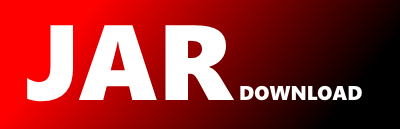
net.java.truecommons.io.OneTimeFoundry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truecommons-io Show documentation
Show all versions of truecommons-io Show documentation
Provides common I/O components.
/*
* Copyright (C) 2012 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package net.java.truecommons.io;
import java.io.Closeable;
import java.io.IOException;
import java.nio.channels.Channel;
import java.util.Objects;
import javax.annotation.CheckForNull;
/**
* A source or sink which provides a given stream or channel at most once.
*
* @param the type of the stream which gets returned by {@link #stream()}.
* @param the type of the channel which gets returned by {@link #channel()}.
* @author Christian Schlichtherle
*/
public abstract class OneTimeFoundry {
private @CheckForNull S stream;
private @CheckForNull C channel;
OneTimeFoundry(final S stream) {
this.stream = Objects.requireNonNull(stream);
}
OneTimeFoundry(final C channel) {
this.channel = Objects.requireNonNull(channel);
}
public S stream() throws IOException {
final S stream = this.stream;
if (null == stream)
throw new IllegalStateException();
this.stream = null;
return stream;
}
public C channel() throws IOException {
final C channel = this.channel;
if (null == channel)
throw new IllegalStateException();
this.channel = null;
return channel;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy