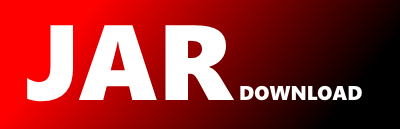
net.jradius.dictionary.vsa_acc.Attr_AccClearingCause Maven / Gradle / Ivy
// DO NOT EDIT THIS FILE DIRECTLY! - AUTOMATICALLY GENERATED
// Generated by: class net.jradius.freeradius.RadiusDictionary
// Generated on: Thu, 5 Jan 2017 15:56:13 +0200
package net.jradius.dictionary.vsa_acc;
import java.io.Serializable;
import java.util.LinkedHashMap;
import java.util.Map;
import net.jradius.packet.attribute.VSAttribute;
import net.jradius.packet.attribute.value.NamedValue;
/**
* Attribute Name: Acc-Clearing-Cause
* Attribute Type: 26
* Vendor Id: 5
* VSA Type: 15
* Value Type: NamedValue
* Possible Values:
*
* - Cause-unspecified (0)
*
- Unassigned-number (1)
*
- No-route-to-transit-network (2)
*
- No-route-to-destination (3)
*
- Channel-unacceptable (6)
*
- Call-awarded-being-delivered (7)
*
- Normal-clearing (16)
*
- User-busy (17)
*
- No-user-responding (18)
*
- User-alerted-no-answer (19)
*
- Call-rejected (21)
*
- Number-changed (22)
*
- Non-selected-user-clearing (26)
*
- Destination-out-of-order (27)
*
- Invalid-or-incomplete-number (28)
*
- Facility-rejected (29)
*
- Response-to-status-inquiry (30)
*
- Normal-unspecified-cause (31)
*
- No-circuit-or-channel-available (34)
*
- Network-out-of-order (38)
*
- Temporary-failure (41)
*
- Switching-equipment-congestion (42)
*
- Access-information-discarded (43)
*
- Circuit-or-channel-unavailable (44)
*
- Circuit-or-channed-preempted (45)
*
- Resources-unavailable (47)
*
- Quality-of-service-unavailable (49)
*
- Facility-not-subscribed (50)
*
- Outgoing-calls-barred (52)
*
- Incoming-calls-barred (54)
*
- Bearer-capability-unauthorized (57)
*
- Bearer-capability-not-available (58)
*
- Service-not-available (63)
*
- Bearer-capablity-not-implmented (65)
*
- Channel-type-not-implemented (66)
*
- Facility-not-implemented (69)
*
- Restrcted-digtal-infrmtion-only (70)
*
- Service-not-implemented (79)
*
- Invalid-call-reference (81)
*
- Identified-channel-doesnt-exist (82)
*
- Call-identify-in-use (84)
*
- No-call-suspended (85)
*
- Suspended-call-cleared (86)
*
- Incompatible-destination (88)
*
- Invalid-transit-network-selctin (91)
*
- Invalid-message (95)
*
- Mandtory-infrmtion-elment-miss (96)
*
- Message-not-implemented (97)
*
- Inopportune-message (98)
*
- Infrmtion-elemnt-not-implmented (99)
*
- Invlid-infrmtion-element-contnt (100)
*
- Message-incompatible-with-state (101)
*
- Recovery-on-timer-expiration (102)
*
- Mndtry-infrmtion-elmnt-lngt-err (103)
*
- Protocol-error (111)
*
- Interworking (127)
*
*
* @author class net.jradius.freeradius.RadiusDictionary
*/
public final class Attr_AccClearingCause extends VSAttribute
{
public static final String NAME = "Acc-Clearing-Cause";
public static final int VENDOR_ID = 5;
public static final int VSA_TYPE = 15;
public static final long TYPE = ((VENDOR_ID & 0xFFFF) << 16) | VSA_TYPE;
public static final long serialVersionUID = TYPE;
public static final Long Causeunspecified = new Long(0L);
public static final Long Unassignednumber = new Long(1L);
public static final Long Noroutetotransitnetwork = new Long(2L);
public static final Long Noroutetodestination = new Long(3L);
public static final Long Channelunacceptable = new Long(6L);
public static final Long Callawardedbeingdelivered = new Long(7L);
public static final Long Normalclearing = new Long(16L);
public static final Long Userbusy = new Long(17L);
public static final Long Nouserresponding = new Long(18L);
public static final Long Useralertednoanswer = new Long(19L);
public static final Long Callrejected = new Long(21L);
public static final Long Numberchanged = new Long(22L);
public static final Long Nonselecteduserclearing = new Long(26L);
public static final Long Destinationoutoforder = new Long(27L);
public static final Long Invalidorincompletenumber = new Long(28L);
public static final Long Facilityrejected = new Long(29L);
public static final Long Responsetostatusinquiry = new Long(30L);
public static final Long Normalunspecifiedcause = new Long(31L);
public static final Long Nocircuitorchannelavailable = new Long(34L);
public static final Long Networkoutoforder = new Long(38L);
public static final Long Temporaryfailure = new Long(41L);
public static final Long Switchingequipmentcongestion = new Long(42L);
public static final Long Accessinformationdiscarded = new Long(43L);
public static final Long Circuitorchannelunavailable = new Long(44L);
public static final Long Circuitorchannedpreempted = new Long(45L);
public static final Long Resourcesunavailable = new Long(47L);
public static final Long Qualityofserviceunavailable = new Long(49L);
public static final Long Facilitynotsubscribed = new Long(50L);
public static final Long Outgoingcallsbarred = new Long(52L);
public static final Long Incomingcallsbarred = new Long(54L);
public static final Long Bearercapabilityunauthorized = new Long(57L);
public static final Long Bearercapabilitynotavailable = new Long(58L);
public static final Long Servicenotavailable = new Long(63L);
public static final Long Bearercapablitynotimplmented = new Long(65L);
public static final Long Channeltypenotimplemented = new Long(66L);
public static final Long Facilitynotimplemented = new Long(69L);
public static final Long Restrcteddigtalinfrmtiononly = new Long(70L);
public static final Long Servicenotimplemented = new Long(79L);
public static final Long Invalidcallreference = new Long(81L);
public static final Long Identifiedchanneldoesntexist = new Long(82L);
public static final Long Callidentifyinuse = new Long(84L);
public static final Long Nocallsuspended = new Long(85L);
public static final Long Suspendedcallcleared = new Long(86L);
public static final Long Incompatibledestination = new Long(88L);
public static final Long Invalidtransitnetworkselctin = new Long(91L);
public static final Long Invalidmessage = new Long(95L);
public static final Long Mandtoryinfrmtionelmentmiss = new Long(96L);
public static final Long Messagenotimplemented = new Long(97L);
public static final Long Inopportunemessage = new Long(98L);
public static final Long Infrmtionelemntnotimplmented = new Long(99L);
public static final Long Invlidinfrmtionelementcontnt = new Long(100L);
public static final Long Messageincompatiblewithstate = new Long(101L);
public static final Long Recoveryontimerexpiration = new Long(102L);
public static final Long Mndtryinfrmtionelmntlngterr = new Long(103L);
public static final Long Protocolerror = new Long(111L);
public static final Long Interworking = new Long(127L);
@SuppressWarnings("serial")
protected class NamedValueMap implements NamedValue.NamedValueMap, Serializable
{
public Long[] knownValues = { new Long(0L),new Long(1L),new Long(2L),new Long(3L),new Long(6L),new Long(7L),new Long(16L),new Long(17L),new Long(18L),new Long(19L),new Long(21L),new Long(22L),new Long(26L),new Long(27L),new Long(28L),new Long(29L),new Long(30L),new Long(31L),new Long(34L),new Long(38L),new Long(41L),new Long(42L),new Long(43L),new Long(44L),new Long(45L),new Long(47L),new Long(49L),new Long(50L),new Long(52L),new Long(54L),new Long(57L),new Long(58L),new Long(63L),new Long(65L),new Long(66L),new Long(69L),new Long(70L),new Long(79L),new Long(81L),new Long(82L),new Long(84L),new Long(85L),new Long(86L),new Long(88L),new Long(91L),new Long(95L),new Long(96L),new Long(97L),new Long(98L),new Long(99L),new Long(100L),new Long(101L),new Long(102L),new Long(103L),new Long(111L),new Long(127L)};
public Long[] getKnownValues() { return knownValues; }
public Long getNamedValue(String name)
{
if ("Cause-unspecified".equals(name)) return new Long(0L);
if ("Unassigned-number".equals(name)) return new Long(1L);
if ("No-route-to-transit-network".equals(name)) return new Long(2L);
if ("No-route-to-destination".equals(name)) return new Long(3L);
if ("Channel-unacceptable".equals(name)) return new Long(6L);
if ("Call-awarded-being-delivered".equals(name)) return new Long(7L);
if ("Normal-clearing".equals(name)) return new Long(16L);
if ("User-busy".equals(name)) return new Long(17L);
if ("No-user-responding".equals(name)) return new Long(18L);
if ("User-alerted-no-answer".equals(name)) return new Long(19L);
if ("Call-rejected".equals(name)) return new Long(21L);
if ("Number-changed".equals(name)) return new Long(22L);
if ("Non-selected-user-clearing".equals(name)) return new Long(26L);
if ("Destination-out-of-order".equals(name)) return new Long(27L);
if ("Invalid-or-incomplete-number".equals(name)) return new Long(28L);
if ("Facility-rejected".equals(name)) return new Long(29L);
if ("Response-to-status-inquiry".equals(name)) return new Long(30L);
if ("Normal-unspecified-cause".equals(name)) return new Long(31L);
if ("No-circuit-or-channel-available".equals(name)) return new Long(34L);
if ("Network-out-of-order".equals(name)) return new Long(38L);
if ("Temporary-failure".equals(name)) return new Long(41L);
if ("Switching-equipment-congestion".equals(name)) return new Long(42L);
if ("Access-information-discarded".equals(name)) return new Long(43L);
if ("Circuit-or-channel-unavailable".equals(name)) return new Long(44L);
if ("Circuit-or-channed-preempted".equals(name)) return new Long(45L);
if ("Resources-unavailable".equals(name)) return new Long(47L);
if ("Quality-of-service-unavailable".equals(name)) return new Long(49L);
if ("Facility-not-subscribed".equals(name)) return new Long(50L);
if ("Outgoing-calls-barred".equals(name)) return new Long(52L);
if ("Incoming-calls-barred".equals(name)) return new Long(54L);
if ("Bearer-capability-unauthorized".equals(name)) return new Long(57L);
if ("Bearer-capability-not-available".equals(name)) return new Long(58L);
if ("Service-not-available".equals(name)) return new Long(63L);
if ("Bearer-capablity-not-implmented".equals(name)) return new Long(65L);
if ("Channel-type-not-implemented".equals(name)) return new Long(66L);
if ("Facility-not-implemented".equals(name)) return new Long(69L);
if ("Restrcted-digtal-infrmtion-only".equals(name)) return new Long(70L);
if ("Service-not-implemented".equals(name)) return new Long(79L);
if ("Invalid-call-reference".equals(name)) return new Long(81L);
if ("Identified-channel-doesnt-exist".equals(name)) return new Long(82L);
if ("Call-identify-in-use".equals(name)) return new Long(84L);
if ("No-call-suspended".equals(name)) return new Long(85L);
if ("Suspended-call-cleared".equals(name)) return new Long(86L);
if ("Incompatible-destination".equals(name)) return new Long(88L);
if ("Invalid-transit-network-selctin".equals(name)) return new Long(91L);
if ("Invalid-message".equals(name)) return new Long(95L);
if ("Mandtory-infrmtion-elment-miss".equals(name)) return new Long(96L);
if ("Message-not-implemented".equals(name)) return new Long(97L);
if ("Inopportune-message".equals(name)) return new Long(98L);
if ("Infrmtion-elemnt-not-implmented".equals(name)) return new Long(99L);
if ("Invlid-infrmtion-element-contnt".equals(name)) return new Long(100L);
if ("Message-incompatible-with-state".equals(name)) return new Long(101L);
if ("Recovery-on-timer-expiration".equals(name)) return new Long(102L);
if ("Mndtry-infrmtion-elmnt-lngt-err".equals(name)) return new Long(103L);
if ("Protocol-error".equals(name)) return new Long(111L);
if ("Interworking".equals(name)) return new Long(127L);
return null;
}
public String getNamedValue(Long value)
{
if (new Long(0L).equals(value)) return "Cause-unspecified";
if (new Long(1L).equals(value)) return "Unassigned-number";
if (new Long(2L).equals(value)) return "No-route-to-transit-network";
if (new Long(3L).equals(value)) return "No-route-to-destination";
if (new Long(6L).equals(value)) return "Channel-unacceptable";
if (new Long(7L).equals(value)) return "Call-awarded-being-delivered";
if (new Long(16L).equals(value)) return "Normal-clearing";
if (new Long(17L).equals(value)) return "User-busy";
if (new Long(18L).equals(value)) return "No-user-responding";
if (new Long(19L).equals(value)) return "User-alerted-no-answer";
if (new Long(21L).equals(value)) return "Call-rejected";
if (new Long(22L).equals(value)) return "Number-changed";
if (new Long(26L).equals(value)) return "Non-selected-user-clearing";
if (new Long(27L).equals(value)) return "Destination-out-of-order";
if (new Long(28L).equals(value)) return "Invalid-or-incomplete-number";
if (new Long(29L).equals(value)) return "Facility-rejected";
if (new Long(30L).equals(value)) return "Response-to-status-inquiry";
if (new Long(31L).equals(value)) return "Normal-unspecified-cause";
if (new Long(34L).equals(value)) return "No-circuit-or-channel-available";
if (new Long(38L).equals(value)) return "Network-out-of-order";
if (new Long(41L).equals(value)) return "Temporary-failure";
if (new Long(42L).equals(value)) return "Switching-equipment-congestion";
if (new Long(43L).equals(value)) return "Access-information-discarded";
if (new Long(44L).equals(value)) return "Circuit-or-channel-unavailable";
if (new Long(45L).equals(value)) return "Circuit-or-channed-preempted";
if (new Long(47L).equals(value)) return "Resources-unavailable";
if (new Long(49L).equals(value)) return "Quality-of-service-unavailable";
if (new Long(50L).equals(value)) return "Facility-not-subscribed";
if (new Long(52L).equals(value)) return "Outgoing-calls-barred";
if (new Long(54L).equals(value)) return "Incoming-calls-barred";
if (new Long(57L).equals(value)) return "Bearer-capability-unauthorized";
if (new Long(58L).equals(value)) return "Bearer-capability-not-available";
if (new Long(63L).equals(value)) return "Service-not-available";
if (new Long(65L).equals(value)) return "Bearer-capablity-not-implmented";
if (new Long(66L).equals(value)) return "Channel-type-not-implemented";
if (new Long(69L).equals(value)) return "Facility-not-implemented";
if (new Long(70L).equals(value)) return "Restrcted-digtal-infrmtion-only";
if (new Long(79L).equals(value)) return "Service-not-implemented";
if (new Long(81L).equals(value)) return "Invalid-call-reference";
if (new Long(82L).equals(value)) return "Identified-channel-doesnt-exist";
if (new Long(84L).equals(value)) return "Call-identify-in-use";
if (new Long(85L).equals(value)) return "No-call-suspended";
if (new Long(86L).equals(value)) return "Suspended-call-cleared";
if (new Long(88L).equals(value)) return "Incompatible-destination";
if (new Long(91L).equals(value)) return "Invalid-transit-network-selctin";
if (new Long(95L).equals(value)) return "Invalid-message";
if (new Long(96L).equals(value)) return "Mandtory-infrmtion-elment-miss";
if (new Long(97L).equals(value)) return "Message-not-implemented";
if (new Long(98L).equals(value)) return "Inopportune-message";
if (new Long(99L).equals(value)) return "Infrmtion-elemnt-not-implmented";
if (new Long(100L).equals(value)) return "Invlid-infrmtion-element-contnt";
if (new Long(101L).equals(value)) return "Message-incompatible-with-state";
if (new Long(102L).equals(value)) return "Recovery-on-timer-expiration";
if (new Long(103L).equals(value)) return "Mndtry-infrmtion-elmnt-lngt-err";
if (new Long(111L).equals(value)) return "Protocol-error";
if (new Long(127L).equals(value)) return "Interworking";
return null;
}
};
public static transient NamedValueMap map = null;
public void setup()
{
attributeName = NAME;
attributeType = 26;
vendorId = VENDOR_ID;
vsaAttributeType = VSA_TYPE;
attributeValue = new NamedValue(map != null ? map : (map = new NamedValueMap()));
}
public Attr_AccClearingCause()
{
setup();
}
public Attr_AccClearingCause(Serializable o)
{
setup(o);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy