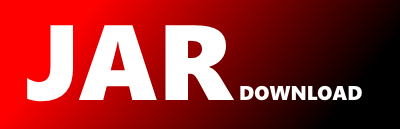
net.nicoulaj.maven.plugins.checksum.mojo.ArtifactsMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-checksum-plugin Show documentation
Show all versions of maven-checksum-plugin Show documentation
Compute project artifacts/dependencies/files checksum digests and output them to individual or summary files.
/*
* Copyright 2010 Julien Nicoulaud
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.nicoulaj.maven.plugins.checksum.mojo;
import net.nicoulaj.maven.plugins.checksum.Constants;
import net.nicoulaj.maven.plugins.checksum.execution.Execution;
import net.nicoulaj.maven.plugins.checksum.execution.ExecutionException;
import net.nicoulaj.maven.plugins.checksum.execution.FailOnErrorExecution;
import net.nicoulaj.maven.plugins.checksum.execution.NeverFailExecution;
import net.nicoulaj.maven.plugins.checksum.execution.target.CsvSummaryFileTarget;
import net.nicoulaj.maven.plugins.checksum.execution.target.MavenLogTarget;
import net.nicoulaj.maven.plugins.checksum.execution.target.OneHashPerFileTarget;
import net.nicoulaj.maven.plugins.checksum.execution.target.XmlSummaryFileTarget;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import org.codehaus.plexus.util.FileUtils;
import java.io.File;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.List;
/**
* Compute project artifacts checksum digests and store them in individual files and/or a summary file.
*
* @author Julien Nicoulaud
* @goal artifacts
* @phase verify
* @requiresProject true
* @inheritByDefault false
* @since 1.0
*/
public class ArtifactsMojo extends AbstractMojo
{
/**
* The Maven project.
*
* @parameter expression="${project}"
* @required
* @readonly
* @since 1.0
*/
protected MavenProject project;
/**
* The list of checksum algorithms used.
*
* Default value is MD5 and SHA-1.
Allowed values are CRC32, MD2, MD4, MD5, SHA-1, SHA-224, SHA-256, SHA-384,
* SHA-512, RIPEMD128, RIPEMD160, RIPEMD256, RIPEMD320, GOST3411 and Tiger.
*
* Use the following syntax:
*
<algorithms>
* <algorithm>MD5<algorithm>
* <algorithm>SHA-1<algorithm>
* </algorithms>
*
*
* @parameter
* @since 1.0
*/
protected List algorithms = Arrays.asList( Constants.DEFAULT_EXECUTION_ALGORITHMS );
/**
* Indicates whether the build will fail if there are errors.
*
* @parameter default-value="true"
* @since 1.0
*/
protected boolean failOnError;
/**
* Encoding to use for generated files.
*
* @parameter expression="${encoding}" default-value="${project.build.sourceEncoding}"
*/
protected String encoding = Constants.DEFAULT_ENCODING;
/**
* Indicates whether the build will store checksums in separate files (one file per algorithm per artifact).
*
* @parameter default-value="true"
* @since 1.0
*/
protected boolean individualFiles;
/**
* Indicates whether the build will print checksums in the build log.
*
* @parameter default-value="false"
* @since 1.0
*/
protected boolean quiet;
/**
* Indicates whether the build will store checksums to a single CSV summary file.
*
* @parameter default-value="false"
* @since 1.0
*/
protected boolean csvSummary;
/**
* The name of the summary file created if the option is activated.
*
* @parameter default-value="artifacts-checksums.csv"
* @see #csvSummary
* @since 1.0
*/
protected String csvSummaryFile;
/**
* Indicates whether the build will store checksums to a single XML summary file.
*
* @parameter default-value="false"
* @since 1.0
*/
protected boolean xmlSummary;
/**
* The name of the summary file created if the option is activated.
*
* @parameter default-value="artifacts-checksums.xml"
* @see #xmlSummary
* @since 1.0
*/
protected String xmlSummaryFile;
/**
* {@inheritDoc}
*/
public void execute() throws MojoExecutionException, MojoFailureException
{
// Prepare an execution.
Execution execution = ( failOnError ) ? new FailOnErrorExecution() : new NeverFailExecution( getLog() );
execution.setAlgorithms( algorithms );
execution.setFiles( getFilesToProcess() );
if ( !quiet )
{
execution.addTarget( new MavenLogTarget( getLog() ) );
}
if ( individualFiles )
{
execution.addTarget( new OneHashPerFileTarget( encoding ) );
}
if ( csvSummary )
{
execution.addTarget( new CsvSummaryFileTarget( FileUtils.resolveFile( new File( project.getBuild()
.getDirectory() ),
csvSummaryFile ),
encoding ) );
}
if ( xmlSummary )
{
execution.addTarget( new XmlSummaryFileTarget( FileUtils.resolveFile( new File( project.getBuild()
.getDirectory() ),
xmlSummaryFile ),
encoding ) );
}
// Run the execution.
try
{
execution.run();
}
catch ( ExecutionException e )
{
getLog().error( e.getMessage() );
throw new MojoFailureException( e.getMessage() );
}
}
/**
* Build the list of files from which digests should be generated.
*
* The list is composed of the project main and attached artifacts.
*
* @return the list of files that should be processed.
* @see #hasValidFile(org.apache.maven.artifact.Artifact)
*/
protected List getFilesToProcess()
{
List files = new LinkedList();
// Add project main artifact.
if ( hasValidFile( project.getArtifact() ) )
{
files.add( project.getArtifact().getFile() );
}
// Add projects attached.
if ( project.getAttachedArtifacts() != null )
{
for ( Artifact artifact : ( List ) project.getAttachedArtifacts() )
{
if ( hasValidFile( artifact ) )
{
files.add( artifact.getFile() );
}
}
}
return files;
}
/**
* Decide wether the artifact file should be processed.
*
* Excludes the project POM file and any file outside the build directory, because this could lead to writing
* files on the user local repository for example.
*
* @param artifact the artifact to check.
* @return true if the artifact should be included in the files to process.
*/
protected boolean hasValidFile( Artifact artifact )
{
// Make sure the file exists.
boolean hasValidFile = artifact != null && artifact.getFile() != null && artifact.getFile().exists();
// Exclude project POM file.
hasValidFile = hasValidFile && !artifact.getFile().getPath().equals( project.getFile().getPath() );
// Exclude files outside of build directory.
hasValidFile = hasValidFile && artifact.getFile().getPath().startsWith( project.getBuild().getDirectory() );
return hasValidFile;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy