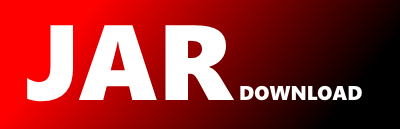
net.jqwik.engine.properties.arbitraries.randomized.UniqueGenerator Maven / Gradle / Ivy
package net.jqwik.engine.properties.arbitraries.randomized;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import net.jqwik.api.*;
import net.jqwik.engine.properties.shrinking.*;
public class UniqueGenerator implements RandomGenerator {
private static final long MAX_MISSES = 10000;
private final RandomGenerator toFilter;
private final Set usedValues = ConcurrentHashMap.newKeySet();
public UniqueGenerator(RandomGenerator toFilter) {
this.toFilter = toFilter;
}
@Override
public Shrinkable next(Random random) {
return nextUntilAccepted(random, r -> {
Shrinkable next = toFilter.next(r);
return new UniqueShrinkable<>(next, usedValues);
});
}
@Override
public String toString() {
return String.format("Unique [%s]", toFilter);
}
private Shrinkable nextUntilAccepted(Random random, Function> fetchShrinkable) {
long count = 0;
while (true) {
Shrinkable next = fetchShrinkable.apply(random);
if (usedValues.contains(next.value())) {
if (++count > MAX_MISSES) {
throw new TooManyFilterMissesException(String.format("%s missed more than %s times.", toString(), MAX_MISSES));
}
continue;
} else {
usedValues.add(next.value());
}
return next;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy