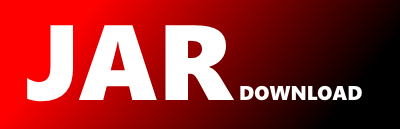
net.kemitix.node.Nodes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of node Show documentation
Show all versions of node Show documentation
A parent/children data structure
package net.kemitix.node;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Utility class for {@link Node} items.
*
* @author pcampbell
*/
public final class Nodes {
private Nodes() {
}
/**
* Creates a new unnamed root node.
*
* @param data the data the node will contain
* @param the type of the data
*
* @return the new node
*/
public static Node unnamedRoot(final T data) {
return new NodeItem<>(data);
}
/**
* Creates a new named root node.
*
* @param data the data the node will contain
* @param name the name of the node
* @param the type of the data
*
* @return the new node
*/
public static Node namedRoot(final T data, final String name) {
return new NodeItem<>(data, name);
}
/**
* Creates a new unnamed child node.
*
* @param data the data the node will contain
* @param parent the parent of the node
* @param the type of the data
*
* @return the new node
*/
public static Node unnamedChild(final T data, final Node parent) {
return new NodeItem<>(data, parent);
}
/**
* Creates a new named child node.
*
* @param data the data the node will contain
* @param name the name of the node
* @param parent the parent of the node
* @param the type of the data
*
* @return the new node
*/
public static Node namedChild(
final T data, final String name, final Node parent) {
return new NodeItem<>(data, name, parent);
}
/**
* Creates an immutable copy of an existing node tree.
*
* @param root the root node of the source tree
* @param the type of the data
*
* @return the immutable copy of the tree
*/
public static Node asImmutable(final Node root) {
if (root.getParent().isPresent()) {
throw new IllegalArgumentException("source must be the root node");
}
final Set> children = getImmutableChildren(root);
return ImmutableNodeItem.newRoot(root.getData().orElse(null),
root.getName(), children);
}
private static Set> getImmutableChildren(final Node source) {
return source.getChildren()
.stream()
.map(Nodes::asImmutableChild)
.collect(Collectors.toSet());
}
private static Node asImmutableChild(
final Node source) {
final Optional> sourceParent = source.getParent();
if (sourceParent.isPresent()) {
return ImmutableNodeItem.newChild(source.getData().orElse(null),
source.getName(), sourceParent.get(),
getImmutableChildren(source));
} else {
throw new IllegalArgumentException(
"source must not be the root node");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy