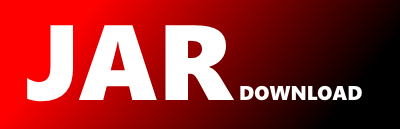
net.jradius.dictionary.vsa_acme.VSADictionaryImpl Maven / Gradle / Ivy
The newest version!
// DO NOT EDIT THIS FILE DIRECTLY! - AUTOMATICALLY GENERATED
// Generated by: class net.jradius.freeradius.RadiusDictionary
// Generated on: Thu, 5 Jan 2017 15:56:13 +0200
package net.jradius.dictionary.vsa_acme;
import java.util.Map;
import net.jradius.packet.attribute.VSADictionary;
/**
* Dictionary for package net.jradius.dictionary.vsa_acme
* @author class net.jradius.freeradius.RadiusDictionary
*/
public class VSADictionaryImpl implements VSADictionary
{
public String getVendorName() { return "Acme"; }
public void loadAttributes(Map> map)
{
map.put(new Long(1L), Attr_AcmeFlowIDFS1F.class);
map.put(new Long(2L), Attr_AcmeFlowTypeFS1F.class);
map.put(new Long(3L), Attr_AcmeSessionIngressCallId.class);
map.put(new Long(4L), Attr_AcmeSessionEgressCallId.class);
map.put(new Long(10L), Attr_AcmeFlowInRealmFS1F.class);
map.put(new Long(11L), Attr_AcmeFlowInSrcAddrFS1F.class);
map.put(new Long(12L), Attr_AcmeFlowInSrcPortFS1F.class);
map.put(new Long(13L), Attr_AcmeFlowInDstAddrFS1F.class);
map.put(new Long(14L), Attr_AcmeFlowInDstPortFS1F.class);
map.put(new Long(20L), Attr_AcmeFlowOutRealmFS1F.class);
map.put(new Long(21L), Attr_AcmeFlowOutSrcAddrFS1F.class);
map.put(new Long(22L), Attr_AcmeFlowOutSrcPortFS1F.class);
map.put(new Long(23L), Attr_AcmeFlowOutDstAddrFS1F.class);
map.put(new Long(24L), Attr_AcmeFlowOutDstPortFS1F.class);
map.put(new Long(28L), Attr_AcmeCallingOctetsFS1.class);
map.put(new Long(29L), Attr_AcmeCallingPacketsFS1.class);
map.put(new Long(32L), Attr_AcmeCallingRTCPPacketsLostFS1.class);
map.put(new Long(33L), Attr_AcmeCallingRTCPAvgJitterFS1.class);
map.put(new Long(34L), Attr_AcmeCallingRTCPAvgLatencyFS1.class);
map.put(new Long(35L), Attr_AcmeCallingRTCPMaxJitterFS1.class);
map.put(new Long(36L), Attr_AcmeCallingRTCPMaxLatencyFS1.class);
map.put(new Long(37L), Attr_AcmeCallingRTPPacketsLostFS1.class);
map.put(new Long(38L), Attr_AcmeCallingRTPAvgJitterFS1.class);
map.put(new Long(39L), Attr_AcmeCallingRTPMaxJitterFS1.class);
map.put(new Long(40L), Attr_AcmeSessionGenericId.class);
map.put(new Long(41L), Attr_AcmeSessionIngressRealm.class);
map.put(new Long(42L), Attr_AcmeSessionEgressRealm.class);
map.put(new Long(43L), Attr_AcmeSessionProtocolType.class);
map.put(new Long(44L), Attr_AcmeCalledOctetsFS1.class);
map.put(new Long(45L), Attr_AcmeCalledPacketsFS1.class);
map.put(new Long(46L), Attr_AcmeCalledRTCPPacketsLostFS1.class);
map.put(new Long(47L), Attr_AcmeCalledRTCPAvgJitterFS1.class);
map.put(new Long(48L), Attr_AcmeCalledRTCPAvgLatencyFS1.class);
map.put(new Long(49L), Attr_AcmeCalledRTCPMaxJitterFS1.class);
map.put(new Long(50L), Attr_AcmeCalledRTCPMaxLatencyFS1.class);
map.put(new Long(51L), Attr_AcmeCalledRTPPacketsLostFS1.class);
map.put(new Long(52L), Attr_AcmeCalledRTPAvgJitterFS1.class);
map.put(new Long(53L), Attr_AcmeCalledRTPMaxJitterFS1.class);
map.put(new Long(54L), Attr_AcmeSessionChargingVector.class);
map.put(new Long(55L), Attr_AcmeSessionChargingFunctionAddress.class);
map.put(new Long(56L), Attr_AcmeFirmwareVersion.class);
map.put(new Long(57L), Attr_AcmeLocalTimeZone.class);
map.put(new Long(58L), Attr_AcmePostDialDelay.class);
map.put(new Long(59L), Attr_AcmeCDRSequenceNumber.class);
map.put(new Long(60L), Attr_AcmeSessionDisposition.class);
map.put(new Long(61L), Attr_AcmeDisconnectInitiator.class);
map.put(new Long(62L), Attr_AcmeDisconnectCause.class);
map.put(new Long(63L), Attr_AcmeIntermediateTime.class);
map.put(new Long(64L), Attr_AcmePrimaryRoutingNumber.class);
map.put(new Long(65L), Attr_AcmeOriginatingTrunkGroup.class);
map.put(new Long(66L), Attr_AcmeTerminatingTrunkGroup.class);
map.put(new Long(67L), Attr_AcmeOriginatingTrunkContext.class);
map.put(new Long(68L), Attr_AcmeTerminatingTrunkContext.class);
map.put(new Long(69L), Attr_AcmePAssertedID.class);
map.put(new Long(70L), Attr_AcmeSIPDiversion.class);
map.put(new Long(71L), Attr_AcmeSIPStatus.class);
map.put(new Long(74L), Attr_AcmeIngressLocalAddr.class);
map.put(new Long(75L), Attr_AcmeIngressRemoteAddr.class);
map.put(new Long(76L), Attr_AcmeEgressLocalAddr.class);
map.put(new Long(77L), Attr_AcmeEgressRemoteAddr.class);
map.put(new Long(78L), Attr_AcmeFlowIDFS1R.class);
map.put(new Long(79L), Attr_AcmeFlowTypeFS1R.class);
map.put(new Long(80L), Attr_AcmeFlowInRealmFS1R.class);
map.put(new Long(81L), Attr_AcmeFlowInSrcAddrFS1R.class);
map.put(new Long(82L), Attr_AcmeFlowInSrcPortFS1R.class);
map.put(new Long(83L), Attr_AcmeFlowInDstAddrFS1R.class);
map.put(new Long(84L), Attr_AcmeFlowInDstPortFS1R.class);
map.put(new Long(85L), Attr_AcmeFlowOutRealmFS1R.class);
map.put(new Long(86L), Attr_AcmeFlowOutSrcAddrFS1R.class);
map.put(new Long(87L), Attr_AcmeFlowOutSrcPortFS1R.class);
map.put(new Long(88L), Attr_AcmeFlowOutDstAddrFS1R.class);
map.put(new Long(89L), Attr_AcmeFlowOutDstPortFS1R.class);
map.put(new Long(90L), Attr_AcmeFlowIDFS2F.class);
map.put(new Long(91L), Attr_AcmeFlowTypeFS2F.class);
map.put(new Long(92L), Attr_AcmeFlowInRealmFS2F.class);
map.put(new Long(93L), Attr_AcmeFlowInSrcAddrFS2F.class);
map.put(new Long(94L), Attr_AcmeFlowInSrcPortFS2F.class);
map.put(new Long(95L), Attr_AcmeFlowInDstAddrFS2F.class);
map.put(new Long(96L), Attr_AcmeFlowInDstPortFS2F.class);
map.put(new Long(97L), Attr_AcmeFlowOutRealmFS2F.class);
map.put(new Long(98L), Attr_AcmeFlowOutSrcAddrFS2F.class);
map.put(new Long(99L), Attr_AcmeFlowOutSrcPortFS2F.class);
map.put(new Long(100L), Attr_AcmeFlowOutDstAddrFS2F.class);
map.put(new Long(101L), Attr_AcmeFlowOutDstPortFS2F.class);
map.put(new Long(102L), Attr_AcmeCallingOctetsFS2.class);
map.put(new Long(103L), Attr_AcmeCallingPacketsFS2.class);
map.put(new Long(104L), Attr_AcmeCallingRTCPPacketsLostFS2.class);
map.put(new Long(105L), Attr_AcmeCallingRTCPAvgJitterFS2.class);
map.put(new Long(106L), Attr_AcmeCallingRTCPAvgLatencyFS2.class);
map.put(new Long(107L), Attr_AcmeCallingRTCPMaxJitterFS2.class);
map.put(new Long(108L), Attr_AcmeCallingRTCPMaxLatencyFS2.class);
map.put(new Long(109L), Attr_AcmeCallingRTPPacketsLostFS2.class);
map.put(new Long(110L), Attr_AcmeCallingRTPAvgJitterFS2.class);
map.put(new Long(111L), Attr_AcmeCallingRTPMaxJitterFS2.class);
map.put(new Long(112L), Attr_AcmeFlowIDFS2R.class);
map.put(new Long(113L), Attr_AcmeFlowTypeFS2R.class);
map.put(new Long(114L), Attr_AcmeFlowInRealmFS2R.class);
map.put(new Long(115L), Attr_AcmeFlowInSrcAddrFS2R.class);
map.put(new Long(116L), Attr_AcmeFlowInSrcPortFS2R.class);
map.put(new Long(117L), Attr_AcmeFlowInDstAddrFS2R.class);
map.put(new Long(118L), Attr_AcmeFlowInDstPortFS2R.class);
map.put(new Long(119L), Attr_AcmeFlowOutRealmFS2R.class);
map.put(new Long(120L), Attr_AcmeFlowOutSrcAddrFS2R.class);
map.put(new Long(121L), Attr_AcmeFlowOutSrcPortFS2R.class);
map.put(new Long(122L), Attr_AcmeFlowOutDstAddrFS2R.class);
map.put(new Long(123L), Attr_AcmeFlowOutDstPortFS2R.class);
map.put(new Long(124L), Attr_AcmeCalledOctetsFS2.class);
map.put(new Long(125L), Attr_AcmeCalledPacketsFS2.class);
map.put(new Long(126L), Attr_AcmeCalledRTCPPacketsLostFS2.class);
map.put(new Long(127L), Attr_AcmeCalledRTCPAvgJitterFS2.class);
map.put(new Long(128L), Attr_AcmeCalledRTCPAvgLatencyFS2.class);
map.put(new Long(129L), Attr_AcmeCalledRTCPMaxJitterFS2.class);
map.put(new Long(130L), Attr_AcmeCalledRTCPMaxLatencyFS2.class);
map.put(new Long(131L), Attr_AcmeCalledRTPPacketsLostFS2.class);
map.put(new Long(132L), Attr_AcmeCalledRTPAvgJitterFS2.class);
map.put(new Long(133L), Attr_AcmeCalledRTPMaxJitterFS2.class);
map.put(new Long(134L), Attr_AcmeEgressFinalRoutingNumber.class);
map.put(new Long(135L), Attr_AcmeSessionIngressRPH.class);
map.put(new Long(136L), Attr_AcmeSessionEgressRPH.class);
map.put(new Long(137L), Attr_AcmeIngressNetworkInterfaceId.class);
map.put(new Long(138L), Attr_AcmeIngressVlanTagValue.class);
map.put(new Long(139L), Attr_AcmeEgressNetworkInterfaceId.class);
map.put(new Long(140L), Attr_AcmeEgressVlanTagValue.class);
map.put(new Long(141L), Attr_AcmeReferCallTransferId.class);
map.put(new Long(142L), Attr_AcmeFlowMediaTypeFS1F.class);
map.put(new Long(143L), Attr_AcmeFlowMediaTypeFS1R.class);
map.put(new Long(144L), Attr_AcmeFlowMediaTypeFS2F.class);
map.put(new Long(145L), Attr_AcmeFlowMediaTypeFS2R.class);
map.put(new Long(146L), Attr_AcmeFlowPTimeFS1F.class);
map.put(new Long(147L), Attr_AcmeFlowPTimeFS1R.class);
map.put(new Long(148L), Attr_AcmeFlowPTimeFS2F.class);
map.put(new Long(149L), Attr_AcmeFlowPTimeFS2R.class);
map.put(new Long(150L), Attr_AcmeSessionMediaProcess.class);
map.put(new Long(151L), Attr_AcmeCallingRFactor.class);
map.put(new Long(152L), Attr_AcmeCallingMOS.class);
map.put(new Long(153L), Attr_AcmeCalledRFactor.class);
map.put(new Long(154L), Attr_AcmeCalledMOS.class);
map.put(new Long(155L), Attr_AcmeFlowInSrcIPv6AddrFS1F.class);
map.put(new Long(156L), Attr_AcmeFlowInDstIPv6AddrFS1F.class);
map.put(new Long(157L), Attr_AcmeFlowOutSrcIPv6AddrFS1F.class);
map.put(new Long(158L), Attr_AcmeFlowOutDstIPv6AddrFS1F.class);
map.put(new Long(159L), Attr_AcmeFlowInSrcIPv6AddrFS1R.class);
map.put(new Long(160L), Attr_AcmeFlowInDstIPv6AddrFS1R.class);
map.put(new Long(161L), Attr_AcmeFlowOutSrcIPv6AddrFS1R.class);
map.put(new Long(162L), Attr_AcmeFlowOutDstIPv6AddrFS1R.class);
map.put(new Long(163L), Attr_AcmeFlowInSrcIPv6AddrFS2F.class);
map.put(new Long(164L), Attr_AcmeFlowInDstIPv6AddrFS2F.class);
map.put(new Long(165L), Attr_AcmeFlowOutSrcIPv6AddrFS2F.class);
map.put(new Long(166L), Attr_AcmeFlowOutDstIPv6AddrFS2F.class);
map.put(new Long(167L), Attr_AcmeFlowInSrcIPv6AddrFS2R.class);
map.put(new Long(168L), Attr_AcmeFlowInDstIPv6AddrFS2R.class);
map.put(new Long(169L), Attr_AcmeFlowOutSrcIPv6AddrFS2R.class);
map.put(new Long(170L), Attr_AcmeFlowOutDstIPv6AddrFS2R.class);
map.put(new Long(171L), Attr_AcmeSessionForkedCallId.class);
map.put(new Long(200L), Attr_AcmeCustomVSA200.class);
map.put(new Long(201L), Attr_AcmeCustomVSA201.class);
map.put(new Long(202L), Attr_AcmeCustomVSA202.class);
map.put(new Long(203L), Attr_AcmeCustomVSA203.class);
map.put(new Long(204L), Attr_AcmeCustomVSA204.class);
map.put(new Long(205L), Attr_AcmeCustomVSA205.class);
map.put(new Long(206L), Attr_AcmeCustomVSA206.class);
map.put(new Long(207L), Attr_AcmeCustomVSA207.class);
map.put(new Long(208L), Attr_AcmeCustomVSA208.class);
map.put(new Long(209L), Attr_AcmeCustomVSA209.class);
map.put(new Long(210L), Attr_AcmeCustomVSA210.class);
map.put(new Long(211L), Attr_AcmeCustomVSA211.class);
map.put(new Long(212L), Attr_AcmeCustomVSA212.class);
map.put(new Long(213L), Attr_AcmeCustomVSA213.class);
map.put(new Long(214L), Attr_AcmeCustomVSA214.class);
map.put(new Long(215L), Attr_AcmeCustomVSA215.class);
map.put(new Long(216L), Attr_AcmeCustomVSA216.class);
map.put(new Long(217L), Attr_AcmeCustomVSA217.class);
map.put(new Long(218L), Attr_AcmeCustomVSA218.class);
map.put(new Long(219L), Attr_AcmeCustomVSA219.class);
map.put(new Long(220L), Attr_AcmeCustomVSA220.class);
map.put(new Long(221L), Attr_AcmeCustomVSA221.class);
map.put(new Long(222L), Attr_AcmeCustomVSA222.class);
map.put(new Long(223L), Attr_AcmeCustomVSA223.class);
map.put(new Long(224L), Attr_AcmeCustomVSA224.class);
map.put(new Long(225L), Attr_AcmeCustomVSA225.class);
map.put(new Long(226L), Attr_AcmeCustomVSA226.class);
map.put(new Long(227L), Attr_AcmeCustomVSA227.class);
map.put(new Long(228L), Attr_AcmeCustomVSA228.class);
map.put(new Long(229L), Attr_AcmeCustomVSA229.class);
map.put(new Long(230L), Attr_AcmeCustomVSA230.class);
map.put(new Long(254L), Attr_AcmeUserClass.class);
}
public void loadAttributesNames(Map> map)
{
map.put(Attr_AcmeFlowIDFS1F.NAME, Attr_AcmeFlowIDFS1F.class);
map.put(Attr_AcmeFlowTypeFS1F.NAME, Attr_AcmeFlowTypeFS1F.class);
map.put(Attr_AcmeSessionIngressCallId.NAME, Attr_AcmeSessionIngressCallId.class);
map.put(Attr_AcmeSessionEgressCallId.NAME, Attr_AcmeSessionEgressCallId.class);
map.put(Attr_AcmeFlowInRealmFS1F.NAME, Attr_AcmeFlowInRealmFS1F.class);
map.put(Attr_AcmeFlowInSrcAddrFS1F.NAME, Attr_AcmeFlowInSrcAddrFS1F.class);
map.put(Attr_AcmeFlowInSrcPortFS1F.NAME, Attr_AcmeFlowInSrcPortFS1F.class);
map.put(Attr_AcmeFlowInDstAddrFS1F.NAME, Attr_AcmeFlowInDstAddrFS1F.class);
map.put(Attr_AcmeFlowInDstPortFS1F.NAME, Attr_AcmeFlowInDstPortFS1F.class);
map.put(Attr_AcmeFlowOutRealmFS1F.NAME, Attr_AcmeFlowOutRealmFS1F.class);
map.put(Attr_AcmeFlowOutSrcAddrFS1F.NAME, Attr_AcmeFlowOutSrcAddrFS1F.class);
map.put(Attr_AcmeFlowOutSrcPortFS1F.NAME, Attr_AcmeFlowOutSrcPortFS1F.class);
map.put(Attr_AcmeFlowOutDstAddrFS1F.NAME, Attr_AcmeFlowOutDstAddrFS1F.class);
map.put(Attr_AcmeFlowOutDstPortFS1F.NAME, Attr_AcmeFlowOutDstPortFS1F.class);
map.put(Attr_AcmeCallingOctetsFS1.NAME, Attr_AcmeCallingOctetsFS1.class);
map.put(Attr_AcmeCallingPacketsFS1.NAME, Attr_AcmeCallingPacketsFS1.class);
map.put(Attr_AcmeCallingRTCPPacketsLostFS1.NAME, Attr_AcmeCallingRTCPPacketsLostFS1.class);
map.put(Attr_AcmeCallingRTCPAvgJitterFS1.NAME, Attr_AcmeCallingRTCPAvgJitterFS1.class);
map.put(Attr_AcmeCallingRTCPAvgLatencyFS1.NAME, Attr_AcmeCallingRTCPAvgLatencyFS1.class);
map.put(Attr_AcmeCallingRTCPMaxJitterFS1.NAME, Attr_AcmeCallingRTCPMaxJitterFS1.class);
map.put(Attr_AcmeCallingRTCPMaxLatencyFS1.NAME, Attr_AcmeCallingRTCPMaxLatencyFS1.class);
map.put(Attr_AcmeCallingRTPPacketsLostFS1.NAME, Attr_AcmeCallingRTPPacketsLostFS1.class);
map.put(Attr_AcmeCallingRTPAvgJitterFS1.NAME, Attr_AcmeCallingRTPAvgJitterFS1.class);
map.put(Attr_AcmeCallingRTPMaxJitterFS1.NAME, Attr_AcmeCallingRTPMaxJitterFS1.class);
map.put(Attr_AcmeSessionGenericId.NAME, Attr_AcmeSessionGenericId.class);
map.put(Attr_AcmeSessionIngressRealm.NAME, Attr_AcmeSessionIngressRealm.class);
map.put(Attr_AcmeSessionEgressRealm.NAME, Attr_AcmeSessionEgressRealm.class);
map.put(Attr_AcmeSessionProtocolType.NAME, Attr_AcmeSessionProtocolType.class);
map.put(Attr_AcmeCalledOctetsFS1.NAME, Attr_AcmeCalledOctetsFS1.class);
map.put(Attr_AcmeCalledPacketsFS1.NAME, Attr_AcmeCalledPacketsFS1.class);
map.put(Attr_AcmeCalledRTCPPacketsLostFS1.NAME, Attr_AcmeCalledRTCPPacketsLostFS1.class);
map.put(Attr_AcmeCalledRTCPAvgJitterFS1.NAME, Attr_AcmeCalledRTCPAvgJitterFS1.class);
map.put(Attr_AcmeCalledRTCPAvgLatencyFS1.NAME, Attr_AcmeCalledRTCPAvgLatencyFS1.class);
map.put(Attr_AcmeCalledRTCPMaxJitterFS1.NAME, Attr_AcmeCalledRTCPMaxJitterFS1.class);
map.put(Attr_AcmeCalledRTCPMaxLatencyFS1.NAME, Attr_AcmeCalledRTCPMaxLatencyFS1.class);
map.put(Attr_AcmeCalledRTPPacketsLostFS1.NAME, Attr_AcmeCalledRTPPacketsLostFS1.class);
map.put(Attr_AcmeCalledRTPAvgJitterFS1.NAME, Attr_AcmeCalledRTPAvgJitterFS1.class);
map.put(Attr_AcmeCalledRTPMaxJitterFS1.NAME, Attr_AcmeCalledRTPMaxJitterFS1.class);
map.put(Attr_AcmeSessionChargingVector.NAME, Attr_AcmeSessionChargingVector.class);
map.put(Attr_AcmeSessionChargingFunctionAddress.NAME, Attr_AcmeSessionChargingFunctionAddress.class);
map.put(Attr_AcmeFirmwareVersion.NAME, Attr_AcmeFirmwareVersion.class);
map.put(Attr_AcmeLocalTimeZone.NAME, Attr_AcmeLocalTimeZone.class);
map.put(Attr_AcmePostDialDelay.NAME, Attr_AcmePostDialDelay.class);
map.put(Attr_AcmeCDRSequenceNumber.NAME, Attr_AcmeCDRSequenceNumber.class);
map.put(Attr_AcmeSessionDisposition.NAME, Attr_AcmeSessionDisposition.class);
map.put(Attr_AcmeDisconnectInitiator.NAME, Attr_AcmeDisconnectInitiator.class);
map.put(Attr_AcmeDisconnectCause.NAME, Attr_AcmeDisconnectCause.class);
map.put(Attr_AcmeIntermediateTime.NAME, Attr_AcmeIntermediateTime.class);
map.put(Attr_AcmePrimaryRoutingNumber.NAME, Attr_AcmePrimaryRoutingNumber.class);
map.put(Attr_AcmeOriginatingTrunkGroup.NAME, Attr_AcmeOriginatingTrunkGroup.class);
map.put(Attr_AcmeTerminatingTrunkGroup.NAME, Attr_AcmeTerminatingTrunkGroup.class);
map.put(Attr_AcmeOriginatingTrunkContext.NAME, Attr_AcmeOriginatingTrunkContext.class);
map.put(Attr_AcmeTerminatingTrunkContext.NAME, Attr_AcmeTerminatingTrunkContext.class);
map.put(Attr_AcmePAssertedID.NAME, Attr_AcmePAssertedID.class);
map.put(Attr_AcmeSIPDiversion.NAME, Attr_AcmeSIPDiversion.class);
map.put(Attr_AcmeSIPStatus.NAME, Attr_AcmeSIPStatus.class);
map.put(Attr_AcmeIngressLocalAddr.NAME, Attr_AcmeIngressLocalAddr.class);
map.put(Attr_AcmeIngressRemoteAddr.NAME, Attr_AcmeIngressRemoteAddr.class);
map.put(Attr_AcmeEgressLocalAddr.NAME, Attr_AcmeEgressLocalAddr.class);
map.put(Attr_AcmeEgressRemoteAddr.NAME, Attr_AcmeEgressRemoteAddr.class);
map.put(Attr_AcmeFlowIDFS1R.NAME, Attr_AcmeFlowIDFS1R.class);
map.put(Attr_AcmeFlowTypeFS1R.NAME, Attr_AcmeFlowTypeFS1R.class);
map.put(Attr_AcmeFlowInRealmFS1R.NAME, Attr_AcmeFlowInRealmFS1R.class);
map.put(Attr_AcmeFlowInSrcAddrFS1R.NAME, Attr_AcmeFlowInSrcAddrFS1R.class);
map.put(Attr_AcmeFlowInSrcPortFS1R.NAME, Attr_AcmeFlowInSrcPortFS1R.class);
map.put(Attr_AcmeFlowInDstAddrFS1R.NAME, Attr_AcmeFlowInDstAddrFS1R.class);
map.put(Attr_AcmeFlowInDstPortFS1R.NAME, Attr_AcmeFlowInDstPortFS1R.class);
map.put(Attr_AcmeFlowOutRealmFS1R.NAME, Attr_AcmeFlowOutRealmFS1R.class);
map.put(Attr_AcmeFlowOutSrcAddrFS1R.NAME, Attr_AcmeFlowOutSrcAddrFS1R.class);
map.put(Attr_AcmeFlowOutSrcPortFS1R.NAME, Attr_AcmeFlowOutSrcPortFS1R.class);
map.put(Attr_AcmeFlowOutDstAddrFS1R.NAME, Attr_AcmeFlowOutDstAddrFS1R.class);
map.put(Attr_AcmeFlowOutDstPortFS1R.NAME, Attr_AcmeFlowOutDstPortFS1R.class);
map.put(Attr_AcmeFlowIDFS2F.NAME, Attr_AcmeFlowIDFS2F.class);
map.put(Attr_AcmeFlowTypeFS2F.NAME, Attr_AcmeFlowTypeFS2F.class);
map.put(Attr_AcmeFlowInRealmFS2F.NAME, Attr_AcmeFlowInRealmFS2F.class);
map.put(Attr_AcmeFlowInSrcAddrFS2F.NAME, Attr_AcmeFlowInSrcAddrFS2F.class);
map.put(Attr_AcmeFlowInSrcPortFS2F.NAME, Attr_AcmeFlowInSrcPortFS2F.class);
map.put(Attr_AcmeFlowInDstAddrFS2F.NAME, Attr_AcmeFlowInDstAddrFS2F.class);
map.put(Attr_AcmeFlowInDstPortFS2F.NAME, Attr_AcmeFlowInDstPortFS2F.class);
map.put(Attr_AcmeFlowOutRealmFS2F.NAME, Attr_AcmeFlowOutRealmFS2F.class);
map.put(Attr_AcmeFlowOutSrcAddrFS2F.NAME, Attr_AcmeFlowOutSrcAddrFS2F.class);
map.put(Attr_AcmeFlowOutSrcPortFS2F.NAME, Attr_AcmeFlowOutSrcPortFS2F.class);
map.put(Attr_AcmeFlowOutDstAddrFS2F.NAME, Attr_AcmeFlowOutDstAddrFS2F.class);
map.put(Attr_AcmeFlowOutDstPortFS2F.NAME, Attr_AcmeFlowOutDstPortFS2F.class);
map.put(Attr_AcmeCallingOctetsFS2.NAME, Attr_AcmeCallingOctetsFS2.class);
map.put(Attr_AcmeCallingPacketsFS2.NAME, Attr_AcmeCallingPacketsFS2.class);
map.put(Attr_AcmeCallingRTCPPacketsLostFS2.NAME, Attr_AcmeCallingRTCPPacketsLostFS2.class);
map.put(Attr_AcmeCallingRTCPAvgJitterFS2.NAME, Attr_AcmeCallingRTCPAvgJitterFS2.class);
map.put(Attr_AcmeCallingRTCPAvgLatencyFS2.NAME, Attr_AcmeCallingRTCPAvgLatencyFS2.class);
map.put(Attr_AcmeCallingRTCPMaxJitterFS2.NAME, Attr_AcmeCallingRTCPMaxJitterFS2.class);
map.put(Attr_AcmeCallingRTCPMaxLatencyFS2.NAME, Attr_AcmeCallingRTCPMaxLatencyFS2.class);
map.put(Attr_AcmeCallingRTPPacketsLostFS2.NAME, Attr_AcmeCallingRTPPacketsLostFS2.class);
map.put(Attr_AcmeCallingRTPAvgJitterFS2.NAME, Attr_AcmeCallingRTPAvgJitterFS2.class);
map.put(Attr_AcmeCallingRTPMaxJitterFS2.NAME, Attr_AcmeCallingRTPMaxJitterFS2.class);
map.put(Attr_AcmeFlowIDFS2R.NAME, Attr_AcmeFlowIDFS2R.class);
map.put(Attr_AcmeFlowTypeFS2R.NAME, Attr_AcmeFlowTypeFS2R.class);
map.put(Attr_AcmeFlowInRealmFS2R.NAME, Attr_AcmeFlowInRealmFS2R.class);
map.put(Attr_AcmeFlowInSrcAddrFS2R.NAME, Attr_AcmeFlowInSrcAddrFS2R.class);
map.put(Attr_AcmeFlowInSrcPortFS2R.NAME, Attr_AcmeFlowInSrcPortFS2R.class);
map.put(Attr_AcmeFlowInDstAddrFS2R.NAME, Attr_AcmeFlowInDstAddrFS2R.class);
map.put(Attr_AcmeFlowInDstPortFS2R.NAME, Attr_AcmeFlowInDstPortFS2R.class);
map.put(Attr_AcmeFlowOutRealmFS2R.NAME, Attr_AcmeFlowOutRealmFS2R.class);
map.put(Attr_AcmeFlowOutSrcAddrFS2R.NAME, Attr_AcmeFlowOutSrcAddrFS2R.class);
map.put(Attr_AcmeFlowOutSrcPortFS2R.NAME, Attr_AcmeFlowOutSrcPortFS2R.class);
map.put(Attr_AcmeFlowOutDstAddrFS2R.NAME, Attr_AcmeFlowOutDstAddrFS2R.class);
map.put(Attr_AcmeFlowOutDstPortFS2R.NAME, Attr_AcmeFlowOutDstPortFS2R.class);
map.put(Attr_AcmeCalledOctetsFS2.NAME, Attr_AcmeCalledOctetsFS2.class);
map.put(Attr_AcmeCalledPacketsFS2.NAME, Attr_AcmeCalledPacketsFS2.class);
map.put(Attr_AcmeCalledRTCPPacketsLostFS2.NAME, Attr_AcmeCalledRTCPPacketsLostFS2.class);
map.put(Attr_AcmeCalledRTCPAvgJitterFS2.NAME, Attr_AcmeCalledRTCPAvgJitterFS2.class);
map.put(Attr_AcmeCalledRTCPAvgLatencyFS2.NAME, Attr_AcmeCalledRTCPAvgLatencyFS2.class);
map.put(Attr_AcmeCalledRTCPMaxJitterFS2.NAME, Attr_AcmeCalledRTCPMaxJitterFS2.class);
map.put(Attr_AcmeCalledRTCPMaxLatencyFS2.NAME, Attr_AcmeCalledRTCPMaxLatencyFS2.class);
map.put(Attr_AcmeCalledRTPPacketsLostFS2.NAME, Attr_AcmeCalledRTPPacketsLostFS2.class);
map.put(Attr_AcmeCalledRTPAvgJitterFS2.NAME, Attr_AcmeCalledRTPAvgJitterFS2.class);
map.put(Attr_AcmeCalledRTPMaxJitterFS2.NAME, Attr_AcmeCalledRTPMaxJitterFS2.class);
map.put(Attr_AcmeEgressFinalRoutingNumber.NAME, Attr_AcmeEgressFinalRoutingNumber.class);
map.put(Attr_AcmeSessionIngressRPH.NAME, Attr_AcmeSessionIngressRPH.class);
map.put(Attr_AcmeSessionEgressRPH.NAME, Attr_AcmeSessionEgressRPH.class);
map.put(Attr_AcmeIngressNetworkInterfaceId.NAME, Attr_AcmeIngressNetworkInterfaceId.class);
map.put(Attr_AcmeIngressVlanTagValue.NAME, Attr_AcmeIngressVlanTagValue.class);
map.put(Attr_AcmeEgressNetworkInterfaceId.NAME, Attr_AcmeEgressNetworkInterfaceId.class);
map.put(Attr_AcmeEgressVlanTagValue.NAME, Attr_AcmeEgressVlanTagValue.class);
map.put(Attr_AcmeReferCallTransferId.NAME, Attr_AcmeReferCallTransferId.class);
map.put(Attr_AcmeFlowMediaTypeFS1F.NAME, Attr_AcmeFlowMediaTypeFS1F.class);
map.put(Attr_AcmeFlowMediaTypeFS1R.NAME, Attr_AcmeFlowMediaTypeFS1R.class);
map.put(Attr_AcmeFlowMediaTypeFS2F.NAME, Attr_AcmeFlowMediaTypeFS2F.class);
map.put(Attr_AcmeFlowMediaTypeFS2R.NAME, Attr_AcmeFlowMediaTypeFS2R.class);
map.put(Attr_AcmeFlowPTimeFS1F.NAME, Attr_AcmeFlowPTimeFS1F.class);
map.put(Attr_AcmeFlowPTimeFS1R.NAME, Attr_AcmeFlowPTimeFS1R.class);
map.put(Attr_AcmeFlowPTimeFS2F.NAME, Attr_AcmeFlowPTimeFS2F.class);
map.put(Attr_AcmeFlowPTimeFS2R.NAME, Attr_AcmeFlowPTimeFS2R.class);
map.put(Attr_AcmeSessionMediaProcess.NAME, Attr_AcmeSessionMediaProcess.class);
map.put(Attr_AcmeCallingRFactor.NAME, Attr_AcmeCallingRFactor.class);
map.put(Attr_AcmeCallingMOS.NAME, Attr_AcmeCallingMOS.class);
map.put(Attr_AcmeCalledRFactor.NAME, Attr_AcmeCalledRFactor.class);
map.put(Attr_AcmeCalledMOS.NAME, Attr_AcmeCalledMOS.class);
map.put(Attr_AcmeFlowInSrcIPv6AddrFS1F.NAME, Attr_AcmeFlowInSrcIPv6AddrFS1F.class);
map.put(Attr_AcmeFlowInDstIPv6AddrFS1F.NAME, Attr_AcmeFlowInDstIPv6AddrFS1F.class);
map.put(Attr_AcmeFlowOutSrcIPv6AddrFS1F.NAME, Attr_AcmeFlowOutSrcIPv6AddrFS1F.class);
map.put(Attr_AcmeFlowOutDstIPv6AddrFS1F.NAME, Attr_AcmeFlowOutDstIPv6AddrFS1F.class);
map.put(Attr_AcmeFlowInSrcIPv6AddrFS1R.NAME, Attr_AcmeFlowInSrcIPv6AddrFS1R.class);
map.put(Attr_AcmeFlowInDstIPv6AddrFS1R.NAME, Attr_AcmeFlowInDstIPv6AddrFS1R.class);
map.put(Attr_AcmeFlowOutSrcIPv6AddrFS1R.NAME, Attr_AcmeFlowOutSrcIPv6AddrFS1R.class);
map.put(Attr_AcmeFlowOutDstIPv6AddrFS1R.NAME, Attr_AcmeFlowOutDstIPv6AddrFS1R.class);
map.put(Attr_AcmeFlowInSrcIPv6AddrFS2F.NAME, Attr_AcmeFlowInSrcIPv6AddrFS2F.class);
map.put(Attr_AcmeFlowInDstIPv6AddrFS2F.NAME, Attr_AcmeFlowInDstIPv6AddrFS2F.class);
map.put(Attr_AcmeFlowOutSrcIPv6AddrFS2F.NAME, Attr_AcmeFlowOutSrcIPv6AddrFS2F.class);
map.put(Attr_AcmeFlowOutDstIPv6AddrFS2F.NAME, Attr_AcmeFlowOutDstIPv6AddrFS2F.class);
map.put(Attr_AcmeFlowInSrcIPv6AddrFS2R.NAME, Attr_AcmeFlowInSrcIPv6AddrFS2R.class);
map.put(Attr_AcmeFlowInDstIPv6AddrFS2R.NAME, Attr_AcmeFlowInDstIPv6AddrFS2R.class);
map.put(Attr_AcmeFlowOutSrcIPv6AddrFS2R.NAME, Attr_AcmeFlowOutSrcIPv6AddrFS2R.class);
map.put(Attr_AcmeFlowOutDstIPv6AddrFS2R.NAME, Attr_AcmeFlowOutDstIPv6AddrFS2R.class);
map.put(Attr_AcmeSessionForkedCallId.NAME, Attr_AcmeSessionForkedCallId.class);
map.put(Attr_AcmeCustomVSA200.NAME, Attr_AcmeCustomVSA200.class);
map.put(Attr_AcmeCustomVSA201.NAME, Attr_AcmeCustomVSA201.class);
map.put(Attr_AcmeCustomVSA202.NAME, Attr_AcmeCustomVSA202.class);
map.put(Attr_AcmeCustomVSA203.NAME, Attr_AcmeCustomVSA203.class);
map.put(Attr_AcmeCustomVSA204.NAME, Attr_AcmeCustomVSA204.class);
map.put(Attr_AcmeCustomVSA205.NAME, Attr_AcmeCustomVSA205.class);
map.put(Attr_AcmeCustomVSA206.NAME, Attr_AcmeCustomVSA206.class);
map.put(Attr_AcmeCustomVSA207.NAME, Attr_AcmeCustomVSA207.class);
map.put(Attr_AcmeCustomVSA208.NAME, Attr_AcmeCustomVSA208.class);
map.put(Attr_AcmeCustomVSA209.NAME, Attr_AcmeCustomVSA209.class);
map.put(Attr_AcmeCustomVSA210.NAME, Attr_AcmeCustomVSA210.class);
map.put(Attr_AcmeCustomVSA211.NAME, Attr_AcmeCustomVSA211.class);
map.put(Attr_AcmeCustomVSA212.NAME, Attr_AcmeCustomVSA212.class);
map.put(Attr_AcmeCustomVSA213.NAME, Attr_AcmeCustomVSA213.class);
map.put(Attr_AcmeCustomVSA214.NAME, Attr_AcmeCustomVSA214.class);
map.put(Attr_AcmeCustomVSA215.NAME, Attr_AcmeCustomVSA215.class);
map.put(Attr_AcmeCustomVSA216.NAME, Attr_AcmeCustomVSA216.class);
map.put(Attr_AcmeCustomVSA217.NAME, Attr_AcmeCustomVSA217.class);
map.put(Attr_AcmeCustomVSA218.NAME, Attr_AcmeCustomVSA218.class);
map.put(Attr_AcmeCustomVSA219.NAME, Attr_AcmeCustomVSA219.class);
map.put(Attr_AcmeCustomVSA220.NAME, Attr_AcmeCustomVSA220.class);
map.put(Attr_AcmeCustomVSA221.NAME, Attr_AcmeCustomVSA221.class);
map.put(Attr_AcmeCustomVSA222.NAME, Attr_AcmeCustomVSA222.class);
map.put(Attr_AcmeCustomVSA223.NAME, Attr_AcmeCustomVSA223.class);
map.put(Attr_AcmeCustomVSA224.NAME, Attr_AcmeCustomVSA224.class);
map.put(Attr_AcmeCustomVSA225.NAME, Attr_AcmeCustomVSA225.class);
map.put(Attr_AcmeCustomVSA226.NAME, Attr_AcmeCustomVSA226.class);
map.put(Attr_AcmeCustomVSA227.NAME, Attr_AcmeCustomVSA227.class);
map.put(Attr_AcmeCustomVSA228.NAME, Attr_AcmeCustomVSA228.class);
map.put(Attr_AcmeCustomVSA229.NAME, Attr_AcmeCustomVSA229.class);
map.put(Attr_AcmeCustomVSA230.NAME, Attr_AcmeCustomVSA230.class);
map.put(Attr_AcmeUserClass.NAME, Attr_AcmeUserClass.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy