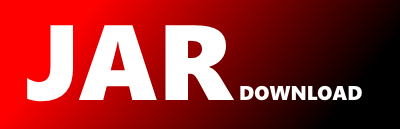
net.java.truelicense.maven.plugin.obfuscation.Processor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truelicense-maven-plugin Show documentation
Show all versions of truelicense-maven-plugin Show documentation
Provides a Maven plugin with goals for the obfuscation of constant
string values in Java class and test-class files (byte code).
/*
* Copyright (C) 2005-2013 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package net.java.truelicense.maven.plugin.obfuscation;
import de.schlichtherle.truezip.file.TFile;
import java.io.File;
import java.util.Formatter;
import java.util.HashSet;
import java.util.Set;
import net.java.truelicense.obfuscate.Obfuscate;
import org.objectweb.asm.ClassVisitor;
import org.slf4j.Logger;
/**
* Runs the obfuscation based on the configuration parameters provided to its
* constructor(s).
*
* @author Christian Schlichtherle
*/
public final class Processor implements Runnable {
/**
* The default value of the {@code maxBytes} property, which is {@value}.
*
* @see #maxBytes()
*/
public static final int DEFAULT_MAX_BYTES = 64 * 1024;
/**
* The default value of the {@code obfuscateAll} property, which is {@value}.
*
* @see #obfuscateAll()
*/
public static final boolean DEFAULT_OBFUSCATE_ALL = false;
/**
* The default value of the {@code methodNameFormat} property, which is {@value}.
*
* @see #methodNameFormat()
*/
public static final String DEFAULT_METHOD_NAME_FORMAT = "_%s#%d";
/**
* The default value of the {@code internStrings} property, which is {@value}.
*
* @see #internStrings()
*/
public static final boolean DEFAULT_INTERN_STRINGS = true;
private final Set constantStrings = new HashSet();
private final Logger logger;
private final TFile directory;
private final int maxBytes;
private final boolean obfuscateAll;
private final String methodNameFormat;
private final boolean internStrings;
/**
* Constructs a processor with the given parameters.
* The constructed processor uses
* {@link #DEFAULT_MAX_BYTES}, {@link #DEFAULT_OBFUSCATE_ALL},
* {@link #DEFAULT_METHOD_NAME_FORMAT} and {@link #DEFAULT_INTERN_STRINGS}.
*
* @see #logger()
* @see #directory()
*/
public Processor(Logger logger, File directory) {
this(logger, directory,
DEFAULT_MAX_BYTES,
DEFAULT_OBFUSCATE_ALL,
DEFAULT_METHOD_NAME_FORMAT,
DEFAULT_INTERN_STRINGS);
}
/**
* Constructs a processor with the given parameters.
*
* @see #logger()
* @see #directory()
* @see #maxBytes()
* @see #obfuscateAll()
* @see #methodNameFormat()
* @see #internStrings()
*/
public Processor(
final Logger logger,
final File directory,
final int maxBytes,
final boolean obfuscateAll,
final String methodNameFormat,
final boolean internStrings) {
this.obfuscateAll = obfuscateAll;
if (null == (this.logger = logger)) throw new NullPointerException();
this.directory = directory instanceof TFile
? (TFile) directory
: new TFile(directory);
if (0 >= (this.maxBytes = maxBytes))
throw new IllegalArgumentException();
if (null == (this.methodNameFormat = methodNameFormat))
throw new NullPointerException();
this.internStrings = internStrings;
}
/**
* Returns the logger to use.
* Any errors should be logged at the error level to enable subsequent
* checking.
*/
public Logger logger() { return logger; }
/** Returns the directory to scan for class files to process. */
public File directory() { return directory; }
/** Returns the maximum allowed size of a class file in bytes. */
public int maxBytes() { return maxBytes; }
/**
* Returns {@code true} if all constant string values shall get obfuscated.
* Otherwise only constant string values of fields annotated with
* {@link Obfuscate} shall get obfuscated.
*
* @return Whether or not all constant string values shall get obfuscated.
*/
public boolean obfuscateAll() { return obfuscateAll; }
/**
* Returns the methodName for synthesized method names.
* This a methodName string for the class {@link Formatter}.
* It's first parameter is a string identifier for the obfuscation stage
* and its second parameter is an integer index for the synthesized method.
*/
public String methodNameFormat() { return methodNameFormat; }
/**
* Returns whether or not a call to java.lang.String.intern()
* shall get added when computing the original constant string values again.
* Use this to preserve the identity relation of constant string values if
* required.
*/
public boolean internStrings() { return internStrings; }
/**
* Returns the set of constant strings to obfuscate.
* The returned set is only used when {@link #obfuscateAll} is
* {@code false} and is modifiable so as to exchange the set between
* different processing paths.
*
* @return The set of constant strings to obfuscate.
*/
@SuppressWarnings("ReturnOfCollectionOrArrayField")
Set constantStrings() { return constantStrings; }
Logger logger(String subject) {
return new SubjectLogger(logger(), subject);
}
String methodName(String stage, int index) {
return new Formatter().format(methodNameFormat(), stage, index).toString();
}
/** Runs the obfuscation processor. */
@Override public void run() {
firstPass().run();
secondPass().run();
}
Runnable firstPass() { return new FirstPass(this); }
Runnable secondPass() { return new SecondPass(this); }
ClassVisitor collector() { return new Collector(this); }
ClassVisitor obfuscator(ClassVisitor cv) {
return new Obfuscator(this, cv);
}
ClassVisitor merger(ClassVisitor cv, String prefix) {
return new Merger(this, prefix, cv);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy