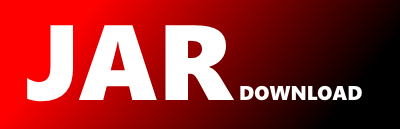
net.kaczmarzyk.spring.data.jpa.web.EnhancerUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of specification-arg-resolver Show documentation
Show all versions of specification-arg-resolver Show documentation
An alternative API for filtering data with Spring MVC and Spring Data JPA.
This library provides a custom HandlerMethodArgumentResolver that transforms HTTP parameters
into a Specification object ready to use with Spring Data repositories.
/**
* Copyright 2014-2022 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.kaczmarzyk.spring.data.jpa.web;
import org.springframework.cglib.proxy.Enhancer;
import org.springframework.cglib.proxy.MethodInterceptor;
import org.springframework.data.jpa.domain.Specification;
import java.lang.reflect.Field;
/**
* @author Tomasz Kaczmarzyk
*/
class EnhancerUtil {
@SuppressWarnings("unchecked")
static T wrapWithIfaceImplementation(final Class iface, final Specification
© 2015 - 2024 Weber Informatics LLC | Privacy Policy