sell
*
*
*
*/
public void setSide(String side) {
this.side = side;
}
/**
* See Orders page
*
*
*
*/
public String getTimeInForce() {
return timeInForce;
}
/**
* See Orders page
*
*
*
*/
public void setTimeInForce(String timeInForce) {
this.timeInForce = timeInForce;
}
/**
* Limit price
*
*
*
*/
public String getLimitPrice() {
return limitPrice;
}
/**
* Limit price
*
*
*
*/
public void setLimitPrice(String limitPrice) {
this.limitPrice = limitPrice;
}
/**
* Stop price
*
*
*
*/
public String getStopPrice() {
return stopPrice;
}
/**
* Stop price
*
*
*
*/
public void setStopPrice(String stopPrice) {
this.stopPrice = stopPrice;
}
/**
* Stop price
*
*
*
*/
public String getFilledAvgPrice() {
return filledAvgPrice;
}
/**
* Stop price
*
*
*
*/
public void setFilledAvgPrice(String filledAvgPrice) {
this.filledAvgPrice = filledAvgPrice;
}
/**
* See Orders page
*
*
*
*/
public String getStatus() {
return status;
}
/**
* See Orders page
*
*
*
*/
public void setStatus(String status) {
this.status = status;
}
/**
* If true, eligible for execution outside regular trading hours.
*
*
*
*/
public Boolean getExtendedHours() {
return extendedHours;
}
/**
* If true, eligible for execution outside regular trading hours.
*
*
*
*/
public void setExtendedHours(Boolean extendedHours) {
this.extendedHours = extendedHours;
}
/**
* When querying non-simple order_class orders in a nested style, an array of Order entities associated with this order is returned. Otherwise, null.
*
*
*
*/
public ArrayList getLegs() {
return legs;
}
/**
* When querying non-simple order_class orders in a nested style, an array of Order entities associated with this order is returned. Otherwise, null.
*
*
*
*/
public void setLegs(ArrayList legs) {
this.legs = legs;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(Order.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("id");
sb.append('=');
sb.append(((this.id == null)?"":this.id));
sb.append(',');
sb.append("clientOrderId");
sb.append('=');
sb.append(((this.clientOrderId == null)?"":this.clientOrderId));
sb.append(',');
sb.append("createdAt");
sb.append('=');
sb.append(((this.createdAt == null)?"":this.createdAt));
sb.append(',');
sb.append("updatedAt");
sb.append('=');
sb.append(((this.updatedAt == null)?"":this.updatedAt));
sb.append(',');
sb.append("submittedAt");
sb.append('=');
sb.append(((this.submittedAt == null)?"":this.submittedAt));
sb.append(',');
sb.append("filledAt");
sb.append('=');
sb.append(((this.filledAt == null)?"":this.filledAt));
sb.append(',');
sb.append("expiredAt");
sb.append('=');
sb.append(((this.expiredAt == null)?"":this.expiredAt));
sb.append(',');
sb.append("canceledAt");
sb.append('=');
sb.append(((this.canceledAt == null)?"":this.canceledAt));
sb.append(',');
sb.append("failedAt");
sb.append('=');
sb.append(((this.failedAt == null)?"":this.failedAt));
sb.append(',');
sb.append("replacedAt");
sb.append('=');
sb.append(((this.replacedAt == null)?"":this.replacedAt));
sb.append(',');
sb.append("replacedBy");
sb.append('=');
sb.append(((this.replacedBy == null)?"":this.replacedBy));
sb.append(',');
sb.append("replaces");
sb.append('=');
sb.append(((this.replaces == null)?"":this.replaces));
sb.append(',');
sb.append("assetId");
sb.append('=');
sb.append(((this.assetId == null)?"":this.assetId));
sb.append(',');
sb.append("symbol");
sb.append('=');
sb.append(((this.symbol == null)?"":this.symbol));
sb.append(',');
sb.append("assetClass");
sb.append('=');
sb.append(((this.assetClass == null)?"":this.assetClass));
sb.append(',');
sb.append("qty");
sb.append('=');
sb.append(((this.qty == null)?"":this.qty));
sb.append(',');
sb.append("filledQty");
sb.append('=');
sb.append(((this.filledQty == null)?"":this.filledQty));
sb.append(',');
sb.append("type");
sb.append('=');
sb.append(((this.type == null)?"":this.type));
sb.append(',');
sb.append("side");
sb.append('=');
sb.append(((this.side == null)?"":this.side));
sb.append(',');
sb.append("timeInForce");
sb.append('=');
sb.append(((this.timeInForce == null)?"":this.timeInForce));
sb.append(',');
sb.append("limitPrice");
sb.append('=');
sb.append(((this.limitPrice == null)?"":this.limitPrice));
sb.append(',');
sb.append("stopPrice");
sb.append('=');
sb.append(((this.stopPrice == null)?"":this.stopPrice));
sb.append(',');
sb.append("filledAvgPrice");
sb.append('=');
sb.append(((this.filledAvgPrice == null)?"":this.filledAvgPrice));
sb.append(',');
sb.append("status");
sb.append('=');
sb.append(((this.status == null)?"":this.status));
sb.append(',');
sb.append("extendedHours");
sb.append('=');
sb.append(((this.extendedHours == null)?"":this.extendedHours));
sb.append(',');
sb.append("legs");
sb.append('=');
sb.append(((this.legs == null)?"":this.legs));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.symbol == null)? 0 :this.symbol.hashCode()));
result = ((result* 31)+((this.replacedAt == null)? 0 :this.replacedAt.hashCode()));
result = ((result* 31)+((this.extendedHours == null)? 0 :this.extendedHours.hashCode()));
result = ((result* 31)+((this.assetClass == null)? 0 :this.assetClass.hashCode()));
result = ((result* 31)+((this.type == null)? 0 :this.type.hashCode()));
result = ((result* 31)+((this.createdAt == null)? 0 :this.createdAt.hashCode()));
result = ((result* 31)+((this.expiredAt == null)? 0 :this.expiredAt.hashCode()));
result = ((result* 31)+((this.failedAt == null)? 0 :this.failedAt.hashCode()));
result = ((result* 31)+((this.assetId == null)? 0 :this.assetId.hashCode()));
result = ((result* 31)+((this.legs == null)? 0 :this.legs.hashCode()));
result = ((result* 31)+((this.id == null)? 0 :this.id.hashCode()));
result = ((result* 31)+((this.submittedAt == null)? 0 :this.submittedAt.hashCode()));
result = ((result* 31)+((this.timeInForce == null)? 0 :this.timeInForce.hashCode()));
result = ((result* 31)+((this.updatedAt == null)? 0 :this.updatedAt.hashCode()));
result = ((result* 31)+((this.side == null)? 0 :this.side.hashCode()));
result = ((result* 31)+((this.limitPrice == null)? 0 :this.limitPrice.hashCode()));
result = ((result* 31)+((this.replacedBy == null)? 0 :this.replacedBy.hashCode()));
result = ((result* 31)+((this.replaces == null)? 0 :this.replaces.hashCode()));
result = ((result* 31)+((this.clientOrderId == null)? 0 :this.clientOrderId.hashCode()));
result = ((result* 31)+((this.filledAt == null)? 0 :this.filledAt.hashCode()));
result = ((result* 31)+((this.filledAvgPrice == null)? 0 :this.filledAvgPrice.hashCode()));
result = ((result* 31)+((this.stopPrice == null)? 0 :this.stopPrice.hashCode()));
result = ((result* 31)+((this.canceledAt == null)? 0 :this.canceledAt.hashCode()));
result = ((result* 31)+((this.qty == null)? 0 :this.qty.hashCode()));
result = ((result* 31)+((this.filledQty == null)? 0 :this.filledQty.hashCode()));
result = ((result* 31)+((this.status == null)? 0 :this.status.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof Order) == false) {
return false;
}
Order rhs = ((Order) other);
return (((((((((((((((((((((((((((this.symbol == rhs.symbol)||((this.symbol!= null)&&this.symbol.equals(rhs.symbol)))&&((this.replacedAt == rhs.replacedAt)||((this.replacedAt!= null)&&this.replacedAt.equals(rhs.replacedAt))))&&((this.extendedHours == rhs.extendedHours)||((this.extendedHours!= null)&&this.extendedHours.equals(rhs.extendedHours))))&&((this.assetClass == rhs.assetClass)||((this.assetClass!= null)&&this.assetClass.equals(rhs.assetClass))))&&((this.type == rhs.type)||((this.type!= null)&&this.type.equals(rhs.type))))&&((this.createdAt == rhs.createdAt)||((this.createdAt!= null)&&this.createdAt.equals(rhs.createdAt))))&&((this.expiredAt == rhs.expiredAt)||((this.expiredAt!= null)&&this.expiredAt.equals(rhs.expiredAt))))&&((this.failedAt == rhs.failedAt)||((this.failedAt!= null)&&this.failedAt.equals(rhs.failedAt))))&&((this.assetId == rhs.assetId)||((this.assetId!= null)&&this.assetId.equals(rhs.assetId))))&&((this.legs == rhs.legs)||((this.legs!= null)&&this.legs.equals(rhs.legs))))&&((this.id == rhs.id)||((this.id!= null)&&this.id.equals(rhs.id))))&&((this.submittedAt == rhs.submittedAt)||((this.submittedAt!= null)&&this.submittedAt.equals(rhs.submittedAt))))&&((this.timeInForce == rhs.timeInForce)||((this.timeInForce!= null)&&this.timeInForce.equals(rhs.timeInForce))))&&((this.updatedAt == rhs.updatedAt)||((this.updatedAt!= null)&&this.updatedAt.equals(rhs.updatedAt))))&&((this.side == rhs.side)||((this.side!= null)&&this.side.equals(rhs.side))))&&((this.limitPrice == rhs.limitPrice)||((this.limitPrice!= null)&&this.limitPrice.equals(rhs.limitPrice))))&&((this.replacedBy == rhs.replacedBy)||((this.replacedBy!= null)&&this.replacedBy.equals(rhs.replacedBy))))&&((this.replaces == rhs.replaces)||((this.replaces!= null)&&this.replaces.equals(rhs.replaces))))&&((this.clientOrderId == rhs.clientOrderId)||((this.clientOrderId!= null)&&this.clientOrderId.equals(rhs.clientOrderId))))&&((this.filledAt == rhs.filledAt)||((this.filledAt!= null)&&this.filledAt.equals(rhs.filledAt))))&&((this.filledAvgPrice == rhs.filledAvgPrice)||((this.filledAvgPrice!= null)&&this.filledAvgPrice.equals(rhs.filledAvgPrice))))&&((this.stopPrice == rhs.stopPrice)||((this.stopPrice!= null)&&this.stopPrice.equals(rhs.stopPrice))))&&((this.canceledAt == rhs.canceledAt)||((this.canceledAt!= null)&&this.canceledAt.equals(rhs.canceledAt))))&&((this.qty == rhs.qty)||((this.qty!= null)&&this.qty.equals(rhs.qty))))&&((this.filledQty == rhs.filledQty)||((this.filledQty!= null)&&this.filledQty.equals(rhs.filledQty))))&&((this.status == rhs.status)||((this.status!= null)&&this.status.equals(rhs.status))));
}
}