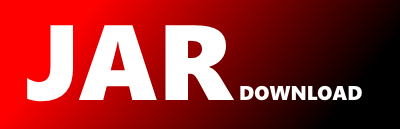
net.jradius.dictionary.vsa_acc.VSADictionaryImpl Maven / Gradle / Ivy
// DO NOT EDIT THIS FILE DIRECTLY! - AUTOMATICALLY GENERATED
// Generated by: class net.jradius.freeradius.RadiusDictionary
// Generated on: Thu, 5 Jan 2017 15:56:13 +0200
package net.jradius.dictionary.vsa_acc;
import java.util.Map;
import net.jradius.packet.attribute.VSADictionary;
/**
* Dictionary for package net.jradius.dictionary.vsa_acc
* @author class net.jradius.freeradius.RadiusDictionary
*/
public class VSADictionaryImpl implements VSADictionary
{
public String getVendorName() { return "Acc"; }
public void loadAttributes(Map> map)
{
map.put(new Long(1L), Attr_AccReasonCode.class);
map.put(new Long(2L), Attr_AccCcpOption.class);
map.put(new Long(3L), Attr_AccInputErrors.class);
map.put(new Long(4L), Attr_AccOutputErrors.class);
map.put(new Long(5L), Attr_AccAccessPartition.class);
map.put(new Long(6L), Attr_AccCustomerId.class);
map.put(new Long(7L), Attr_AccIpGatewayPri.class);
map.put(new Long(8L), Attr_AccIpGatewaySec.class);
map.put(new Long(9L), Attr_AccRoutePolicy.class);
map.put(new Long(10L), Attr_AccMLMLXAdminState.class);
map.put(new Long(11L), Attr_AccMLCallThreshold.class);
map.put(new Long(12L), Attr_AccMLClearThreshold.class);
map.put(new Long(13L), Attr_AccMLDampingFactor.class);
map.put(new Long(14L), Attr_AccTunnelSecret.class);
map.put(new Long(15L), Attr_AccClearingCause.class);
map.put(new Long(16L), Attr_AccClearingLocation.class);
map.put(new Long(17L), Attr_AccServiceProfile.class);
map.put(new Long(18L), Attr_AccRequestType.class);
map.put(new Long(19L), Attr_AccBridgingSupport.class);
map.put(new Long(20L), Attr_AccApsmOversubscribed.class);
map.put(new Long(21L), Attr_AccAcctOnOffReason.class);
map.put(new Long(22L), Attr_AccTunnelPort.class);
map.put(new Long(23L), Attr_AccDnsServerPri.class);
map.put(new Long(24L), Attr_AccDnsServerSec.class);
map.put(new Long(25L), Attr_AccNbnsServerPri.class);
map.put(new Long(26L), Attr_AccNbnsServerSec.class);
map.put(new Long(27L), Attr_AccDialPortIndex.class);
map.put(new Long(28L), Attr_AccIpCompression.class);
map.put(new Long(29L), Attr_AccIpxCompression.class);
map.put(new Long(30L), Attr_AccConnectTxSpeed.class);
map.put(new Long(31L), Attr_AccConnectRxSpeed.class);
map.put(new Long(32L), Attr_AccModemModulationType.class);
map.put(new Long(33L), Attr_AccModemErrorProtocol.class);
map.put(new Long(34L), Attr_AccCallbackDelay.class);
map.put(new Long(35L), Attr_AccCallbackNumValid.class);
map.put(new Long(36L), Attr_AccCallbackMode.class);
map.put(new Long(37L), Attr_AccCallbackCBCPType.class);
map.put(new Long(38L), Attr_AccDialoutAuthMode.class);
map.put(new Long(39L), Attr_AccDialoutAuthPassword.class);
map.put(new Long(40L), Attr_AccDialoutAuthUsername.class);
map.put(new Long(42L), Attr_AccAccessCommunity.class);
map.put(new Long(43L), Attr_AccVpsmRejectCause.class);
map.put(new Long(44L), Attr_AccAceToken.class);
map.put(new Long(45L), Attr_AccAceTokenTtl.class);
map.put(new Long(46L), Attr_AccIpPoolName.class);
map.put(new Long(47L), Attr_AccIgmpAdminState.class);
map.put(new Long(48L), Attr_AccIgmpVersion.class);
map.put(new Long(73L), Attr_AccMNHASecret.class);
map.put(new Long(98L), Attr_AccLocationId.class);
map.put(new Long(99L), Attr_AccCallingStationCategory.class);
}
public void loadAttributesNames(Map> map)
{
map.put(Attr_AccReasonCode.NAME, Attr_AccReasonCode.class);
map.put(Attr_AccCcpOption.NAME, Attr_AccCcpOption.class);
map.put(Attr_AccInputErrors.NAME, Attr_AccInputErrors.class);
map.put(Attr_AccOutputErrors.NAME, Attr_AccOutputErrors.class);
map.put(Attr_AccAccessPartition.NAME, Attr_AccAccessPartition.class);
map.put(Attr_AccCustomerId.NAME, Attr_AccCustomerId.class);
map.put(Attr_AccIpGatewayPri.NAME, Attr_AccIpGatewayPri.class);
map.put(Attr_AccIpGatewaySec.NAME, Attr_AccIpGatewaySec.class);
map.put(Attr_AccRoutePolicy.NAME, Attr_AccRoutePolicy.class);
map.put(Attr_AccMLMLXAdminState.NAME, Attr_AccMLMLXAdminState.class);
map.put(Attr_AccMLCallThreshold.NAME, Attr_AccMLCallThreshold.class);
map.put(Attr_AccMLClearThreshold.NAME, Attr_AccMLClearThreshold.class);
map.put(Attr_AccMLDampingFactor.NAME, Attr_AccMLDampingFactor.class);
map.put(Attr_AccTunnelSecret.NAME, Attr_AccTunnelSecret.class);
map.put(Attr_AccClearingCause.NAME, Attr_AccClearingCause.class);
map.put(Attr_AccClearingLocation.NAME, Attr_AccClearingLocation.class);
map.put(Attr_AccServiceProfile.NAME, Attr_AccServiceProfile.class);
map.put(Attr_AccRequestType.NAME, Attr_AccRequestType.class);
map.put(Attr_AccBridgingSupport.NAME, Attr_AccBridgingSupport.class);
map.put(Attr_AccApsmOversubscribed.NAME, Attr_AccApsmOversubscribed.class);
map.put(Attr_AccAcctOnOffReason.NAME, Attr_AccAcctOnOffReason.class);
map.put(Attr_AccTunnelPort.NAME, Attr_AccTunnelPort.class);
map.put(Attr_AccDnsServerPri.NAME, Attr_AccDnsServerPri.class);
map.put(Attr_AccDnsServerSec.NAME, Attr_AccDnsServerSec.class);
map.put(Attr_AccNbnsServerPri.NAME, Attr_AccNbnsServerPri.class);
map.put(Attr_AccNbnsServerSec.NAME, Attr_AccNbnsServerSec.class);
map.put(Attr_AccDialPortIndex.NAME, Attr_AccDialPortIndex.class);
map.put(Attr_AccIpCompression.NAME, Attr_AccIpCompression.class);
map.put(Attr_AccIpxCompression.NAME, Attr_AccIpxCompression.class);
map.put(Attr_AccConnectTxSpeed.NAME, Attr_AccConnectTxSpeed.class);
map.put(Attr_AccConnectRxSpeed.NAME, Attr_AccConnectRxSpeed.class);
map.put(Attr_AccModemModulationType.NAME, Attr_AccModemModulationType.class);
map.put(Attr_AccModemErrorProtocol.NAME, Attr_AccModemErrorProtocol.class);
map.put(Attr_AccCallbackDelay.NAME, Attr_AccCallbackDelay.class);
map.put(Attr_AccCallbackNumValid.NAME, Attr_AccCallbackNumValid.class);
map.put(Attr_AccCallbackMode.NAME, Attr_AccCallbackMode.class);
map.put(Attr_AccCallbackCBCPType.NAME, Attr_AccCallbackCBCPType.class);
map.put(Attr_AccDialoutAuthMode.NAME, Attr_AccDialoutAuthMode.class);
map.put(Attr_AccDialoutAuthPassword.NAME, Attr_AccDialoutAuthPassword.class);
map.put(Attr_AccDialoutAuthUsername.NAME, Attr_AccDialoutAuthUsername.class);
map.put(Attr_AccAccessCommunity.NAME, Attr_AccAccessCommunity.class);
map.put(Attr_AccVpsmRejectCause.NAME, Attr_AccVpsmRejectCause.class);
map.put(Attr_AccAceToken.NAME, Attr_AccAceToken.class);
map.put(Attr_AccAceTokenTtl.NAME, Attr_AccAceTokenTtl.class);
map.put(Attr_AccIpPoolName.NAME, Attr_AccIpPoolName.class);
map.put(Attr_AccIgmpAdminState.NAME, Attr_AccIgmpAdminState.class);
map.put(Attr_AccIgmpVersion.NAME, Attr_AccIgmpVersion.class);
map.put(Attr_AccMNHASecret.NAME, Attr_AccMNHASecret.class);
map.put(Attr_AccLocationId.NAME, Attr_AccLocationId.class);
map.put(Attr_AccCallingStationCategory.NAME, Attr_AccCallingStationCategory.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy