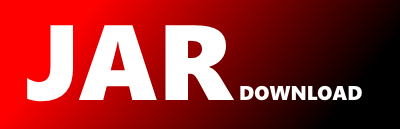
net.java.truelicense.swing.WelcomePanel Maven / Gradle / Ivy
/*
* Copyright (C) 2005-2013 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package net.java.truelicense.swing;
import java.security.GeneralSecurityException;
import javax.swing.*;
import net.java.truelicense.core.util.Objects;
import static net.java.truelicense.swing.Messages.*;
import net.java.truelicense.swing.nexes.WizardModel;
import net.java.truelicense.swing.nexes.WizardPanelDescriptor;
/**
* @author Christian Schlichtherle
*/
public class WelcomePanel extends javax.swing.JPanel {
private static final long serialVersionUID = 1L;
private final ObservableLicenseConsumerManager manager;
public WelcomePanel(final ObservableLicenseConsumerManager manager) {
this.manager = Objects.requireNonNull(manager);
initComponents();
uninstallButton.setVisible(false);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
prompt = new javax.swing.JTextArea();
installButton = new net.java.truelicense.swing.util.EnhancedRadioButton();
verifyButton = new net.java.truelicense.swing.util.EnhancedRadioButton();
uninstallButton = new net.java.truelicense.swing.util.EnhancedRadioButton();
setLayout(new java.awt.GridBagLayout());
prompt.setEditable(false);
prompt.setLineWrap(true);
prompt.setText(message("WelcomePanel.prompt.text", new Object[] {manager.subject()}).toString()); // NOI18N
prompt.setWrapStyleWord(true);
prompt.setBorder(null);
prompt.setOpaque(false);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 0;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.ipady = 5;
gridBagConstraints.anchor = java.awt.GridBagConstraints.SOUTH;
gridBagConstraints.weightx = 1.0;
add(prompt, gridBagConstraints);
buttonGroup.add(installButton);
installButton.setSelected(true);
installButton.setText(message("WelcomePanel.installButton.text").toString()); // NOI18N
installButton.setActionCommand(InstallPanel.Descriptor.IDENTIFIER);
installButton.setName("install"); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 1;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
add(installButton, gridBagConstraints);
buttonGroup.add(verifyButton);
verifyButton.setText(message("WelcomePanel.verifyButton.text").toString()); // NOI18N
verifyButton.setActionCommand(LicensePanel.Descriptor.IDENTIFIER);
verifyButton.setName("verify"); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 2;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
add(verifyButton, gridBagConstraints);
buttonGroup.add(uninstallButton);
uninstallButton.setText(message("WelcomePanel.uninstallButton.text").toString()); // NOI18N
uninstallButton.setActionCommand(UninstallPanel.Descriptor.IDENTIFIER);
uninstallButton.setName("uninstall"); // NOI18N
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 3;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
add(uninstallButton, gridBagConstraints);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private final javax.swing.ButtonGroup buttonGroup = new javax.swing.ButtonGroup();
private net.java.truelicense.swing.util.EnhancedRadioButton installButton;
private javax.swing.JTextArea prompt;
private net.java.truelicense.swing.util.EnhancedRadioButton uninstallButton;
private net.java.truelicense.swing.util.EnhancedRadioButton verifyButton;
// End of variables declaration//GEN-END:variables
String getNextPanelIdentifier() {
return buttonGroup.getSelection().getActionCommand();
}
/**
* Returns {@code true} if and only if the uninstall button is visible.
* By default, this button is not visible.
*/
public boolean isUninstallButtonVisible() {
return uninstallButton.isVisible();
}
/** Sets the visibility of the uninstall button. */
public void setUninstallButtonVisible(final boolean visible) {
uninstallButton.setVisible(visible);
validate();
}
/**
* Starts a background task to verify the license certificate. This is
* provided for faster startup of the welcome panel.
*/
private void startVerifyingLicense(final WizardModel model) {
model.setNextButtonEnabled(Boolean.FALSE);
verifyButton.setEnabled(false);
uninstallButton.setEnabled(false);
new SwingWorker() {
@Override protected Boolean doInBackground() throws Exception {
try {
manager.verify();
return true;
} catch (final GeneralSecurityException ex) {
return false;
}
}
@Override protected void done() {
boolean verified;
try { verified = get(); }
catch (final Exception ex) { verified = false; }
verifyButton.setEnabled(verified);
uninstallButton.setEnabled(verified);
buttonGroup.setSelected((verified ? verifyButton : installButton).getModel(), true);
model.setNextButtonEnabled(Boolean.TRUE);
}
}.execute();
}
public static class Descriptor extends WizardPanelDescriptor {
public static final String IDENTIFIER = "WELCOME_PANEL"; // NOI18N
public Descriptor(ObservableLicenseConsumerManager ocm) {
super(IDENTIFIER, new WelcomePanel(ocm));
}
@Override public WelcomePanel getPanel() {
return (WelcomePanel) super.getPanel();
}
@Override public void aboutToDisplayPanel() {
getPanel().startVerifyingLicense(getWizardModel());
}
@Override public String getNextPanelIdentifier() {
return getPanel().getNextPanelIdentifier();
}
/**
* Returns {@code true} if and only if the uninstall button is visible.
* By default, this button is not visible.
*/
public boolean isUninstallButtonVisible() {
return getPanel().isUninstallButtonVisible();
}
/** Sets the visibility of the uninstall button. */
public void setUninstallButtonVisible(boolean visible) {
getPanel().setUninstallButtonVisible(visible);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy