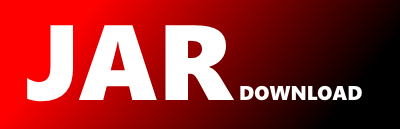
kieker.model.analysismodel.assembly.util.AssemblySwitch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kieker Show documentation
Show all versions of kieker Show documentation
Kieker: Application Performance Monitoring and Dynamic Software Analysis
/**
*/
package kieker.model.analysismodel.assembly.util;
import java.util.Map;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.util.Switch;
import kieker.model.analysismodel.assembly.AssemblyComponent;
import kieker.model.analysismodel.assembly.AssemblyModel;
import kieker.model.analysismodel.assembly.AssemblyOperation;
import kieker.model.analysismodel.assembly.AssemblyPackage;
import kieker.model.analysismodel.assembly.AssemblyProvidedInterface;
import kieker.model.analysismodel.assembly.AssemblyRequiredInterface;
import kieker.model.analysismodel.assembly.AssemblyStorage;
/**
*
* The Switch for the model's inheritance hierarchy.
* It supports the call {@link #doSwitch(EObject) doSwitch(object)}
* to invoke the caseXXX
method for each class of the model,
* starting with the actual class of the object
* and proceeding up the inheritance hierarchy
* until a non-null result is returned,
* which is the result of the switch.
*
*
* @see kieker.model.analysismodel.assembly.AssemblyPackage
* @generated
*/
public class AssemblySwitch extends Switch {
/**
* The cached model package
*
*
*
* @generated
*/
protected static AssemblyPackage modelPackage;
/**
* Creates an instance of the switch.
*
*
*
* @generated
*/
public AssemblySwitch() {
if (modelPackage == null) {
modelPackage = AssemblyPackage.eINSTANCE;
}
}
/**
* Checks whether this is a switch for the given package.
*
*
*
* @param ePackage
* the package in question.
* @return whether this is a switch for the given package.
* @generated
*/
@Override
protected boolean isSwitchFor(final EPackage ePackage) {
return ePackage == modelPackage;
}
/**
* Calls caseXXX
for each class of the model until one returns a non null result; it yields that result.
*
*
*
* @return the first non-null result returned by a caseXXX
call.
* @generated
*/
@Override
protected T doSwitch(final int classifierID, final EObject theEObject) {
switch (classifierID) {
case AssemblyPackage.ASSEMBLY_MODEL: {
final AssemblyModel assemblyModel = (AssemblyModel) theEObject;
T result = this.caseAssemblyModel(assemblyModel);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ESTRING_TO_ASSEMBLY_COMPONENT_MAP_ENTRY: {
@SuppressWarnings("unchecked")
final Map.Entry eStringToAssemblyComponentMapEntry = (Map.Entry) theEObject;
T result = this.caseEStringToAssemblyComponentMapEntry(eStringToAssemblyComponentMapEntry);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ASSEMBLY_COMPONENT: {
final AssemblyComponent assemblyComponent = (AssemblyComponent) theEObject;
T result = this.caseAssemblyComponent(assemblyComponent);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ESTRING_TO_ASSEMBLY_OPERATION_MAP_ENTRY: {
@SuppressWarnings("unchecked")
final Map.Entry eStringToAssemblyOperationMapEntry = (Map.Entry) theEObject;
T result = this.caseEStringToAssemblyOperationMapEntry(eStringToAssemblyOperationMapEntry);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ASSEMBLY_OPERATION: {
final AssemblyOperation assemblyOperation = (AssemblyOperation) theEObject;
T result = this.caseAssemblyOperation(assemblyOperation);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ASSEMBLY_STORAGE: {
final AssemblyStorage assemblyStorage = (AssemblyStorage) theEObject;
T result = this.caseAssemblyStorage(assemblyStorage);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ESTRING_TO_ASSEMBLY_STORAGE_MAP_ENTRY: {
@SuppressWarnings("unchecked")
final Map.Entry eStringToAssemblyStorageMapEntry = (Map.Entry) theEObject;
T result = this.caseEStringToAssemblyStorageMapEntry(eStringToAssemblyStorageMapEntry);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ASSEMBLY_PROVIDED_INTERFACE: {
final AssemblyProvidedInterface assemblyProvidedInterface = (AssemblyProvidedInterface) theEObject;
T result = this.caseAssemblyProvidedInterface(assemblyProvidedInterface);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ESTRING_TO_ASSEMBLY_PROVIDED_INTERFACE_MAP_ENTRY: {
@SuppressWarnings("unchecked")
final Map.Entry eStringToAssemblyProvidedInterfaceMapEntry = (Map.Entry) theEObject;
T result = this.caseEStringToAssemblyProvidedInterfaceMapEntry(eStringToAssemblyProvidedInterfaceMapEntry);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
case AssemblyPackage.ASSEMBLY_REQUIRED_INTERFACE: {
final AssemblyRequiredInterface assemblyRequiredInterface = (AssemblyRequiredInterface) theEObject;
T result = this.caseAssemblyRequiredInterface(assemblyRequiredInterface);
if (result == null) {
result = this.defaultCase(theEObject);
}
return result;
}
default:
return this.defaultCase(theEObject);
}
}
/**
* Returns the result of interpreting the object as an instance of 'Model'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'Model'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAssemblyModel(final AssemblyModel object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EString To Assembly Component Map Entry'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'EString To Assembly Component Map Entry'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseEStringToAssemblyComponentMapEntry(final Map.Entry object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Component'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'Component'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAssemblyComponent(final AssemblyComponent object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EString To Assembly Operation Map Entry'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'EString To Assembly Operation Map Entry'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseEStringToAssemblyOperationMapEntry(final Map.Entry object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Operation'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'Operation'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAssemblyOperation(final AssemblyOperation object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Storage'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'Storage'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAssemblyStorage(final AssemblyStorage object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EString To Assembly Storage Map Entry'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'EString To Assembly Storage Map Entry'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseEStringToAssemblyStorageMapEntry(final Map.Entry object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Provided Interface'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'Provided Interface'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAssemblyProvidedInterface(final AssemblyProvidedInterface object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EString To Assembly Provided Interface Map Entry'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'EString To Assembly Provided Interface Map Entry'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseEStringToAssemblyProvidedInterfaceMapEntry(final Map.Entry object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'Required Interface'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'Required Interface'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject) doSwitch(EObject)
* @generated
*/
public T caseAssemblyRequiredInterface(final AssemblyRequiredInterface object) {
return null;
}
/**
* Returns the result of interpreting the object as an instance of 'EObject'.
*
* This implementation returns null;
* returning a non-null result will terminate the switch, but this is the last case anyway.
*
*
* @param object
* the target of the switch.
* @return the result of interpreting the object as an instance of 'EObject'.
* @see #doSwitch(org.eclipse.emf.ecore.EObject)
* @generated
*/
@Override
public T defaultCase(final EObject object) {
return null;
}
} // AssemblySwitch
© 2015 - 2024 Weber Informatics LLC | Privacy Policy