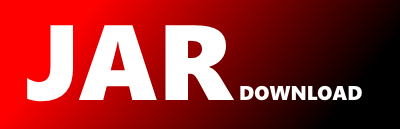
net.kemitix.mon.Mon Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mon Show documentation
Show all versions of mon Show documentation
TypeAlias, Result and Maybe for Java
/**
* The MIT License (MIT)
*
* Copyright (c) 2017 Paul Campbell
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software
* and associated documentation files (the "Software"), to deal in the Software without restriction,
* including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense,
* and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so,
* subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all copies
* or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
* INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE
* AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package net.kemitix.mon;
import lombok.AccessLevel;
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
* An almost-monad type-alias.
*
* @param the type of the alias
*
* @author Paul Campbell ([email protected])
*/
@RequiredArgsConstructor(access = AccessLevel.PROTECTED)
public class Mon implements Functor> {
/**
* The value.
*/
private final T value;
/**
* Create a factory for creating validated instances.
*
* The value can never be null.
*
* @param validator the validator function
* @param onValid the function to create when valid
* @param onInvalid the function to create when invalid
* @param the type of the value
* @param the type of the factory output
*
* @return a function to apply to values to alias
*/
public static Function factory(
@NonNull final Predicate validator,
@NonNull final Function, R> onValid,
@NonNull final Supplier onInvalid
) {
return v -> {
if (v != null && validator.test(v)) {
return onValid.apply(Mon.of(v));
}
return onInvalid.get();
};
}
/**
* Create a new Mon for the value.
*
* @param v the value
* @param the type of the value
*
* @return a Mon containing the value
*/
public static Mon of(@NonNull final T v) {
return new Mon<>(v);
}
@Override
public final Mon map(final Function f) {
return Mon.of(f.apply(value));
}
/**
* Returns a Mon consisting of the results of replacing the content of this Mon with the contents of a mapped Mon
* produced by applying the provided mapping function to the content of the Mon.
*
* @param f the mapping function the produces a Mon
* @param the type of the result of the mapping function
*
* @return a Mon containing the result of the function
*/
public final Mon flatMap(final Function> f) {
return f.apply(value);
}
/**
* The hashcode.
*
* @return the hashcode
*/
@Override
public int hashCode() {
return value.hashCode();
}
/**
* equals.
*
* @param o the object to compare to
*
* @return true if they are the same
*/
@Override
public boolean equals(final Object o) {
return this == o || o != null && getClass() == o.getClass() && value.equals(((Mon>) o).value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy