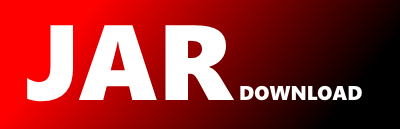
test.ca.odell.glazedlists.CompositeListTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glazedlists_java15 Show documentation
Show all versions of glazedlists_java15 Show documentation
Event-driven lists for dynamically filtered and sorted tables
/* Glazed Lists (c) 2003-2006 */
/* http://publicobject.com/glazedlists/ publicobject.com,*/
/* O'Dell Engineering Ltd.*/
package ca.odell.glazedlists;
import ca.odell.glazedlists.event.ListEventAssembler;
import ca.odell.glazedlists.event.ListEventPublisher;
import ca.odell.glazedlists.impl.testing.GlazedListsTests;
import ca.odell.glazedlists.impl.testing.ListConsistencyListener;
import ca.odell.glazedlists.util.concurrent.LockFactory;
import ca.odell.glazedlists.util.concurrent.ReadWriteLock;
import junit.framework.TestCase;
import java.util.ArrayList;
import java.util.List;
/**
* A CompositeListTest tests the functionality of the CompositeList.
*
* @author Jesse Wilson
*/
public class CompositeListTest extends TestCase {
/**
* Verifies that a single source works.
*/
public void testSingleSource() {
CompositeList fastFood = new CompositeList();
ListConsistencyListener.install(fastFood);
EventList wendys = fastFood.createMemberList();
wendys.add("Classic Single");
wendys.add("Chili");
wendys.add("Frosty");
wendys.add("Junior Bacon Cheeseburger");
fastFood.addMemberList(wendys);
assertEquals(wendys, fastFood);
wendys.add("Sour Cream 'n' Onion Baked Potato");
wendys.add("Taco Supremo Salad");
assertEquals(wendys, fastFood);
wendys.remove(1);
fastFood.set(0, "Big Bacon Classic");
fastFood.remove(1);
assertEquals(wendys, fastFood);
}
/**
* Verifies that multiple sources work.
*/
public void testMultipleSources() {
CompositeList fastFood = new CompositeList();
ListConsistencyListener.install(fastFood);
List fastFoodVerify = new ArrayList();
EventList wendys = fastFood.createMemberList();
wendys.add("Classic Single");
wendys.add("Chili");
wendys.add("Frosty");
wendys.add("Junior Bacon Cheeseburger");
EventList mcDonalds = fastFood.createMemberList();
mcDonalds.add("McDLT");
mcDonalds.add("McPizza");
mcDonalds.add("McSalad Shaker");
mcDonalds.add("Royal with Cheese");
EventList tacoBell = fastFood.createMemberList();
tacoBell.add("Fries Supreme");
tacoBell.add("Bean Burrito");
fastFood.addMemberList(wendys);
fastFood.addMemberList(mcDonalds);
fastFood.addMemberList(tacoBell);
fastFoodVerify.clear();
fastFoodVerify.addAll(wendys);
fastFoodVerify.addAll(mcDonalds);
fastFoodVerify.addAll(tacoBell);
assertEquals(fastFoodVerify, fastFood);
wendys.add("Sour Cream 'n' Onion Baked Potato");
wendys.add("Taco Supremo Salad");
fastFoodVerify.clear();
fastFoodVerify.addAll(wendys);
fastFoodVerify.addAll(mcDonalds);
fastFoodVerify.addAll(tacoBell);
assertEquals(fastFoodVerify, fastFood);
wendys.remove(1);
fastFood.set(0, "Big Bacon Classic");
fastFood.remove(1);
fastFoodVerify.clear();
fastFoodVerify.addAll(wendys);
fastFoodVerify.addAll(mcDonalds);
fastFoodVerify.addAll(tacoBell);
assertEquals(fastFoodVerify, fastFood);
mcDonalds.add("Big Mac");
fastFoodVerify.clear();
fastFoodVerify.addAll(wendys);
fastFoodVerify.addAll(mcDonalds);
fastFoodVerify.addAll(tacoBell);
assertEquals(fastFoodVerify, fastFood);
fastFood.removeMemberList(mcDonalds);
fastFoodVerify.clear();
fastFoodVerify.addAll(wendys);
fastFoodVerify.addAll(tacoBell);
assertEquals(fastFoodVerify, fastFood);
}
/**
* Verifies that remove member list does so by reference.
*/
public void testRemoveByReference() {
CompositeList fastFood = new CompositeList();
ListConsistencyListener.install(fastFood);
EventList wendys = fastFood.createMemberList();
EventList mcDonalds = fastFood.createMemberList();
EventList tacoBell = fastFood.createMemberList();
fastFood.addMemberList(wendys);
fastFood.addMemberList(mcDonalds);
fastFood.addMemberList(tacoBell);
fastFood.removeMemberList(tacoBell);
fastFood.removeMemberList(wendys);
assertEquals(mcDonalds, fastFood);
mcDonalds.add("Arch Deluxe");
assertEquals(mcDonalds, fastFood);
}
/**
* Verifies that multiple copies of the same list can be added.
*/
public void testMultipleCopies() {
List fastFoodVerify = new ArrayList();
CompositeList fastFood = new CompositeList();
EventList wendys = fastFood.createMemberList();
wendys.add("Spicy Chicken Sandwich");
EventList mcDonalds = fastFood.createMemberList();
mcDonalds.add("Arch Deluxe");
mcDonalds.add("McLean Deluxe");
fastFood.addMemberList(wendys);
fastFood.addMemberList(mcDonalds);
fastFoodVerify.clear();
fastFoodVerify.addAll(wendys);
fastFoodVerify.addAll(mcDonalds);
assertEquals(fastFoodVerify, fastFood);
fastFood.addMemberList(wendys);
fastFood.addMemberList(mcDonalds);
fastFoodVerify.clear();
fastFoodVerify.addAll(wendys);
fastFoodVerify.addAll(mcDonalds);
fastFoodVerify.addAll(wendys);
fastFoodVerify.addAll(mcDonalds);
assertEquals(fastFoodVerify, fastFood);
fastFood.removeMemberList(wendys);
fastFood.removeMemberList(wendys);
fastFoodVerify.clear();
fastFoodVerify.addAll(mcDonalds);
fastFoodVerify.addAll(mcDonalds);
assertEquals(fastFoodVerify, fastFood);
}
/**
* Test that {@link CompositeList} is well behaved when only a single element
* is removed.
*/
public void testSingleElements() {
CompositeList aToB = new CompositeList();
ListConsistencyListener.install(aToB);
EventList alpha = aToB.createMemberList();
alpha.add("A");
EventList beta = aToB.createMemberList();
beta.add("B");
aToB.addMemberList(alpha);
aToB.removeMemberList(alpha);
aToB.addMemberList(beta);
}
/**
* Test that when {@link CompositeList} is constructed with a publisher and read/write
* lock, it uses them and produces member lists which also use them.
*/
public void testPublisherAndLockConstructor() {
final ReadWriteLock sharedLock = LockFactory.DEFAULT.createReadWriteLock();
final ListEventPublisher sharedPublisher = ListEventAssembler.createListEventPublisher();
final EventList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy