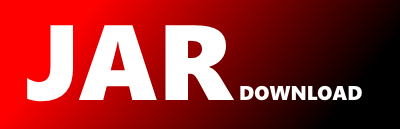
net.jradius.dictionary.vsa_starent.Attr_SNDisconnectReason Maven / Gradle / Ivy
The newest version!
// DO NOT EDIT THIS FILE DIRECTLY! - AUTOMATICALLY GENERATED
// Generated by: class net.jradius.freeradius.RadiusDictionary
// Generated on: Thu, 5 Jan 2017 15:56:13 +0200
package net.jradius.dictionary.vsa_starent;
import java.io.Serializable;
import java.util.LinkedHashMap;
import java.util.Map;
import net.jradius.packet.attribute.VSAttribute;
import net.jradius.packet.attribute.value.NamedValue;
/**
* Attribute Name: SN-Disconnect-Reason
* Attribute Type: 26
* Vendor Id: 8164
* VSA Type: 3
* Value Type: NamedValue
* Possible Values:
*
* - Not-Defined (0)
*
- Admin-Disconnect (1)
*
- Remote-Disconnect (2)
*
- Local-Disconnect (3)
*
- Disc-No-Resource (4)
*
- Disc-Excd-Service-Limit (5)
*
- PPP-LCP-Neg-Failed (6)
*
- PPP-LCP-No-Response (7)
*
- PPP-LCP-Loopback (8)
*
- PPP-LCP-Max-Retry (9)
*
- PPP-Echo-Failed (10)
*
- PPP-Auth-Failed (11)
*
- PPP-Auth-Failed-No-AAA-Resp (12)
*
- PPP-Auth-No-Response (13)
*
- PPP-Auth-Max-Retry (14)
*
- Invalid-AAA-Attr (15)
*
- Failed-User-Filter (16)
*
- Failed-Provide-Service (17)
*
- Invalid-IP-Address-AAA (18)
*
- Invalid-IP-Pool-AAA (19)
*
- PPP-IPCP-Neg-Failed (20)
*
- PPP-IPCP-No-Response (21)
*
- PPP-IPCP-Max-Retry (22)
*
- PPP-No-Rem-IP-Address (23)
*
- Inactivity-Timeout (24)
*
- Session-Timeout (25)
*
- Max-Data-Excd (26)
*
- Invalid-IP-Source-Address (27)
*
- MSID-Auth-Failed (28)
*
- MSID-Auth-Fauiled-No-AAA-Resp (29)
*
- A11-Max-Retry (30)
*
- A11-Lifetime-Expired (31)
*
- A11-Message-Integrity-Failure (32)
*
- PPP-lcp-remote-disc (33)
*
- Session-setup-timeout (34)
*
- PPP-keepalive-failure (35)
*
- Flow-add-failed (36)
*
- Call-type-detection-failed (37)
*
- Wrong-ipcp-params (38)
*
- MIP-remote-dereg (39)
*
- MIP-lifetime-expiry (40)
*
- MIP-proto-error (41)
*
- MIP-auth-failure (42)
*
- MIP-reg-timeout (43)
*
- Invalid-dest-context (44)
*
- Source-context-removed (45)
*
- Destination-context-removed (46)
*
- Req-service-addr-unavailable (47)
*
- Demux-mgr-failed (48)
*
- Internal-error (49)
*
*
* @author class net.jradius.freeradius.RadiusDictionary
*/
public final class Attr_SNDisconnectReason extends VSAttribute
{
public static final String NAME = "SN-Disconnect-Reason";
public static final int VENDOR_ID = 8164;
public static final int VSA_TYPE = 3;
public static final long TYPE = ((VENDOR_ID & 0xFFFF) << 16) | VSA_TYPE;
public static final long serialVersionUID = TYPE;
public static final Long NotDefined = new Long(0L);
public static final Long AdminDisconnect = new Long(1L);
public static final Long RemoteDisconnect = new Long(2L);
public static final Long LocalDisconnect = new Long(3L);
public static final Long DiscNoResource = new Long(4L);
public static final Long DiscExcdServiceLimit = new Long(5L);
public static final Long PPPLCPNegFailed = new Long(6L);
public static final Long PPPLCPNoResponse = new Long(7L);
public static final Long PPPLCPLoopback = new Long(8L);
public static final Long PPPLCPMaxRetry = new Long(9L);
public static final Long PPPEchoFailed = new Long(10L);
public static final Long PPPAuthFailed = new Long(11L);
public static final Long PPPAuthFailedNoAAAResp = new Long(12L);
public static final Long PPPAuthNoResponse = new Long(13L);
public static final Long PPPAuthMaxRetry = new Long(14L);
public static final Long InvalidAAAAttr = new Long(15L);
public static final Long FailedUserFilter = new Long(16L);
public static final Long FailedProvideService = new Long(17L);
public static final Long InvalidIPAddressAAA = new Long(18L);
public static final Long InvalidIPPoolAAA = new Long(19L);
public static final Long PPPIPCPNegFailed = new Long(20L);
public static final Long PPPIPCPNoResponse = new Long(21L);
public static final Long PPPIPCPMaxRetry = new Long(22L);
public static final Long PPPNoRemIPAddress = new Long(23L);
public static final Long InactivityTimeout = new Long(24L);
public static final Long SessionTimeout = new Long(25L);
public static final Long MaxDataExcd = new Long(26L);
public static final Long InvalidIPSourceAddress = new Long(27L);
public static final Long MSIDAuthFailed = new Long(28L);
public static final Long MSIDAuthFauiledNoAAAResp = new Long(29L);
public static final Long A11MaxRetry = new Long(30L);
public static final Long A11LifetimeExpired = new Long(31L);
public static final Long A11MessageIntegrityFailure = new Long(32L);
public static final Long PPPlcpremotedisc = new Long(33L);
public static final Long Sessionsetuptimeout = new Long(34L);
public static final Long PPPkeepalivefailure = new Long(35L);
public static final Long Flowaddfailed = new Long(36L);
public static final Long Calltypedetectionfailed = new Long(37L);
public static final Long Wrongipcpparams = new Long(38L);
public static final Long MIPremotedereg = new Long(39L);
public static final Long MIPlifetimeexpiry = new Long(40L);
public static final Long MIPprotoerror = new Long(41L);
public static final Long MIPauthfailure = new Long(42L);
public static final Long MIPregtimeout = new Long(43L);
public static final Long Invaliddestcontext = new Long(44L);
public static final Long Sourcecontextremoved = new Long(45L);
public static final Long Destinationcontextremoved = new Long(46L);
public static final Long Reqserviceaddrunavailable = new Long(47L);
public static final Long Demuxmgrfailed = new Long(48L);
public static final Long Internalerror = new Long(49L);
@SuppressWarnings("serial")
protected class NamedValueMap implements NamedValue.NamedValueMap, Serializable
{
public Long[] knownValues = { new Long(0L),new Long(1L),new Long(2L),new Long(3L),new Long(4L),new Long(5L),new Long(6L),new Long(7L),new Long(8L),new Long(9L),new Long(10L),new Long(11L),new Long(12L),new Long(13L),new Long(14L),new Long(15L),new Long(16L),new Long(17L),new Long(18L),new Long(19L),new Long(20L),new Long(21L),new Long(22L),new Long(23L),new Long(24L),new Long(25L),new Long(26L),new Long(27L),new Long(28L),new Long(29L),new Long(30L),new Long(31L),new Long(32L),new Long(33L),new Long(34L),new Long(35L),new Long(36L),new Long(37L),new Long(38L),new Long(39L),new Long(40L),new Long(41L),new Long(42L),new Long(43L),new Long(44L),new Long(45L),new Long(46L),new Long(47L),new Long(48L),new Long(49L)};
public Long[] getKnownValues() { return knownValues; }
public Long getNamedValue(String name)
{
if ("Not-Defined".equals(name)) return new Long(0L);
if ("Admin-Disconnect".equals(name)) return new Long(1L);
if ("Remote-Disconnect".equals(name)) return new Long(2L);
if ("Local-Disconnect".equals(name)) return new Long(3L);
if ("Disc-No-Resource".equals(name)) return new Long(4L);
if ("Disc-Excd-Service-Limit".equals(name)) return new Long(5L);
if ("PPP-LCP-Neg-Failed".equals(name)) return new Long(6L);
if ("PPP-LCP-No-Response".equals(name)) return new Long(7L);
if ("PPP-LCP-Loopback".equals(name)) return new Long(8L);
if ("PPP-LCP-Max-Retry".equals(name)) return new Long(9L);
if ("PPP-Echo-Failed".equals(name)) return new Long(10L);
if ("PPP-Auth-Failed".equals(name)) return new Long(11L);
if ("PPP-Auth-Failed-No-AAA-Resp".equals(name)) return new Long(12L);
if ("PPP-Auth-No-Response".equals(name)) return new Long(13L);
if ("PPP-Auth-Max-Retry".equals(name)) return new Long(14L);
if ("Invalid-AAA-Attr".equals(name)) return new Long(15L);
if ("Failed-User-Filter".equals(name)) return new Long(16L);
if ("Failed-Provide-Service".equals(name)) return new Long(17L);
if ("Invalid-IP-Address-AAA".equals(name)) return new Long(18L);
if ("Invalid-IP-Pool-AAA".equals(name)) return new Long(19L);
if ("PPP-IPCP-Neg-Failed".equals(name)) return new Long(20L);
if ("PPP-IPCP-No-Response".equals(name)) return new Long(21L);
if ("PPP-IPCP-Max-Retry".equals(name)) return new Long(22L);
if ("PPP-No-Rem-IP-Address".equals(name)) return new Long(23L);
if ("Inactivity-Timeout".equals(name)) return new Long(24L);
if ("Session-Timeout".equals(name)) return new Long(25L);
if ("Max-Data-Excd".equals(name)) return new Long(26L);
if ("Invalid-IP-Source-Address".equals(name)) return new Long(27L);
if ("MSID-Auth-Failed".equals(name)) return new Long(28L);
if ("MSID-Auth-Fauiled-No-AAA-Resp".equals(name)) return new Long(29L);
if ("A11-Max-Retry".equals(name)) return new Long(30L);
if ("A11-Lifetime-Expired".equals(name)) return new Long(31L);
if ("A11-Message-Integrity-Failure".equals(name)) return new Long(32L);
if ("PPP-lcp-remote-disc".equals(name)) return new Long(33L);
if ("Session-setup-timeout".equals(name)) return new Long(34L);
if ("PPP-keepalive-failure".equals(name)) return new Long(35L);
if ("Flow-add-failed".equals(name)) return new Long(36L);
if ("Call-type-detection-failed".equals(name)) return new Long(37L);
if ("Wrong-ipcp-params".equals(name)) return new Long(38L);
if ("MIP-remote-dereg".equals(name)) return new Long(39L);
if ("MIP-lifetime-expiry".equals(name)) return new Long(40L);
if ("MIP-proto-error".equals(name)) return new Long(41L);
if ("MIP-auth-failure".equals(name)) return new Long(42L);
if ("MIP-reg-timeout".equals(name)) return new Long(43L);
if ("Invalid-dest-context".equals(name)) return new Long(44L);
if ("Source-context-removed".equals(name)) return new Long(45L);
if ("Destination-context-removed".equals(name)) return new Long(46L);
if ("Req-service-addr-unavailable".equals(name)) return new Long(47L);
if ("Demux-mgr-failed".equals(name)) return new Long(48L);
if ("Internal-error".equals(name)) return new Long(49L);
return null;
}
public String getNamedValue(Long value)
{
if (new Long(0L).equals(value)) return "Not-Defined";
if (new Long(1L).equals(value)) return "Admin-Disconnect";
if (new Long(2L).equals(value)) return "Remote-Disconnect";
if (new Long(3L).equals(value)) return "Local-Disconnect";
if (new Long(4L).equals(value)) return "Disc-No-Resource";
if (new Long(5L).equals(value)) return "Disc-Excd-Service-Limit";
if (new Long(6L).equals(value)) return "PPP-LCP-Neg-Failed";
if (new Long(7L).equals(value)) return "PPP-LCP-No-Response";
if (new Long(8L).equals(value)) return "PPP-LCP-Loopback";
if (new Long(9L).equals(value)) return "PPP-LCP-Max-Retry";
if (new Long(10L).equals(value)) return "PPP-Echo-Failed";
if (new Long(11L).equals(value)) return "PPP-Auth-Failed";
if (new Long(12L).equals(value)) return "PPP-Auth-Failed-No-AAA-Resp";
if (new Long(13L).equals(value)) return "PPP-Auth-No-Response";
if (new Long(14L).equals(value)) return "PPP-Auth-Max-Retry";
if (new Long(15L).equals(value)) return "Invalid-AAA-Attr";
if (new Long(16L).equals(value)) return "Failed-User-Filter";
if (new Long(17L).equals(value)) return "Failed-Provide-Service";
if (new Long(18L).equals(value)) return "Invalid-IP-Address-AAA";
if (new Long(19L).equals(value)) return "Invalid-IP-Pool-AAA";
if (new Long(20L).equals(value)) return "PPP-IPCP-Neg-Failed";
if (new Long(21L).equals(value)) return "PPP-IPCP-No-Response";
if (new Long(22L).equals(value)) return "PPP-IPCP-Max-Retry";
if (new Long(23L).equals(value)) return "PPP-No-Rem-IP-Address";
if (new Long(24L).equals(value)) return "Inactivity-Timeout";
if (new Long(25L).equals(value)) return "Session-Timeout";
if (new Long(26L).equals(value)) return "Max-Data-Excd";
if (new Long(27L).equals(value)) return "Invalid-IP-Source-Address";
if (new Long(28L).equals(value)) return "MSID-Auth-Failed";
if (new Long(29L).equals(value)) return "MSID-Auth-Fauiled-No-AAA-Resp";
if (new Long(30L).equals(value)) return "A11-Max-Retry";
if (new Long(31L).equals(value)) return "A11-Lifetime-Expired";
if (new Long(32L).equals(value)) return "A11-Message-Integrity-Failure";
if (new Long(33L).equals(value)) return "PPP-lcp-remote-disc";
if (new Long(34L).equals(value)) return "Session-setup-timeout";
if (new Long(35L).equals(value)) return "PPP-keepalive-failure";
if (new Long(36L).equals(value)) return "Flow-add-failed";
if (new Long(37L).equals(value)) return "Call-type-detection-failed";
if (new Long(38L).equals(value)) return "Wrong-ipcp-params";
if (new Long(39L).equals(value)) return "MIP-remote-dereg";
if (new Long(40L).equals(value)) return "MIP-lifetime-expiry";
if (new Long(41L).equals(value)) return "MIP-proto-error";
if (new Long(42L).equals(value)) return "MIP-auth-failure";
if (new Long(43L).equals(value)) return "MIP-reg-timeout";
if (new Long(44L).equals(value)) return "Invalid-dest-context";
if (new Long(45L).equals(value)) return "Source-context-removed";
if (new Long(46L).equals(value)) return "Destination-context-removed";
if (new Long(47L).equals(value)) return "Req-service-addr-unavailable";
if (new Long(48L).equals(value)) return "Demux-mgr-failed";
if (new Long(49L).equals(value)) return "Internal-error";
return null;
}
};
public static transient NamedValueMap map = null;
public void setup()
{
attributeName = NAME;
attributeType = 26;
vendorId = VENDOR_ID;
vsaAttributeType = VSA_TYPE;
setFormat("2,2");
attributeValue = new NamedValue(map != null ? map : (map = new NamedValueMap()));
}
public Attr_SNDisconnectReason()
{
setup();
}
public Attr_SNDisconnectReason(Serializable o)
{
setup(o);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy