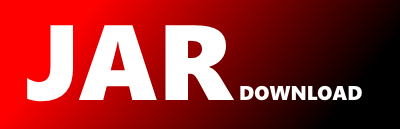
net.nicoulaj.maven.plugins.checksum.mojo.DependenciesMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-checksum-plugin Show documentation
Show all versions of maven-checksum-plugin Show documentation
Compute project artifacts/dependencies/files checksum digests and output them to individual or summary files.
/*
* Copyright 2010 Julien Nicoulaud
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.nicoulaj.maven.plugins.checksum.mojo;
import net.nicoulaj.maven.plugins.checksum.Constants;
import net.nicoulaj.maven.plugins.checksum.execution.Execution;
import net.nicoulaj.maven.plugins.checksum.execution.ExecutionException;
import net.nicoulaj.maven.plugins.checksum.execution.FailOnErrorExecution;
import net.nicoulaj.maven.plugins.checksum.execution.NeverFailExecution;
import net.nicoulaj.maven.plugins.checksum.execution.target.CsvSummaryFileTarget;
import net.nicoulaj.maven.plugins.checksum.execution.target.MavenLogTarget;
import net.nicoulaj.maven.plugins.checksum.execution.target.XmlSummaryFileTarget;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import org.codehaus.plexus.util.FileUtils;
import java.io.File;
import java.util.Arrays;
import java.util.LinkedList;
import java.util.List;
import java.util.Set;
/**
* Compute project dependencies checksum digests and store them in a summary file.
*
* @author Julien Nicoulaud
* @goal dependencies
* @phase verify
* @requiresProject true
* @inheritByDefault false
* @requiresDependencyResolution runtime
* @since 1.0
*/
public class DependenciesMojo extends AbstractMojo
{
/**
* The Maven project.
*
* @parameter expression="${project}"
* @required
* @readonly
* @since 1.0
*/
protected MavenProject project;
/**
* The list of checksum algorithms used.
*
* Default value is MD5 and SHA-1.
Allowed values are CRC32, MD2, MD4, MD5, SHA-1, SHA-224, SHA-256, SHA-384,
* SHA-512, RIPEMD128, RIPEMD160, RIPEMD256, RIPEMD320, GOST3411 and Tiger.
*
* Use the following syntax:
*
<algorithms>
* <algorithm>MD5<algorithm>
* <algorithm>SHA-1<algorithm>
* </algorithms>
*
*
* @parameter
* @since 1.0
*/
protected List algorithms = Arrays.asList( Constants.DEFAULT_EXECUTION_ALGORITHMS );
/**
* Indicates whether the build will fail if there are errors.
*
* @parameter default-value="true"
* @since 1.0
*/
protected boolean failOnError;
/**
* Encoding to use for generated files.
*
* @parameter expression="${encoding}" default-value="${project.build.sourceEncoding}"
*/
protected String encoding = Constants.DEFAULT_ENCODING;
/**
* Indicates whether the build will print checksums in the build log.
*
* @parameter default-value="false"
* @since 1.0
*/
protected boolean quiet;
/**
* Indicates whether the build will store checksums to a single CSV summary file.
*
* @parameter default-value="true"
* @since 1.0
*/
protected boolean csvSummary;
/**
* The name of the summary file created if the option is activated.
*
* @parameter default-value="dependencies-checksums.csv"
* @see #csvSummary
* @since 1.0
*/
protected String csvSummaryFile;
/**
* Indicates whether the build will store checksums to a single XML summary file.
*
* @parameter default-value="false"
* @since 1.0
*/
protected boolean xmlSummary;
/**
* The name of the summary file created if the option is activated.
*
* @parameter default-value="dependencies-checksums.xml"
* @see #xmlSummary
* @since 1.0
*/
protected String xmlSummaryFile;
/**
* The dependency scopes to include.
*
* Allowed values are compile, test, runtime, provided and system.
All scopes are included by default.
*
* Use the following syntax:
*
<scopes>
* <scope>compile<scope>
* <scope>runtime<scope>
* </scopes>
*
*
* @parameter
* @since 1.0
*/
protected List scopes;
/**
* The dependency types to include.
*
* All types are included by default.
*
* Use the following syntax:
*
<types>
* <type>jar<type>
* <type>zip<type>
* </types>
*
*
* @parameter
* @since 1.0
*/
protected List types;
/**
* {@inheritDoc}
*/
public void execute() throws MojoExecutionException, MojoFailureException
{
// Prepare an execution.
Execution execution = ( failOnError ) ? new FailOnErrorExecution() : new NeverFailExecution( getLog() );
execution.setAlgorithms( algorithms );
execution.setFiles( getFilesToProcess() );
if ( !quiet )
{
execution.addTarget( new MavenLogTarget( getLog() ) );
}
if ( csvSummary )
{
execution.addTarget( new CsvSummaryFileTarget( FileUtils.resolveFile( new File( project.getBuild()
.getDirectory() ),
csvSummaryFile ),
encoding ) );
}
if ( xmlSummary )
{
execution.addTarget( new XmlSummaryFileTarget( FileUtils.resolveFile( new File( project.getBuild()
.getDirectory() ),
xmlSummaryFile ),
encoding ) );
}
// Run the execution.
try
{
execution.run();
}
catch ( ExecutionException e )
{
getLog().error( e.getMessage() );
throw new MojoFailureException( e.getMessage() );
}
}
/**
* Build the list of files from which digests should be generated.
*
* The list is composed of the project dependencies.
*
* @return the list of files that should be processed.
*/
protected List getFilesToProcess()
{
List files = new LinkedList();
for ( Artifact artifact : ( Set ) project.getDependencyArtifacts() )
{
if ( ( scopes == null || scopes.contains( artifact.getScope() ) )
&& ( types == null || types.contains( artifact.getType() ) ) )
{
files.add( artifact.getFile() );
}
}
return files;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy