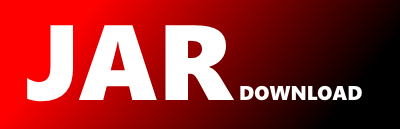
net.java.truevfs.comp.zip.DefaultExtraField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truevfs-comp-zip Show documentation
Show all versions of truevfs-comp-zip Show documentation
Provides basic functionality for reading and writing ZIP files.
/*
* Copyright (C) 2005-2015 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package net.java.truevfs.comp.zip;
import static java.lang.System.arraycopy;
import javax.annotation.CheckForNull;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Default implementation for an Extra Field in a Local or Central Header of a
* ZIP file.
*
* @author Christian Schlichtherle
*/
@NotThreadSafe
final class DefaultExtraField extends ExtraField {
private final short headerId;
private @CheckForNull byte[] data;
/**
* Constructs a new extra field.
*
* @param headerId an unsigned short integer (two bytes) indicating the
* type of the extra field.
*/
DefaultExtraField(final int headerId) {
assert UShort.check(headerId);
this.headerId = (short) headerId;
}
@Override
int getHeaderId() {
return headerId & UShort.MAX_VALUE;
}
@Override
int getDataSize() {
final byte[] data = this.data;
return null != data ? data.length : 0;
}
@Override
void readFrom(final byte[] buf, final int off, final int len) {
assert UShort.check(len);
arraycopy(buf, off, this.data = new byte[len], 0, len);
}
@Override
void writeTo(byte[] buf, int off) {
final byte[] src = this.data;
if (null != src) arraycopy(src, 0, buf, off, src.length);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy