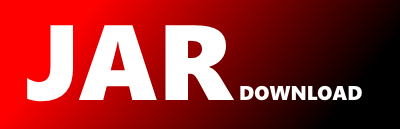
kieker.analysis.generic.sink.graph.graphml.GraphMLTransformer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kieker Show documentation
Show all versions of kieker Show documentation
Kieker: Application Performance Monitoring and Dynamic Software Analysis
/***************************************************************************
* Copyright 2022 Kieker Project (http://kieker-monitoring.net)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
***************************************************************************/
package kieker.analysis.generic.sink.graph.graphml;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.graphdrawing.graphml.DataType;
import org.graphdrawing.graphml.EdgeType;
import org.graphdrawing.graphml.GraphEdgedefaultType;
import org.graphdrawing.graphml.GraphType;
import org.graphdrawing.graphml.GraphmlType;
import org.graphdrawing.graphml.KeyForType;
import org.graphdrawing.graphml.KeyType;
import org.graphdrawing.graphml.KeyTypeType;
import org.graphdrawing.graphml.NodeType;
import com.google.common.graph.EndpointPair;
import com.google.common.graph.MutableNetwork;
import kieker.analysis.generic.graph.IEdge;
import kieker.analysis.generic.graph.IGraph;
import kieker.analysis.generic.graph.INode;
import kieker.analysis.generic.sink.graph.AbstractTransformer;
/**
* @param
* node type
* @param
* edge type
*
* @author Sören Henning
*
* @since 1.14
*/
public class GraphMLTransformer extends AbstractTransformer {
private final GraphType graphType;
private final Set nodeKeys = new HashSet<>();
private final Set edgeKeys = new HashSet<>();
public GraphMLTransformer(final IGraph graph) {
super(graph);
this.graphType = new GraphType();
this.graphType.setEdgedefault(GraphEdgedefaultType.DIRECTED);
this.graphType.setId(graph.getLabel());
}
public GraphMLTransformer(final MutableNetwork graph, final String label) {
super(graph, label);
this.graphType = new GraphType();
this.graphType.setEdgedefault(GraphEdgedefaultType.DIRECTED);
this.graphType.setId(label);
}
@Override
protected void transformVertex(final INode vertex) {
final NodeType nodeType = new NodeType();
nodeType.setId(vertex.getId().toString());
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy