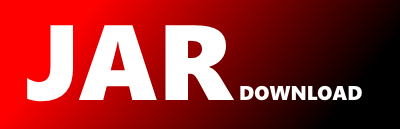
org.jdesktop.el.MethodExpression Maven / Gradle / Ivy
/*
* Copyright (C) 2007 Sun Microsystems, Inc. All rights reserved. Use is
* subject to license terms.
*/
package org.jdesktop.el;
/**
* An Expression
that refers to a method on an object.
*
* The {@link ExpressionFactory#createMethodExpression} method
* can be used to parse an expression string and return a concrete instance
* of MethodExpression
that encapsulates the parsed expression.
* The {@link FunctionMapper} is used at parse time, not evaluation time,
* so one is not needed to evaluate an expression using this class.
* However, the {@link ELContext} is needed at evaluation time.
*
* The {@link #getMethodInfo} and {@link #invoke} methods will evaluate the
* expression each time they are called. The {@link ELResolver} in the
* ELContext
is used to resolve the top-level variables and to
* determine the behavior of the .
and []
* operators. For any of the two methods, the {@link ELResolver#getValue}
* method is used to resolve all properties up to but excluding the last
* one. This provides the base
object on which the method
* appears. If the base
object is null, a
* PropertyNotFoundException
must be thrown.
* At the last resolution,
* the final property
is then coerced to a String
,
* which provides the name of the method to be found. A method matching the
* name and expected parameters provided at parse time is found and it is
* either queried or invoked (depending on the method called on this
* MethodExpression
).
*
* See the notes about comparison, serialization and immutability in
* the {@link Expression} javadocs.
*
* @see ELResolver
* @see Expression
* @see ExpressionFactory
* @since JSP 2.1
*/
public abstract class MethodExpression extends Expression
{
// Evaluation
/**
* Evaluates the expression relative to the provided context, and
* returns information about the actual referenced method.
*
* @param context The context of this evaluation
* @return an instance of MethodInfo
containing information
* about the method the expression evaluated to.
* @throws NullPointerException if context is null
* @throws PropertyNotFoundException if one of the property
* resolutions failed because a specified variable or property
* does not exist or is not readable.
* @throws MethodNotFoundException if no suitable method can be found.
* @throws ELException if an exception was thrown while performing
* property or variable resolution. The thrown exception
* must be included as the cause property of this exception, if
* available.
*/
public abstract MethodInfo getMethodInfo(ELContext context);
/**
* If a String literal is specified as the expression, returns the
* String literal coerced to the expected return type of the method
* signature. An ELException
is thrown if
* expectedReturnType
is void or if the coercion of the String literal
* to the expectedReturnType
yields an error (see Section "1.16 Type
* Conversion" of the EL specification).
*
* If not a String literal, evaluates the expression
* relative to the provided context, invokes the method that was
* found using the supplied parameters, and returns the result of
* the method invocation.
*
* Any parameters passed to this method is ignored if isLiteralText()
* is true.
*
* @param context The context of this evaluation.
* @param params The parameters to pass to the method, or
* null
if no parameters.
* @return the result of the method invocation (null
if
* the method has a void
return type).
* @throws NullPointerException if context is null
* @throws PropertyNotFoundException if one of the property
* resolutions failed because a specified variable or property
* does not exist or is not readable.
* @throws MethodNotFoundException if no suitable method can be found.
* @throws ELException if a String literal is specified and
* expectedReturnType of the MethodExpression is void or if the coercion of the String literal
* to the expectedReturnType yields an error (see Section "1.16 Type
* Conversion").
* @throws ELException if
* an exception was thrown while performing
* property or variable resolution. The thrown exception must be
* included as the cause property of this exception, if
* available. If the exception thrown is an
* InvocationTargetException
, extract its
* cause
and pass it to the
* ELException
constructor.
*/
public abstract Object invoke(ELContext context, Object[] params);
}