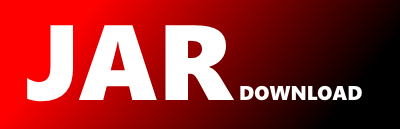
source.ca.odell.glazedlists.PopularityList Maven / Gradle / Ivy
/* Glazed Lists (c) 2003-2006 */
/* http://publicobject.com/glazedlists/ publicobject.com,*/
/* O'Dell Engineering Ltd.*/
package ca.odell.glazedlists;
import ca.odell.glazedlists.event.ListEvent;
import java.util.Comparator;
/**
* An {@link EventList} that shows the unique elements from its source
* {@link EventList} ordered by the frequency of their appearance.
*
* This {@link EventList} supports all write operations.
*
*
Warning: This class
* breaks the contract required by {@link java.util.List}. See
* {@link EventList} for an example.
*
*
Warning: This class is
* thread ready but not thread safe. See {@link EventList} for an example
* of thread safe code.
*
*
* EventList Overview
* Writable: yes
* Concurrency: thread ready, not thread safe
* Performance: reads: O(log N), writes O(log N)
* Memory: 196 bytes per element
* Unit Tests: N/A
* Issues:
* 104
*
*
*
* @author Jesse Wilson
*/
public final class PopularityList extends TransformedList {
/** the list of distinct elements */
private UniqueList uniqueList;
/**
* Creates a new {@link PopularityList} that provides frequency-ranking
* for the specified {@link EventList}. All elements of the source {@link EventList}
* must implement {@link Comparable}.
*/
public static > PopularityList create(EventList source) {
return new PopularityList(UniqueList.create(source));
}
/**
* Creates a new {@link PopularityList} that provides frequency-ranking
* for the specified {@link EventList}. All elements of the source {@link EventList}
* must implement {@link Comparable}.
* Usage of factory method {@link #create(EventList)} is preferable.
*/
public PopularityList(EventList source) {
this(new UniqueList(source));
}
/**
* Creates a new {@link PopularityList} that provides frequency-ranking
* for the specified {@link EventList}.
*
* @param uniqueComparator {@link Comparator} used to determine equality
*/
public PopularityList(EventList source, Comparator uniqueComparator) {
this(new UniqueList(source, uniqueComparator));
}
/**
* Private constructor is used as a Java-language hack to allow us to save
* a reference to the specified {@link UniqueList}.
*/
private PopularityList(UniqueList uniqueList) {
super(new SortedList(uniqueList, new PopularityComparator(uniqueList)));
this.uniqueList = uniqueList;
// listen for changes to the source list
source.addListEventListener(this);
}
/** {@inheritDoc} */
protected boolean isWritable() {
return true;
}
/** {@inheritDoc} */
public void listChanged(ListEvent listChanges) {
updates.forwardEvent(listChanges);
}
/** {@inheritDoc} */
public void dispose() {
SortedList sortedSource = (SortedList)source;
super.dispose();
sortedSource.dispose();
uniqueList.dispose();
}
/**
* Compares objects by their popularity.
*/
private static class PopularityComparator implements Comparator {
private UniqueList target;
public PopularityComparator(UniqueList target) {
this.target = target;
}
public int compare(E a, E b) {
int aCount = target.getCount(a);
int bCount = target.getCount(b);
return bCount - aCount;
}
}
}