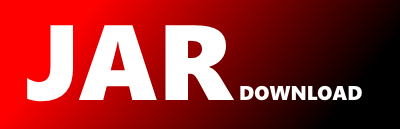
net.jqwik.engine.properties.arbitraries.exhaustive.UniqueExhaustiveGenerator Maven / Gradle / Ivy
package net.jqwik.engine.properties.arbitraries.exhaustive;
import java.util.*;
import java.util.concurrent.*;
import net.jqwik.api.*;
public class UniqueExhaustiveGenerator implements ExhaustiveGenerator {
private static final long MAX_MISSES = 10000;
private final ExhaustiveGenerator base;
public UniqueExhaustiveGenerator(ExhaustiveGenerator base) {
this.base = base;
}
@Override
public boolean isUnique() {
return true;
}
@Override
public long maxCount() {
return base.maxCount();
}
@Override
public Iterator iterator() {
final Iterator mappedIterator = base.iterator();
final Set usedValues = ConcurrentHashMap.newKeySet();
return new Iterator() {
T next = findNext();
@Override
public boolean hasNext() {
return next != null;
}
@Override
public T next() {
if (next == null) {
throw new NoSuchElementException();
}
T result = next;
next = findNext();
return result;
}
private T findNext() {
long count = 0;
while (true) {
if (!mappedIterator.hasNext()) {
return null;
}
T next = mappedIterator.next();
if (!usedValues.contains(next)) {
usedValues.add(next);
return next;
} else {
if (++count > MAX_MISSES) {
String message =
String.format("Uniqueness filter missed more than %s times.", MAX_MISSES);
throw new TooManyFilterMissesException(message);
}
}
}
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy