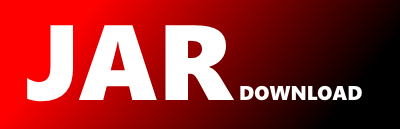
net.jangaroo.exml.generator.ExmlConfigPackage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of exml-compiler Show documentation
Show all versions of exml-compiler Show documentation
parses an EXML and generates an AS config class
package net.jangaroo.exml.generator;
import net.jangaroo.exml.model.ConfigClass;
import net.jangaroo.exml.model.ExmlElement;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
*
*/
public class ExmlConfigPackage {
private static final Comparator EXML_ELEMENT_BY_NAME_COMPARATOR = new Comparator() {
@Override
public int compare(ExmlElement ee1, ExmlElement ee2) {
return ee1.getName().compareTo(ee2.getName());
}
};
private List exmlElements;
private Map usedNamespaces;
private String packageName;
private String ns;
public ExmlConfigPackage(Collection cl, String packageName) {
this.packageName = packageName;
ns = calcNsFromPackageName(packageName, 1);
exmlElements = new ArrayList(cl.size());
for (ConfigClass configClass : cl) {
exmlElements.add(new ExmlElement(configClass));
}
computeShortNamespaces();
Collections.sort(exmlElements, EXML_ELEMENT_BY_NAME_COMPARATOR);
}
private void computeShortNamespaces() {
usedNamespaces = new HashMap();
for (ExmlElement ee : exmlElements) {
calcNsFromPackage(ee);
ExmlElement superElement = ee.getSuperElement();
if (superElement != null) {
calcNsFromPackage(superElement);
}
}
}
private void calcNsFromPackage(ExmlElement exmlElement) {
String packageName = exmlElement.getPackage();
String shortNamespace;
if (this.packageName.equals(packageName)) {
shortNamespace = ns; // do not add to usedNamespaces, as freemarker template cares about this separately!
} else {
shortNamespace = usedNamespaces.get(packageName);
if (shortNamespace == null) {
if (packageName.length() == 0) {
shortNamespace = "top"; // should only occur in tests or demo code!
} else {
for (int n = 1; ; n++) {
shortNamespace = calcNsFromPackageName(packageName, n);
if (!usedNamespaces.values().contains(shortNamespace)) {
break;
}
}
}
usedNamespaces.put(packageName, shortNamespace);
}
}
exmlElement.setNs(shortNamespace);
}
private String calcNsFromPackageName(String packageName, int n) {
if (packageName.length() == 0) {
return "t";
}
String shortNamespace;
String[] parts = packageName.split("\\.");
StringBuilder ns = new StringBuilder();
for (String part : parts) {
ns.append(part.substring(0, n));
}
shortNamespace = ns.toString();
return shortNamespace;
}
public String getNs() {
return ns;
}
public String getPackageName() {
return packageName;
}
public List getExmlElements() {
return exmlElements;
}
public Map getUsedNamespaces() {
return usedNamespaces;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy