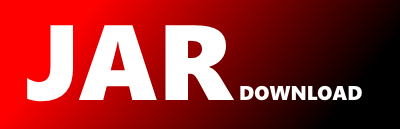
net.java.truelicense.ui.wizard.BasicWizardModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truelicense-ui Show documentation
Show all versions of truelicense-ui Show documentation
The TrueLicense UI module provides a user interface technology
independent model for wizard dialogs in general and license management
wizard dialogs in particular.
/*
* Copyright (C) 2005-2015 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package net.java.truelicense.ui.wizard;
import java.util.*;
import net.java.truelicense.core.util.Objects;
/**
* A basic implementation of a wizard model.
*
* @param the type of the wizard's states.
* @param the type of the wizard's views.
* @since TrueLicense 2.3
* @author Christian Schlichtherle
*/
public class BasicWizardModel implements WizardModel {
/**
* Creates a new wizard model for the given enum class.
* The model will have no views and it's current state will be the first
* enum object.
*
* @param clazz the clazz of the enum type which represents the wizard's
* state.
* @param the enum type of the wizard's states.
* @param the type of the wizard's views.
*/
public static , V> WizardModel create(
final Class clazz) {
return new BasicWizardModel(new EnumMap(clazz),
clazz.getEnumConstants()[0]);
}
private final Map views;
private S state;
/**
* Constructs a new wizard model with the given map of views and state.
*
* @param views the map of views, which will be shared with the caller.
* @param state the initial state of the constructed model;
*/
protected BasicWizardModel(final Map views, final S state) {
this.views = Objects.requireNonNull(views);
this.state = Objects.requireNonNull(state);
}
@Override public S currentState() { return state; }
@Override public void currentState(final S state) {
this.state = Objects.requireNonNull(state);
}
@Override public V view(S state) { return views.get(state); }
@Override public void view(S state, V view) {
views.put(state, Objects.requireNonNull(view));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy