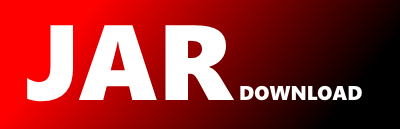
net.jqwik.spring.JqwikSpringReflectionSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jqwik-spring Show documentation
Show all versions of jqwik-spring Show documentation
Jqwik Spring support module
The newest version!
package net.jqwik.spring;
import java.lang.reflect.*;
import java.util.*;
/**
* This should somehow move to jqwik itself and be provided as a service to hooks
*
* TODO Remove as soon as jqwik provides this functionality
*/
class JqwikSpringReflectionSupport {
interface Applier {
void apply(Object instance) throws Exception;
}
static void applyToInstances(Object instance, Applier codeToApply) throws Exception {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy