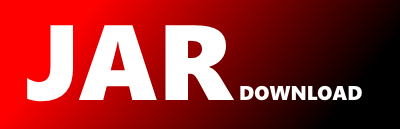
net.java.truevfs.ext.pacemaker.AspectController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truevfs-ext-pacemaker Show documentation
Show all versions of truevfs-ext-pacemaker Show documentation
Constrains the number of concurrently mounted archive file systems in
order to save some heap space.
This module provides a JMX interface for monitoring and management.
Add the JAR artifact of this module to the run time class path to
make its services available for service location in the client API
modules.
The newest version!
/*
* Copyright © 2005 - 2021 Schlichtherle IT Services.
* All rights reserved. Use is subject to license terms.
*/
package net.java.truevfs.ext.pacemaker;
import net.java.truecommons.cio.*;
import net.java.truecommons.shed.BitField;
import net.java.truevfs.kernel.spec.*;
import javax.annotation.CheckForNull;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.channels.SeekableByteChannel;
import java.util.Map;
import java.util.concurrent.Callable;
/**
* Calls a template method to apply an aspect to all file system operations except {@link #sync(BitField)}.
*
* @see #apply
* @author Christian Schlichtherle
*/
abstract class AspectController extends FsDecoratingController {
AspectController(FsController controller) {
super(controller);
}
/**
* Applies the aspect to the given file system operation.
*
* @param op the file system operation to apply an aspect to.
* @return The return value of the file system operation.
*/
abstract T apply(Op op) throws IOException;
interface Op extends Callable {
T call() throws IOException;
}
@CheckForNull
@Override
public final FsNode node(BitField options, FsNodeName name) throws IOException {
return apply(() -> controller.node(options, name));
}
@Override
public final void checkAccess(BitField options, FsNodeName name, BitField types) throws IOException {
apply(() -> {
controller.checkAccess(options, name, types);
return null;
});
}
@Override
public final void setReadOnly(BitField options, FsNodeName name) throws IOException {
apply(() -> {
controller.setReadOnly(options, name);
return null;
});
}
@Override
public final boolean setTime(BitField options, FsNodeName name, Map times) throws IOException {
return apply(() -> controller.setTime(options, name, times));
}
@Override
public final boolean setTime(BitField options, FsNodeName name, BitField types, long value) throws IOException {
return apply(() -> controller.setTime(options, name, types, value));
}
@Override
public final InputSocket extends Entry> input(BitField options, FsNodeName name) {
return new Input(controller.input(options, name));
}
private final class Input extends AbstractInputSocket {
private final InputSocket extends Entry> socket;
Input(final InputSocket extends Entry> socket) {
this.socket = socket;
}
@Override
public Entry target() throws IOException {
return apply(socket::target);
}
@Override
public InputStream stream(OutputSocket extends Entry> peer) throws IOException {
return apply(() -> socket.stream(peer));
}
@Override
public SeekableByteChannel channel(OutputSocket extends Entry> peer) throws IOException {
return apply(() -> socket.channel(peer));
}
}
@Override
public final OutputSocket extends Entry> output(BitField options, FsNodeName name, @CheckForNull Entry template) {
return new Output(controller.output(options, name, template));
}
private final class Output extends AbstractOutputSocket {
private final OutputSocket extends Entry> socket;
Output(final OutputSocket extends Entry> socket) {
this.socket = socket;
}
@Override
public Entry target() throws IOException {
return apply(socket::target);
}
@Override
public OutputStream stream(InputSocket extends Entry> peer) throws IOException {
return apply(() -> socket.stream(peer));
}
@Override
public SeekableByteChannel channel(InputSocket extends Entry> peer) throws IOException {
return apply(() -> socket.channel(peer));
}
}
@Override
public final void make(BitField options, FsNodeName name, Entry.Type type, @CheckForNull Entry template) throws IOException {
apply(() -> {
controller.make(options, name, type, template);
return null;
});
}
@Override
public final void unlink(BitField options, FsNodeName name) throws IOException {
apply(() -> {
controller.unlink(options, name);
return null;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy