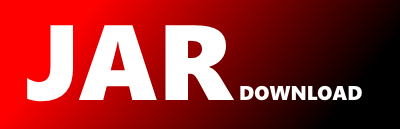
net.java.truelicense.ui.wizard.BasicWizardController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truelicense-ui Show documentation
Show all versions of truelicense-ui Show documentation
The TrueLicense UI module provides a user interface technology
independent model for wizard dialogs in general and license management
wizard dialogs in particular.
/*
* Copyright (C) 2005-2015 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package net.java.truelicense.ui.wizard;
import javax.annotation.concurrent.Immutable;
/**
* A basic implementation of a wizard controller.
*
* @param the type of the wizard's states.
* @param the type of the wizard's views.
* @since TrueLicense 2.3
* @author Christian Schlichtherle
*/
@Immutable
public abstract class BasicWizardController>
implements WizardController {
/**
* Creates a new wizard controller with the given model.
*
* @param model the wizard model.
* @param the type of the wizard's states.
* @param the type of the wizard's views.
*/
public static > WizardController create(
final WizardModel model) {
return new BasicWizardController() {
@Override protected WizardModel model() { return model; }
};
}
/** Returns the wizard model. */
protected abstract WizardModel model();
@Override public final boolean switchBackEnabled() {
return !backState().equals(currentState());
}
@Override public final void switchBack() { switchTo(backState()); }
@Override public final boolean switchNextEnabled() {
return !nextState().equals(currentState());
}
@Override public final void switchNext() { switchTo(nextState()); }
/** Switches from the current state to the given state. */
private void switchTo(final S newState) {
if (newState.equals(currentState())) return;
fireBeforeStateSwitch();
currentState(newState);
fireAfterStateSwitch();
}
/**
* Notifies the current view of the event by calling its
* {@link WizardView#onBeforeStateSwitch} method and then calls this
* controller's {@link #onBeforeStateSwitch} hook.
* This method gets called before this controller switches the state of
* the model.
*/
protected final void fireBeforeStateSwitch() {
currentView().onBeforeStateSwitch();
onBeforeStateSwitch();
}
/**
* Override this template method in order to add some behaviour before this
* controller switches the state of the model, but after the current view
* has been notified via {@link WizardView#onBeforeStateSwitch}.
*
* @see #currentView
*/
protected void onBeforeStateSwitch() { beforeStateSwitch(); }
/** @deprecated Override {@link #onBeforeStateSwitch} instead. */
@Deprecated
protected void beforeStateSwitch() { }
/**
* Calls this controller's {@link #onAfterStateSwitch} hook and then
* notifies the current view of the event by calling its
* {@link WizardView#onAfterStateSwitch} method.
* This method gets called after this controller has switched the state of
* the model.
*/
protected final void fireAfterStateSwitch() {
onAfterStateSwitch();
currentView().onAfterStateSwitch();
}
/**
* Override this template method in order to add some behaviour after this
* controller has switched the state of the model, but before the current
* view gets notified via {@link WizardView#onAfterStateSwitch}.
*
* @see #currentView
*/
protected void onAfterStateSwitch() { afterStateSwitch(); }
/** @deprecated Override {@link #onAfterStateSwitch} instead. */
@Deprecated
protected void afterStateSwitch() { }
/** Returns the view of the current state. */
protected final V currentView() { return view(currentState()); }
/** Returns the view for the given state. */
protected final V view(S state) { return model().view(state); }
/** Sets the view for the given state. */
protected void view(S state, V view) { model().view(state, view); }
/** Returns the current state. */
protected final S currentState() { return model().currentState(); }
/** Sets the current state. */
private void currentState(S state) { model().currentState(state); }
/**
* Returns the state to switch to when the back-button gets pressed,
* or the current state if switching is not possible.
*/
protected final S backState() { return currentView().backState(); }
/**
* Returns the state to switch to when the next-button gets pressed,
* or the current state if switching is not possible.
*/
protected final S nextState() { return currentView().nextState(); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy