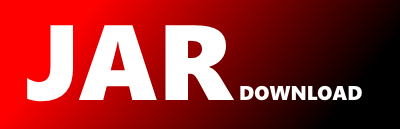
ca.odell.glazedlists.calculation.Calculations Maven / Gradle / Ivy
/* Glazed Lists (c) 2003-2007 */
/* http://publicobject.com/glazedlists/ publicobject.com,*/
/* O'Dell Engineering Ltd.*/
package ca.odell.glazedlists.calculation;
import ca.odell.glazedlists.EventList;
import ca.odell.glazedlists.matchers.Matcher;
public final class Calculations {
private Calculations() {}
//
// Counts
//
/** A Calculation that reports the number of elements
as an Integer. */
public static Calculation count(EventList elements) { return new Count(elements); }
/** A Calculation that reports the number of elements
that satisfy the given matcher
as an Integer. */
public static Calculation count(EventList elements, Matcher matcher) { return new ConditionalCount(elements, matcher); }
/** A Calculation that reports true when the number of elements
is 0
; false otherwise. */
public static Calculation zeroElements(EventList elements) { return new SizeInRange(elements, 0, 0); }
/** A Calculation that reports true when the number of elements
is 1
; false otherwise. */
public static Calculation oneElement(EventList elements) { return new SizeInRange(elements, 1, 1); }
/** A Calculation that reports true when the number of elements
is > 0
; false otherwise. */
public static Calculation oneOrMoreElements(EventList elements) { return new SizeInRange(elements, 1, Integer.MAX_VALUE); }
/** A Calculation that reports true when the number of elements
is > 1
; false otherwise. */
public static Calculation manyElements(EventList elements) { return new SizeInRange(elements, 2, Integer.MAX_VALUE); }
//
// Sum
//
/** A Calculation that sums the given numbers
as a Float. */
public static Calculation sumFloats(EventList extends Number> numbers) { return new Sum.SumFloat(numbers); }
/** A Calculation that sums the given numbers
as a Double. */
public static Calculation sumDoubles(EventList extends Number> numbers) { return new Sum.SumDouble(numbers); }
/** A Calculation that sums the given numbers
as an Integer. */
public static Calculation sumIntegers(EventList extends Number> numbers) { return new Sum.SumInteger(numbers); }
/** A Calculation that sums the given numbers
as a Long. */
public static Calculation sumLongs(EventList extends Number> numbers) { return new Sum.SumLong(numbers); }
//
// Division
//
/** A Calculation that divides the numerator
by the denominator
as Floats. */
public static Calculation divideFloats(Calculation extends Number> numerator, Calculation extends Number> denominator) { return new Division.DivisionFloat(numerator, denominator); }
/** A Calculation that divides the numerator
by the denominator
as Doubles. */
public static Calculation divideDoubles(Calculation extends Number> numerator, Calculation extends Number> denominator) { return new Division.DivisionDouble(numerator, denominator); }
//
// Subtraction
//
/** A Calculation that subtracts b
from a
as Floats. */
public static Calculation subtractFloats(Calculation extends Number> a, Calculation extends Number> b) { return new Subtraction.SubtractionFloat(a, b); }
/** A Calculation that subtracts b
from a
as Doubles. */
public static Calculation subtractDoubles(Calculation extends Number> a, Calculation extends Number> b) { return new Subtraction.SubtractionDouble(a, b); }
/** A Calculation that subtracts b
from a
as Integers. */
public static Calculation subtractIntegers(Calculation extends Number> a, Calculation extends Number> b) { return new Subtraction.SubtractionInteger(a, b); }
/** A Calculation that subtracts b
from a
as Longs. */
public static Calculation subtractLongs(Calculation extends Number> a, Calculation extends Number> b) { return new Subtraction.SubtractionLong(a, b); }
//
// Mean Average
//
/** A Calculation that reports the mean average of all the numbers
as a Float. */
public static Calculation meanFloats(EventList extends Number> numbers) { return divideFloats(sumFloats(numbers), count(numbers)); }
/** A Calculation that reports the mean average of all the numbers
as a Double. */
public static Calculation meanDoubles(EventList extends Number> numbers) { return divideDoubles(sumDoubles(numbers), count(numbers)); }
//
// Miscellaneous
//
/** A Calculation that value at the given index
in the given elements
. If elements
does not contain enough items, the given defaultValue
is returned. */
public static Calculation elementAt(EventList elements, int index, E defaultValue) { return new ElementAt(elements, index, defaultValue); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy