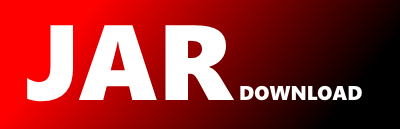
net.java.ao.benchmark.util.StopWatch Maven / Gradle / Ivy
package net.java.ao.benchmark.util;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.SortedMap;
import java.util.TreeMap;
public final class StopWatch {
private final SortedMap laps = new TreeMap();
private final String name;
private long start;
private long stop;
private long lap;
public StopWatch(String name) {
this.name = Objects.requireNonNull(name, "name can't be null");
}
public String getName() {
return name;
}
public void start() {
start = now();
lap = start;
laps.clear();
}
public void lap(K k) {
final long now = now();
laps.put(k, now - lap);
lap = now;
}
public void stop() {
stop = now();
}
private long now() {
return System.nanoTime();
}
private boolean isStopped() {
return stop > 0;
}
public Report getReport() {
if (!isStopped()) {
throw new IllegalStateException("Stop watch must be stopped to generate report");
}
return new Report(name, start, stop, laps());
}
private List laps() {
final List lapValues = new ArrayList(laps.size());
for (Map.Entry e : laps.entrySet()) {
lapValues.add(e.getValue());
}
return lapValues;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy