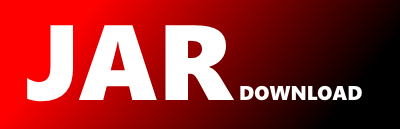
net.kaczmarzyk.spring.data.jpa.web.SpecificationArgumentResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of specification-arg-resolver Show documentation
Show all versions of specification-arg-resolver Show documentation
An alternative API for filtering data with Spring MVC and Spring Data JPA.
This library provides a custom HandlerMethodArgumentResolver that transforms HTTP parameters
into a Specification object ready to use with Spring Data repositories.
/**
* Copyright 2014-2020 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.kaczmarzyk.spring.data.jpa.web;
import net.kaczmarzyk.spring.data.jpa.utils.TypeUtil;
import org.springframework.core.MethodParameter;
import org.springframework.core.convert.ConversionService;
import org.springframework.data.jpa.domain.Specification;
import org.springframework.web.bind.support.WebDataBinderFactory;
import org.springframework.web.context.request.NativeWebRequest;
import org.springframework.web.method.support.HandlerMethodArgumentResolver;
import org.springframework.web.method.support.ModelAndViewContainer;
import java.lang.annotation.Annotation;
import java.util.*;
import java.util.function.Consumer;
import static java.util.Objects.nonNull;
import static java.util.function.Function.identity;
import static java.util.stream.Collectors.toMap;
/**
* @author Tomasz Kaczmarzyk
* @author Jakub Radlica
*/
public class SpecificationArgumentResolver implements HandlerMethodArgumentResolver {
private static Map, SpecificationResolver extends Annotation>> resolversBySupportedType;
public SpecificationArgumentResolver() {
this(null);
}
public SpecificationArgumentResolver(ConversionService conversionService) {
SimpleSpecificationResolver simpleSpecificationResolver = new SimpleSpecificationResolver(conversionService);
resolversBySupportedType = Arrays.asList(
simpleSpecificationResolver,
new OrSpecificationResolver(simpleSpecificationResolver),
new DisjunctionSpecificationResolver(simpleSpecificationResolver),
new ConjunctionSpecificationResolver(simpleSpecificationResolver),
new AndSpecificationResolver(simpleSpecificationResolver),
new JoinSpecificationResolver(),
new JoinsSpecificationResolver(),
new JoinFetchSpecificationResolver()).stream()
.collect(toMap(
SpecificationResolver::getSupportedSpecificationDefinition,
identity(),
(u,v) -> { throw new IllegalStateException(String.format("Duplicate key %s", u)); },
LinkedHashMap::new
));
}
@Override
public boolean supportsParameter(MethodParameter parameter) {
Class> paramType = parameter.getParameterType();
return paramType.isInterface() && Specification.class.isAssignableFrom(paramType) && isAnnotated(parameter);
}
@Override
public Object resolveArgument(MethodParameter parameter, ModelAndViewContainer mavContainer, NativeWebRequest webRequest,
WebDataBinderFactory binderFactory) throws Exception {
WebRequestProcessingContext context = new WebRequestProcessingContext(parameter, webRequest);
List> specs = resolveSpec(context);
if (specs.isEmpty()) {
return null;
}
if (specs.size() == 1) {
Specification
© 2015 - 2024 Weber Informatics LLC | Privacy Policy