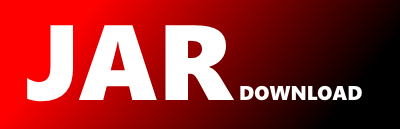
net.jqwik.spring.OutsideLifecycleMethodsHook Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jqwik-spring Show documentation
Show all versions of jqwik-spring Show documentation
Jqwik Spring support module
package net.jqwik.spring;
import java.lang.reflect.*;
import java.util.*;
import net.jqwik.api.lifecycle.*;
import org.springframework.test.context.*;
class OutsideLifecycleMethodsHook implements AroundTryHook {
@Override
public boolean appliesTo(Optional optionalElement) {
// Only apply to methods
return optionalElement.map(element -> element instanceof Method).orElse(false);
}
@Override
public TryExecutionResult aroundTry(TryLifecycleContext context, TryExecutor aTry, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy