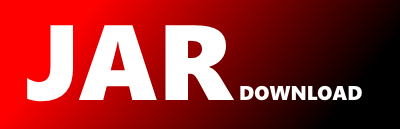
net.java.truelicense.swing.LicensePanel Maven / Gradle / Ivy
/*
* Copyright (C) 2005-2013 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package net.java.truelicense.swing;
import java.text.DateFormat;
import java.util.Date;
import javax.swing.JPanel;
import net.java.truelicense.core.License;
import net.java.truelicense.core.LicenseValidationException;
import net.java.truelicense.core.util.Objects;
import static net.java.truelicense.swing.Messages.*;
import net.java.truelicense.swing.nexes.WizardPanelDescriptor;
/**
* @author Christian Schlichtherle
*/
public class LicensePanel extends JPanel {
private static final long serialVersionUID = 1L;
private final ObservableLicenseConsumerManager ocm;
private License license;
public LicensePanel(final ObservableLicenseConsumerManager ocm) {
this.ocm = Objects.requireNonNull(ocm);
initComponents();
}
/** Updates the panel with the contents of the license and verifies it. */
private void verify() {
try {
license = ocm.view();
updatePanel();
ocm.verify();
} catch (final Exception failure) {
Dialogs.showMessageDialog(
this,
failure instanceof LicenseValidationException
? failure.getLocalizedMessage()
: message("LicensePanel.failure.message").toString(), // don't show details!
message("LicensePanel.failure.title").toString(),
Dialogs.ERROR_MESSAGE);
}
}
/**
* Updates the panel to display the installed license.
*/
protected void updatePanel() {
if (null == license) return;
subjectComponent.setText(toString(license.getSubject()));
holderComponent.setText(toString(license.getHolder()));
issuerComponent.setText(toString(license.getIssuer()));
issuedComponent.setText(format(license.getIssued()));
notBeforeComponent.setText(format(license.getNotBefore()));
notAfterComponent.setText(format(license.getNotAfter()));
consumerComponent.setText(toString(license.getConsumerType()) + " / " + license.getConsumerAmount());
infoComponent.setText(toString(license.getInfo()));
}
private String format(final Date date) {
return null != date
? DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG).format(date)
: "";
}
private String toString(final Object obj) {
return null != obj ? obj.toString() : "";
}
/**
* Adds the given license consumer manager listener.
* It is safe to call this method from a listener implementation.
* The implementation uses a list, so adding the same listener multiple
* times will result in multiple notifications for each event.
*
* @param cml the license consumer manager listener to add.
*/
public void addLicenseConsumerManagerListener(
LicenseConsumerManagerListener cml) {
ocm.addLicenseConsumerManagerListener(cml);
}
/**
* Removes the given license consumer manager listener.
* It is safe to call this method from a listener implementation.
* Removing an listener which is not registered has no effect.
*
* @param cml the license consumer manager listener to remove.
*/
public void removeLicenseConsumerManagerListener(
LicenseConsumerManagerListener cml) {
ocm.removeLicenseConsumerManagerListener(cml);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
subjectLabel = new javax.swing.JLabel();
subjectComponent = new javax.swing.JTextArea();
holderLabel = new javax.swing.JLabel();
holderScrollPane = new javax.swing.JScrollPane();
holderComponent = new javax.swing.JTextArea();
issuerLabel = new javax.swing.JLabel();
issuerScrollPane = new javax.swing.JScrollPane();
issuerComponent = new javax.swing.JTextArea();
issuedLabel = new javax.swing.JLabel();
issuedComponent = new javax.swing.JTextArea();
notBeforeLabel = new javax.swing.JLabel();
notBeforeComponent = new javax.swing.JTextArea();
notAfterLabel = new javax.swing.JLabel();
notAfterComponent = new javax.swing.JTextArea();
consumerLabel = new javax.swing.JLabel();
consumerComponent = new javax.swing.JTextArea();
infoLabel = new javax.swing.JLabel();
infoScrollPane = new javax.swing.JScrollPane();
infoComponent = new javax.swing.JTextArea();
setBorder(javax.swing.BorderFactory.createCompoundBorder(javax.swing.BorderFactory.createTitledBorder(message("LicensePanel.title").toString()), javax.swing.BorderFactory.createEmptyBorder(10, 10, 10, 10))); // NOI18N
setLayout(new java.awt.GridBagLayout());
subjectLabel.setLabelFor(subjectComponent);
subjectLabel.setText(message("LicensePanel.subject.label").toString()); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 0;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
add(subjectLabel, gridBagConstraints);
subjectComponent.setEditable(false);
subjectComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
subjectComponent.setName("subject"); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 0;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
add(subjectComponent, gridBagConstraints);
holderLabel.setLabelFor(holderComponent);
holderLabel.setText(message("LicensePanel.holder.label").toString()); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 1;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
add(holderLabel, gridBagConstraints);
holderScrollPane.setBorder(javax.swing.BorderFactory.createEtchedBorder());
holderScrollPane.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
holderScrollPane.setPreferredSize(new java.awt.Dimension(300, 65));
holderComponent.setEditable(false);
holderComponent.setLineWrap(true);
holderComponent.setWrapStyleWord(true);
holderComponent.setBorder(null);
holderComponent.setName("holder"); // NOI18N
holderScrollPane.setViewportView(holderComponent);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 1;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
add(holderScrollPane, gridBagConstraints);
issuerLabel.setLabelFor(issuerComponent);
issuerLabel.setText(message("LicensePanel.issuer.label").toString()); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 2;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
add(issuerLabel, gridBagConstraints);
issuerScrollPane.setBorder(javax.swing.BorderFactory.createEtchedBorder());
issuerScrollPane.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
issuerScrollPane.setPreferredSize(new java.awt.Dimension(300, 65));
issuerComponent.setEditable(false);
issuerComponent.setLineWrap(true);
issuerComponent.setWrapStyleWord(true);
issuerComponent.setBorder(null);
issuerComponent.setName("issuer"); // NOI18N
issuerScrollPane.setViewportView(issuerComponent);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
add(issuerScrollPane, gridBagConstraints);
issuedLabel.setLabelFor(issuedComponent);
issuedLabel.setText(message("LicensePanel.issued.label").toString()); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 3;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
add(issuedLabel, gridBagConstraints);
issuedComponent.setEditable(false);
issuedComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
issuedComponent.setName("issued"); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 3;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
add(issuedComponent, gridBagConstraints);
notBeforeLabel.setLabelFor(notBeforeComponent);
notBeforeLabel.setText(message("LicensePanel.notBefore.label").toString()); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 4;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
add(notBeforeLabel, gridBagConstraints);
notBeforeComponent.setEditable(false);
notBeforeComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
notBeforeComponent.setName("notBefore"); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 4;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
add(notBeforeComponent, gridBagConstraints);
notAfterLabel.setLabelFor(notAfterComponent);
notAfterLabel.setText(message("LicensePanel.notAfter.label").toString()); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 5;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
add(notAfterLabel, gridBagConstraints);
notAfterComponent.setEditable(false);
notAfterComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
notAfterComponent.setName("notAfter"); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 5;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
add(notAfterComponent, gridBagConstraints);
consumerLabel.setLabelFor(consumerComponent);
consumerLabel.setText(message("LicensePanel.consumer.label").toString()); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 6;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
add(consumerLabel, gridBagConstraints);
consumerComponent.setEditable(false);
consumerComponent.setBorder(javax.swing.BorderFactory.createEtchedBorder());
consumerComponent.setName("consumer"); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 6;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
add(consumerComponent, gridBagConstraints);
infoLabel.setLabelFor(infoComponent);
infoLabel.setText(message("LicensePanel.info.label").toString()); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 7;
gridBagConstraints.ipadx = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
add(infoLabel, gridBagConstraints);
infoScrollPane.setBorder(javax.swing.BorderFactory.createEtchedBorder());
infoScrollPane.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
infoScrollPane.setPreferredSize(new java.awt.Dimension(300, 65));
infoComponent.setEditable(false);
infoComponent.setLineWrap(true);
infoComponent.setWrapStyleWord(true);
infoComponent.setBorder(null);
infoComponent.setName("info"); // NOI18N
infoScrollPane.setViewportView(infoComponent);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 7;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.anchor = java.awt.GridBagConstraints.BASELINE_LEADING;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
add(infoScrollPane, gridBagConstraints);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JTextArea consumerComponent;
private javax.swing.JLabel consumerLabel;
private javax.swing.JTextArea holderComponent;
private javax.swing.JLabel holderLabel;
private javax.swing.JScrollPane holderScrollPane;
private javax.swing.JTextArea infoComponent;
private javax.swing.JLabel infoLabel;
private javax.swing.JScrollPane infoScrollPane;
private javax.swing.JTextArea issuedComponent;
private javax.swing.JLabel issuedLabel;
private javax.swing.JTextArea issuerComponent;
private javax.swing.JLabel issuerLabel;
private javax.swing.JScrollPane issuerScrollPane;
private javax.swing.JTextArea notAfterComponent;
private javax.swing.JLabel notAfterLabel;
private javax.swing.JTextArea notBeforeComponent;
private javax.swing.JLabel notBeforeLabel;
private javax.swing.JTextArea subjectComponent;
private javax.swing.JLabel subjectLabel;
// End of variables declaration//GEN-END:variables
public static class Descriptor extends WizardPanelDescriptor {
public static final String IDENTIFIER = "LICENSE_PANEL"; // NOI18N
public Descriptor(ObservableLicenseConsumerManager ocm) {
super(IDENTIFIER, new LicensePanel(ocm));
}
@Override public LicensePanel getPanel() {
return (LicensePanel) super.getPanel();
}
@Override public String getBackPanelIdentifier() {
return WelcomePanel.Descriptor.IDENTIFIER;
}
@Override public String getNextPanelIdentifier() {
return FINISH;
}
@Override public void aboutToDisplayPanel() {
getPanel().verify();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy