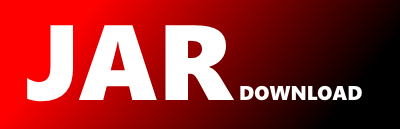
net.kemitix.mon.experimental.either.Either Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mon Show documentation
Show all versions of mon Show documentation
TypeAlias, Result and Maybe for Java
The newest version!
/**
* The MIT License (MIT)
*
* Copyright (c) 2021 Paul Campbell
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software
* and associated documentation files (the "Software"), to deal in the Software without restriction,
* including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense,
* and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so,
* subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all copies
* or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
* INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE
* AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package net.kemitix.mon.experimental.either;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* An Either type for holding a one of two possible values, a left and a right, that may be of different types.
*
* @param the type of the Either for left value
* @param the type of the Either for right value
* @author Paul Campbell ([email protected])
*/
@SuppressWarnings("methodcount")
public interface Either {
/**
* Create a new Either holding a left value.
*
* @param l the left value
* @param the type of the left value
* @param the type of the right value
* @return a Either holding the left value
*/
static Either left(final L l) {
return new Left<>(l);
}
/**
* Create a new Either holding a right value.
*
* @param r the right value
* @param the type of the left value
* @param the type of the right value
* @return a Either holding the right value
*/
static Either right(final R r) {
return new Right<>(r);
}
/**
* Checks if the Either holds a left value.
*
* @return true if this Either is a left
*/
boolean isLeft();
/**
* Checks if the Either holds a right value.
*
* @return true if this Either is a right
*/
boolean isRight();
/**
* Matches the Either, invoking the correct Consumer.
*
* @param onLeft the Consumer to invoke when the Either is a left
* @param onRight the Consumer to invoke when the Either is a right
*/
void match(Consumer onLeft, Consumer onRight);
/**
* Map the function across the left value.
*
* @param f the function to apply to any left value
* @param the type to change the left value to
* @return a new Either
*/
Either mapLeft(Function f);
/**
* Map the function across the right value.
*
* @param f the function to apply to any right value
* @param the type to change the right value to
* @return a new Either
*/
Either mapRight(Function f);
/**
* FlatMap the function across the left value.
*
* @param f the function to apply to any left value
* @param the type to change the left value to
* @return a new Either if is a Left, else this
*/
Either flatMapLeft(Function> f);
/**
* FlatMap the function across the right value.
*
* @param f the function to apply to any right value
* @param the type to change the right value to
* @return a new Either if is a Right, else this
*/
Either flatMapRight(Function> f);
/**
* Returns an Optional containing the left value, if is a left, otherwise
* returns an empty Optional.
*
* @return An Optional containing any left value
*/
Optional getLeft();
/**
* Returns an Optional containing the right value, if is a right, otherwise
* returns an empty Optional.
*
* @return An Optional containing any right value
*/
Optional getRight();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy