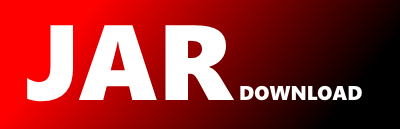
net.jodah.sarge.internal.SupervisionRegistry Maven / Gradle / Ivy
package net.jodah.sarge.internal;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import net.jodah.sarge.Plan;
import net.jodah.sarge.Supervisor;
/**
* Statically stores and retrieves SupervisionContext instances.
*
* @author Jonathan Halterman
*/
public class SupervisionRegistry {
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy