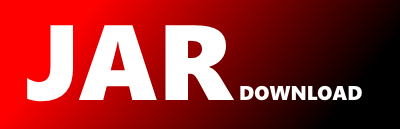
test.ca.odell.glazedlists.swing.EventSelectionModelSwingTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glazedlists_java15 Show documentation
Show all versions of glazedlists_java15 Show documentation
Event-driven lists for dynamically filtered and sorted tables
package ca.odell.glazedlists.swing;
import ca.odell.glazedlists.*;
import ca.odell.glazedlists.impl.testing.GlazedListsTests;
import ca.odell.glazedlists.matchers.Matchers;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
import java.util.Arrays;
import java.util.Collections;
/**
* This test verifies that the EventSelectionModel works.
*
* @author Jesse Wilson
*/
public class EventSelectionModelSwingTest extends SwingTestCase {
/**
* Tests that selection survives a sorting.
*/
public void guiTestSort() {
BasicEventList list = new BasicEventList();
SortedList sorted = new SortedList(list, null);
EventSelectionModel eventSelectionModel = new EventSelectionModel(sorted);
// populate the list
list.add("E");
list.add("C");
list.add("F");
list.add("B");
list.add("A");
list.add("D");
assertEquals(Arrays.asList(new String[] { }), eventSelectionModel.getSelected());
// select the vowels
eventSelectionModel.addSelectionInterval(0, 0);
eventSelectionModel.addSelectionInterval(4, 4);
assertEquals(Arrays.asList(new String[] { "E", "A" }), eventSelectionModel.getSelected());
// flip the list
sorted.setComparator(GlazedLists.comparableComparator());
assertEquals(Arrays.asList(new String[] { "A", "E" }), eventSelectionModel.getSelected());
// flip the list again
sorted.setComparator(GlazedLists.reverseComparator());
assertEquals(Arrays.asList(new String[] { "E", "A" }), eventSelectionModel.getSelected());
}
/**
* Verifies that the selected index is cleared when the selection is cleared.
*/
public void guiTestClear() {
BasicEventList list = new BasicEventList();
EventSelectionModel eventSelectionModel = new EventSelectionModel(list);
// populate the list
list.add("A");
list.add("B");
list.add("C");
list.add("D");
list.add("E");
list.add("F");
// make a selection
eventSelectionModel.addSelectionInterval(1, 4);
// test the selection
assertEquals(list.subList(1, 5), eventSelectionModel.getSelected());
// clear the selection
eventSelectionModel.clearSelection();
// test the selection
assertEquals(Collections.EMPTY_LIST, eventSelectionModel.getSelected());
assertEquals(-1, eventSelectionModel.getMinSelectionIndex());
assertEquals(-1, eventSelectionModel.getMaxSelectionIndex());
assertEquals(true, eventSelectionModel.isSelectionEmpty());
}
/**
* Tests a problem where the {@link ca.odell.glazedlists.swing.EventSelectionModel} fails to fire events
*
* This test was contributed by: Sergey Bogatyrjov
*/
public void guiTestSelectionModel() {
EventList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy