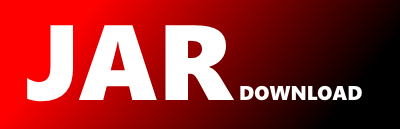
kieker.model.analysismodel.statistics.StatisticsPackage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kieker Show documentation
Show all versions of kieker Show documentation
Kieker: Application Performance Monitoring and Dynamic Software Analysis
/**
*/
package kieker.model.analysismodel.statistics;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EEnum;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.EReference;
/**
*
* The Package for the model.
* It contains accessors for the meta objects to represent
*
* - each class,
* - each feature of each class,
* - each operation of each class,
* - each enum,
* - and each data type
*
*
*
* @see kieker.model.analysismodel.statistics.StatisticsFactory
* @model kind="package"
* @generated
*/
public interface StatisticsPackage extends EPackage {
/**
* The package name.
*
*
*
* @generated
*/
String eNAME = "statistics";
/**
* The package namespace URI.
*
*
*
* @generated
*/
String eNS_URI = "platform:/resource/Kieker/model/analysismodel.ecore/statistics";
/**
* The package namespace name.
*
*
*
* @generated
*/
String eNS_PREFIX = "statistics";
/**
* The singleton instance of the package.
*
*
*
* @generated
*/
StatisticsPackage eINSTANCE = kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl.init();
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.StatisticRecordImpl Statistic Record}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.StatisticRecordImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getStatisticRecord()
* @generated
*/
int STATISTIC_RECORD = 0;
/**
* The feature id for the 'Properties' map.
*
*
*
* @generated
* @ordered
*/
int STATISTIC_RECORD__PROPERTIES = 0;
/**
* The number of structural features of the 'Statistic Record' class.
*
*
*
* @generated
* @ordered
*/
int STATISTIC_RECORD_FEATURE_COUNT = 1;
/**
* The number of operations of the 'Statistic Record' class.
*
*
*
* @generated
* @ordered
*/
int STATISTIC_RECORD_OPERATION_COUNT = 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.EPropertyTypeToValueImpl EProperty Type To Value}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.EPropertyTypeToValueImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getEPropertyTypeToValue()
* @generated
*/
int EPROPERTY_TYPE_TO_VALUE = 1;
/**
* The feature id for the 'Key' attribute.
*
*
*
* @generated
* @ordered
*/
int EPROPERTY_TYPE_TO_VALUE__KEY = 0;
/**
* The feature id for the 'Value' attribute.
*
*
*
* @generated
* @ordered
*/
int EPROPERTY_TYPE_TO_VALUE__VALUE = 1;
/**
* The number of structural features of the 'EProperty Type To Value' class.
*
*
*
* @generated
* @ordered
*/
int EPROPERTY_TYPE_TO_VALUE_FEATURE_COUNT = 2;
/**
* The number of operations of the 'EProperty Type To Value' class.
*
*
*
* @generated
* @ordered
*/
int EPROPERTY_TYPE_TO_VALUE_OPERATION_COUNT = 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.MeasurementImpl Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.MeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getMeasurement()
* @generated
*/
int MEASUREMENT = 2;
/**
* The feature id for the 'Timestamp' attribute.
*
*
*
* @generated
* @ordered
*/
int MEASUREMENT__TIMESTAMP = 0;
/**
* The number of structural features of the 'Measurement' class.
*
*
*
* @generated
* @ordered
*/
int MEASUREMENT_FEATURE_COUNT = 1;
/**
* The number of operations of the 'Measurement' class.
*
*
*
* @generated
* @ordered
*/
int MEASUREMENT_OPERATION_COUNT = 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.ScalarMeasurementImpl Scalar Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.ScalarMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getScalarMeasurement()
* @generated
*/
int SCALAR_MEASUREMENT = 3;
/**
* The feature id for the 'Timestamp' attribute.
*
*
*
* @generated
* @ordered
*/
int SCALAR_MEASUREMENT__TIMESTAMP = MEASUREMENT__TIMESTAMP;
/**
* The feature id for the 'Unit' reference.
*
*
*
* @generated
* @ordered
*/
int SCALAR_MEASUREMENT__UNIT = MEASUREMENT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Scalar Measurement' class.
*
*
*
* @generated
* @ordered
*/
int SCALAR_MEASUREMENT_FEATURE_COUNT = MEASUREMENT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'Scalar Measurement' class.
*
*
*
* @generated
* @ordered
*/
int SCALAR_MEASUREMENT_OPERATION_COUNT = MEASUREMENT_OPERATION_COUNT + 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.VectorMeasurementImpl Vector Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.VectorMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getVectorMeasurement()
* @generated
*/
int VECTOR_MEASUREMENT = 4;
/**
* The feature id for the 'Timestamp' attribute.
*
*
*
* @generated
* @ordered
*/
int VECTOR_MEASUREMENT__TIMESTAMP = MEASUREMENT__TIMESTAMP;
/**
* The feature id for the 'Values' containment reference list.
*
*
*
* @generated
* @ordered
*/
int VECTOR_MEASUREMENT__VALUES = MEASUREMENT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Vector Measurement' class.
*
*
*
* @generated
* @ordered
*/
int VECTOR_MEASUREMENT_FEATURE_COUNT = MEASUREMENT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'Vector Measurement' class.
*
*
*
* @generated
* @ordered
*/
int VECTOR_MEASUREMENT_OPERATION_COUNT = MEASUREMENT_OPERATION_COUNT + 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.IntMeasurementImpl Int Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.IntMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getIntMeasurement()
* @generated
*/
int INT_MEASUREMENT = 5;
/**
* The feature id for the 'Timestamp' attribute.
*
*
*
* @generated
* @ordered
*/
int INT_MEASUREMENT__TIMESTAMP = SCALAR_MEASUREMENT__TIMESTAMP;
/**
* The feature id for the 'Unit' reference.
*
*
*
* @generated
* @ordered
*/
int INT_MEASUREMENT__UNIT = SCALAR_MEASUREMENT__UNIT;
/**
* The feature id for the 'Value' attribute.
*
*
*
* @generated
* @ordered
*/
int INT_MEASUREMENT__VALUE = SCALAR_MEASUREMENT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Int Measurement' class.
*
*
*
* @generated
* @ordered
*/
int INT_MEASUREMENT_FEATURE_COUNT = SCALAR_MEASUREMENT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'Int Measurement' class.
*
*
*
* @generated
* @ordered
*/
int INT_MEASUREMENT_OPERATION_COUNT = SCALAR_MEASUREMENT_OPERATION_COUNT + 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.LongMeasurementImpl Long Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.LongMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getLongMeasurement()
* @generated
*/
int LONG_MEASUREMENT = 6;
/**
* The feature id for the 'Timestamp' attribute.
*
*
*
* @generated
* @ordered
*/
int LONG_MEASUREMENT__TIMESTAMP = SCALAR_MEASUREMENT__TIMESTAMP;
/**
* The feature id for the 'Unit' reference.
*
*
*
* @generated
* @ordered
*/
int LONG_MEASUREMENT__UNIT = SCALAR_MEASUREMENT__UNIT;
/**
* The feature id for the 'Value' attribute.
*
*
*
* @generated
* @ordered
*/
int LONG_MEASUREMENT__VALUE = SCALAR_MEASUREMENT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Long Measurement' class.
*
*
*
* @generated
* @ordered
*/
int LONG_MEASUREMENT_FEATURE_COUNT = SCALAR_MEASUREMENT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'Long Measurement' class.
*
*
*
* @generated
* @ordered
*/
int LONG_MEASUREMENT_OPERATION_COUNT = SCALAR_MEASUREMENT_OPERATION_COUNT + 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.FloatMeasurementImpl Float Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.FloatMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getFloatMeasurement()
* @generated
*/
int FLOAT_MEASUREMENT = 7;
/**
* The feature id for the 'Timestamp' attribute.
*
*
*
* @generated
* @ordered
*/
int FLOAT_MEASUREMENT__TIMESTAMP = SCALAR_MEASUREMENT__TIMESTAMP;
/**
* The feature id for the 'Unit' reference.
*
*
*
* @generated
* @ordered
*/
int FLOAT_MEASUREMENT__UNIT = SCALAR_MEASUREMENT__UNIT;
/**
* The feature id for the 'Value' attribute.
*
*
*
* @generated
* @ordered
*/
int FLOAT_MEASUREMENT__VALUE = SCALAR_MEASUREMENT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Float Measurement' class.
*
*
*
* @generated
* @ordered
*/
int FLOAT_MEASUREMENT_FEATURE_COUNT = SCALAR_MEASUREMENT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'Float Measurement' class.
*
*
*
* @generated
* @ordered
*/
int FLOAT_MEASUREMENT_OPERATION_COUNT = SCALAR_MEASUREMENT_OPERATION_COUNT + 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.DoubleMeasurementImpl Double Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.DoubleMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getDoubleMeasurement()
* @generated
*/
int DOUBLE_MEASUREMENT = 8;
/**
* The feature id for the 'Timestamp' attribute.
*
*
*
* @generated
* @ordered
*/
int DOUBLE_MEASUREMENT__TIMESTAMP = SCALAR_MEASUREMENT__TIMESTAMP;
/**
* The feature id for the 'Unit' reference.
*
*
*
* @generated
* @ordered
*/
int DOUBLE_MEASUREMENT__UNIT = SCALAR_MEASUREMENT__UNIT;
/**
* The feature id for the 'Value' attribute.
*
*
*
* @generated
* @ordered
*/
int DOUBLE_MEASUREMENT__VALUE = SCALAR_MEASUREMENT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Double Measurement' class.
*
*
*
* @generated
* @ordered
*/
int DOUBLE_MEASUREMENT_FEATURE_COUNT = SCALAR_MEASUREMENT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'Double Measurement' class.
*
*
*
* @generated
* @ordered
*/
int DOUBLE_MEASUREMENT_OPERATION_COUNT = SCALAR_MEASUREMENT_OPERATION_COUNT + 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.UnitImpl Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.UnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getUnit()
* @generated
*/
int UNIT = 11;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.ComposedUnitImpl Composed Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.ComposedUnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getComposedUnit()
* @generated
*/
int COMPOSED_UNIT = 12;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.SimpleUnitImpl Simple Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.SimpleUnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getSimpleUnit()
* @generated
*/
int SIMPLE_UNIT = 13;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.SIUnitImpl SI Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.SIUnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getSIUnit()
* @generated
*/
int SI_UNIT = 14;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.CustomUnitImpl Custom Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.CustomUnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getCustomUnit()
* @generated
*/
int CUSTOM_UNIT = 15;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.ESIUnitType ESI Unit Type}' enum.
*
*
*
* @see kieker.model.analysismodel.statistics.ESIUnitType
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getESIUnitType()
* @generated
*/
int ESI_UNIT_TYPE = 16;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.EPrefix EPrefix}' enum.
*
*
*
* @see kieker.model.analysismodel.statistics.EPrefix
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getEPrefix()
* @generated
*/
int EPREFIX = 17;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.StatisticsModelImpl Model}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.StatisticsModelImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getStatisticsModel()
* @generated
*/
int STATISTICS_MODEL = 9;
/**
* The feature id for the 'Statistics' map.
*
*
*
* @generated
* @ordered
*/
int STATISTICS_MODEL__STATISTICS = 0;
/**
* The feature id for the 'Units' containment reference list.
*
*
*
* @generated
* @ordered
*/
int STATISTICS_MODEL__UNITS = 1;
/**
* The number of structural features of the 'Model' class.
*
*
*
* @generated
* @ordered
*/
int STATISTICS_MODEL_FEATURE_COUNT = 2;
/**
* The number of operations of the 'Model' class.
*
*
*
* @generated
* @ordered
*/
int STATISTICS_MODEL_OPERATION_COUNT = 0;
/**
* The meta object id for the '{@link kieker.model.analysismodel.statistics.impl.EObjectToStatisticsMapEntryImpl EObject To Statistics Map Entry}'
* class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.EObjectToStatisticsMapEntryImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getEObjectToStatisticsMapEntry()
* @generated
*/
int EOBJECT_TO_STATISTICS_MAP_ENTRY = 10;
/**
* The feature id for the 'Value' containment reference.
*
*
*
* @generated
* @ordered
*/
int EOBJECT_TO_STATISTICS_MAP_ENTRY__VALUE = 0;
/**
* The feature id for the 'Key' reference.
*
*
*
* @generated
* @ordered
*/
int EOBJECT_TO_STATISTICS_MAP_ENTRY__KEY = 1;
/**
* The number of structural features of the 'EObject To Statistics Map Entry' class.
*
*
*
* @generated
* @ordered
*/
int EOBJECT_TO_STATISTICS_MAP_ENTRY_FEATURE_COUNT = 2;
/**
* The number of operations of the 'EObject To Statistics Map Entry' class.
*
*
*
* @generated
* @ordered
*/
int EOBJECT_TO_STATISTICS_MAP_ENTRY_OPERATION_COUNT = 0;
/**
* The number of structural features of the 'Unit' class.
*
*
*
* @generated
* @ordered
*/
int UNIT_FEATURE_COUNT = 0;
/**
* The number of operations of the 'Unit' class.
*
*
*
* @generated
* @ordered
*/
int UNIT_OPERATION_COUNT = 0;
/**
* The feature id for the 'Units' containment reference list.
*
*
*
* @generated
* @ordered
*/
int COMPOSED_UNIT__UNITS = 0;
/**
* The feature id for the 'Exponent' attribute.
*
*
*
* @generated
* @ordered
*/
int COMPOSED_UNIT__EXPONENT = 1;
/**
* The number of structural features of the 'Composed Unit' class.
*
*
*
* @generated
* @ordered
*/
int COMPOSED_UNIT_FEATURE_COUNT = 2;
/**
* The number of operations of the 'Composed Unit' class.
*
*
*
* @generated
* @ordered
*/
int COMPOSED_UNIT_OPERATION_COUNT = 0;
/**
* The feature id for the 'Prefix' attribute.
*
*
*
* @generated
* @ordered
*/
int SIMPLE_UNIT__PREFIX = UNIT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Simple Unit' class.
*
*
*
* @generated
* @ordered
*/
int SIMPLE_UNIT_FEATURE_COUNT = UNIT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'Simple Unit' class.
*
*
*
* @generated
* @ordered
*/
int SIMPLE_UNIT_OPERATION_COUNT = UNIT_OPERATION_COUNT + 0;
/**
* The feature id for the 'Prefix' attribute.
*
*
*
* @generated
* @ordered
*/
int SI_UNIT__PREFIX = SIMPLE_UNIT__PREFIX;
/**
* The feature id for the 'Unit Type' attribute.
*
*
*
* @generated
* @ordered
*/
int SI_UNIT__UNIT_TYPE = SIMPLE_UNIT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'SI Unit' class.
*
*
*
* @generated
* @ordered
*/
int SI_UNIT_FEATURE_COUNT = SIMPLE_UNIT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'SI Unit' class.
*
*
*
* @generated
* @ordered
*/
int SI_UNIT_OPERATION_COUNT = SIMPLE_UNIT_OPERATION_COUNT + 0;
/**
* The feature id for the 'Prefix' attribute.
*
*
*
* @generated
* @ordered
*/
int CUSTOM_UNIT__PREFIX = SIMPLE_UNIT__PREFIX;
/**
* The feature id for the 'Name' attribute.
*
*
*
* @generated
* @ordered
*/
int CUSTOM_UNIT__NAME = SIMPLE_UNIT_FEATURE_COUNT + 0;
/**
* The number of structural features of the 'Custom Unit' class.
*
*
*
* @generated
* @ordered
*/
int CUSTOM_UNIT_FEATURE_COUNT = SIMPLE_UNIT_FEATURE_COUNT + 1;
/**
* The number of operations of the 'Custom Unit' class.
*
*
*
* @generated
* @ordered
*/
int CUSTOM_UNIT_OPERATION_COUNT = SIMPLE_UNIT_OPERATION_COUNT + 0;
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.StatisticRecord Statistic Record}'.
*
*
*
* @return the meta object for class 'Statistic Record'.
* @see kieker.model.analysismodel.statistics.StatisticRecord
* @generated
*/
EClass getStatisticRecord();
/**
* Returns the meta object for the map '{@link kieker.model.analysismodel.statistics.StatisticRecord#getProperties Properties}'.
*
*
*
* @return the meta object for the map 'Properties'.
* @see kieker.model.analysismodel.statistics.StatisticRecord#getProperties()
* @see #getStatisticRecord()
* @generated
*/
EReference getStatisticRecord_Properties();
/**
* Returns the meta object for class '{@link java.util.Map.Entry EProperty Type To Value}'.
*
*
*
* @return the meta object for class 'EProperty Type To Value'.
* @see java.util.Map.Entry
* @model keyDataType="org.eclipse.emf.ecore.EString"
* valueDataType="org.eclipse.emf.ecore.EJavaObject"
* @generated
*/
EClass getEPropertyTypeToValue();
/**
* Returns the meta object for the attribute '{@link java.util.Map.Entry Key}'.
*
*
*
* @return the meta object for the attribute 'Key'.
* @see java.util.Map.Entry
* @see #getEPropertyTypeToValue()
* @generated
*/
EAttribute getEPropertyTypeToValue_Key();
/**
* Returns the meta object for the attribute '{@link java.util.Map.Entry Value}'.
*
*
*
* @return the meta object for the attribute 'Value'.
* @see java.util.Map.Entry
* @see #getEPropertyTypeToValue()
* @generated
*/
EAttribute getEPropertyTypeToValue_Value();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.Measurement Measurement}'.
*
*
*
* @return the meta object for class 'Measurement'.
* @see kieker.model.analysismodel.statistics.Measurement
* @generated
*/
EClass getMeasurement();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.Measurement#getTimestamp Timestamp}'.
*
*
*
* @return the meta object for the attribute 'Timestamp'.
* @see kieker.model.analysismodel.statistics.Measurement#getTimestamp()
* @see #getMeasurement()
* @generated
*/
EAttribute getMeasurement_Timestamp();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.ScalarMeasurement Scalar Measurement}'.
*
*
*
* @return the meta object for class 'Scalar Measurement'.
* @see kieker.model.analysismodel.statistics.ScalarMeasurement
* @generated
*/
EClass getScalarMeasurement();
/**
* Returns the meta object for the reference '{@link kieker.model.analysismodel.statistics.ScalarMeasurement#getUnit Unit}'.
*
*
*
* @return the meta object for the reference 'Unit'.
* @see kieker.model.analysismodel.statistics.ScalarMeasurement#getUnit()
* @see #getScalarMeasurement()
* @generated
*/
EReference getScalarMeasurement_Unit();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.VectorMeasurement Vector Measurement}'.
*
*
*
* @return the meta object for class 'Vector Measurement'.
* @see kieker.model.analysismodel.statistics.VectorMeasurement
* @generated
*/
EClass getVectorMeasurement();
/**
* Returns the meta object for the containment reference list '{@link kieker.model.analysismodel.statistics.VectorMeasurement#getValues Values}'.
*
*
*
* @return the meta object for the containment reference list 'Values'.
* @see kieker.model.analysismodel.statistics.VectorMeasurement#getValues()
* @see #getVectorMeasurement()
* @generated
*/
EReference getVectorMeasurement_Values();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.IntMeasurement Int Measurement}'.
*
*
*
* @return the meta object for class 'Int Measurement'.
* @see kieker.model.analysismodel.statistics.IntMeasurement
* @generated
*/
EClass getIntMeasurement();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.IntMeasurement#getValue Value}'.
*
*
*
* @return the meta object for the attribute 'Value'.
* @see kieker.model.analysismodel.statistics.IntMeasurement#getValue()
* @see #getIntMeasurement()
* @generated
*/
EAttribute getIntMeasurement_Value();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.LongMeasurement Long Measurement}'.
*
*
*
* @return the meta object for class 'Long Measurement'.
* @see kieker.model.analysismodel.statistics.LongMeasurement
* @generated
*/
EClass getLongMeasurement();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.LongMeasurement#getValue Value}'.
*
*
*
* @return the meta object for the attribute 'Value'.
* @see kieker.model.analysismodel.statistics.LongMeasurement#getValue()
* @see #getLongMeasurement()
* @generated
*/
EAttribute getLongMeasurement_Value();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.FloatMeasurement Float Measurement}'.
*
*
*
* @return the meta object for class 'Float Measurement'.
* @see kieker.model.analysismodel.statistics.FloatMeasurement
* @generated
*/
EClass getFloatMeasurement();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.FloatMeasurement#getValue Value}'.
*
*
*
* @return the meta object for the attribute 'Value'.
* @see kieker.model.analysismodel.statistics.FloatMeasurement#getValue()
* @see #getFloatMeasurement()
* @generated
*/
EAttribute getFloatMeasurement_Value();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.DoubleMeasurement Double Measurement}'.
*
*
*
* @return the meta object for class 'Double Measurement'.
* @see kieker.model.analysismodel.statistics.DoubleMeasurement
* @generated
*/
EClass getDoubleMeasurement();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.DoubleMeasurement#getValue Value}'.
*
*
*
* @return the meta object for the attribute 'Value'.
* @see kieker.model.analysismodel.statistics.DoubleMeasurement#getValue()
* @see #getDoubleMeasurement()
* @generated
*/
EAttribute getDoubleMeasurement_Value();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.Unit Unit}'.
*
*
*
* @return the meta object for class 'Unit'.
* @see kieker.model.analysismodel.statistics.Unit
* @generated
*/
EClass getUnit();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.ComposedUnit Composed Unit}'.
*
*
*
* @return the meta object for class 'Composed Unit'.
* @see kieker.model.analysismodel.statistics.ComposedUnit
* @generated
*/
EClass getComposedUnit();
/**
* Returns the meta object for the containment reference list '{@link kieker.model.analysismodel.statistics.ComposedUnit#getUnits Units}'.
*
*
*
* @return the meta object for the containment reference list 'Units'.
* @see kieker.model.analysismodel.statistics.ComposedUnit#getUnits()
* @see #getComposedUnit()
* @generated
*/
EReference getComposedUnit_Units();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.ComposedUnit#getExponent Exponent}'.
*
*
*
* @return the meta object for the attribute 'Exponent'.
* @see kieker.model.analysismodel.statistics.ComposedUnit#getExponent()
* @see #getComposedUnit()
* @generated
*/
EAttribute getComposedUnit_Exponent();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.SimpleUnit Simple Unit}'.
*
*
*
* @return the meta object for class 'Simple Unit'.
* @see kieker.model.analysismodel.statistics.SimpleUnit
* @generated
*/
EClass getSimpleUnit();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.SimpleUnit#getPrefix Prefix}'.
*
*
*
* @return the meta object for the attribute 'Prefix'.
* @see kieker.model.analysismodel.statistics.SimpleUnit#getPrefix()
* @see #getSimpleUnit()
* @generated
*/
EAttribute getSimpleUnit_Prefix();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.SIUnit SI Unit}'.
*
*
*
* @return the meta object for class 'SI Unit'.
* @see kieker.model.analysismodel.statistics.SIUnit
* @generated
*/
EClass getSIUnit();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.SIUnit#getUnitType Unit Type}'.
*
*
*
* @return the meta object for the attribute 'Unit Type'.
* @see kieker.model.analysismodel.statistics.SIUnit#getUnitType()
* @see #getSIUnit()
* @generated
*/
EAttribute getSIUnit_UnitType();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.CustomUnit Custom Unit}'.
*
*
*
* @return the meta object for class 'Custom Unit'.
* @see kieker.model.analysismodel.statistics.CustomUnit
* @generated
*/
EClass getCustomUnit();
/**
* Returns the meta object for the attribute '{@link kieker.model.analysismodel.statistics.CustomUnit#getName Name}'.
*
*
*
* @return the meta object for the attribute 'Name'.
* @see kieker.model.analysismodel.statistics.CustomUnit#getName()
* @see #getCustomUnit()
* @generated
*/
EAttribute getCustomUnit_Name();
/**
* Returns the meta object for enum '{@link kieker.model.analysismodel.statistics.ESIUnitType ESI Unit Type}'.
*
*
*
* @return the meta object for enum 'ESI Unit Type'.
* @see kieker.model.analysismodel.statistics.ESIUnitType
* @generated
*/
EEnum getESIUnitType();
/**
* Returns the meta object for enum '{@link kieker.model.analysismodel.statistics.EPrefix EPrefix}'.
*
*
*
* @return the meta object for enum 'EPrefix'.
* @see kieker.model.analysismodel.statistics.EPrefix
* @generated
*/
EEnum getEPrefix();
/**
* Returns the meta object for class '{@link kieker.model.analysismodel.statistics.StatisticsModel Model}'.
*
*
*
* @return the meta object for class 'Model'.
* @see kieker.model.analysismodel.statistics.StatisticsModel
* @generated
*/
EClass getStatisticsModel();
/**
* Returns the meta object for the map '{@link kieker.model.analysismodel.statistics.StatisticsModel#getStatistics Statistics}'.
*
*
*
* @return the meta object for the map 'Statistics'.
* @see kieker.model.analysismodel.statistics.StatisticsModel#getStatistics()
* @see #getStatisticsModel()
* @generated
*/
EReference getStatisticsModel_Statistics();
/**
* Returns the meta object for the containment reference list '{@link kieker.model.analysismodel.statistics.StatisticsModel#getUnits Units}'.
*
*
*
* @return the meta object for the containment reference list 'Units'.
* @see kieker.model.analysismodel.statistics.StatisticsModel#getUnits()
* @see #getStatisticsModel()
* @generated
*/
EReference getStatisticsModel_Units();
/**
* Returns the meta object for class '{@link java.util.Map.Entry EObject To Statistics Map Entry}'.
*
*
*
* @return the meta object for class 'EObject To Statistics Map Entry'.
* @see java.util.Map.Entry
* @model features="value key"
* valueType="kieker.model.analysismodel.statistics.StatisticRecord" valueContainment="true"
* keyType="org.eclipse.emf.ecore.EObject"
* @generated
*/
EClass getEObjectToStatisticsMapEntry();
/**
* Returns the meta object for the containment reference '{@link java.util.Map.Entry Value}'.
*
*
*
* @return the meta object for the containment reference 'Value'.
* @see java.util.Map.Entry
* @see #getEObjectToStatisticsMapEntry()
* @generated
*/
EReference getEObjectToStatisticsMapEntry_Value();
/**
* Returns the meta object for the reference '{@link java.util.Map.Entry Key}'.
*
*
*
* @return the meta object for the reference 'Key'.
* @see java.util.Map.Entry
* @see #getEObjectToStatisticsMapEntry()
* @generated
*/
EReference getEObjectToStatisticsMapEntry_Key();
/**
* Returns the factory that creates the instances of the model.
*
*
*
* @return the factory that creates the instances of the model.
* @generated
*/
StatisticsFactory getStatisticsFactory();
/**
*
* Defines literals for the meta objects that represent
*
* - each class,
* - each feature of each class,
* - each operation of each class,
* - each enum,
* - and each data type
*
*
*
* @generated
*/
interface Literals {
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.StatisticRecordImpl Statistic Record}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.StatisticRecordImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getStatisticRecord()
* @generated
*/
EClass STATISTIC_RECORD = eINSTANCE.getStatisticRecord();
/**
* The meta object literal for the 'Properties' map feature.
*
*
*
* @generated
*/
EReference STATISTIC_RECORD__PROPERTIES = eINSTANCE.getStatisticRecord_Properties();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.EPropertyTypeToValueImpl EProperty Type To Value}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.EPropertyTypeToValueImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getEPropertyTypeToValue()
* @generated
*/
EClass EPROPERTY_TYPE_TO_VALUE = eINSTANCE.getEPropertyTypeToValue();
/**
* The meta object literal for the 'Key' attribute feature.
*
*
*
* @generated
*/
EAttribute EPROPERTY_TYPE_TO_VALUE__KEY = eINSTANCE.getEPropertyTypeToValue_Key();
/**
* The meta object literal for the 'Value' attribute feature.
*
*
*
* @generated
*/
EAttribute EPROPERTY_TYPE_TO_VALUE__VALUE = eINSTANCE.getEPropertyTypeToValue_Value();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.MeasurementImpl Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.MeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getMeasurement()
* @generated
*/
EClass MEASUREMENT = eINSTANCE.getMeasurement();
/**
* The meta object literal for the 'Timestamp' attribute feature.
*
*
*
* @generated
*/
EAttribute MEASUREMENT__TIMESTAMP = eINSTANCE.getMeasurement_Timestamp();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.ScalarMeasurementImpl Scalar Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.ScalarMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getScalarMeasurement()
* @generated
*/
EClass SCALAR_MEASUREMENT = eINSTANCE.getScalarMeasurement();
/**
* The meta object literal for the 'Unit' reference feature.
*
*
*
* @generated
*/
EReference SCALAR_MEASUREMENT__UNIT = eINSTANCE.getScalarMeasurement_Unit();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.VectorMeasurementImpl Vector Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.VectorMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getVectorMeasurement()
* @generated
*/
EClass VECTOR_MEASUREMENT = eINSTANCE.getVectorMeasurement();
/**
* The meta object literal for the 'Values' containment reference list feature.
*
*
*
* @generated
*/
EReference VECTOR_MEASUREMENT__VALUES = eINSTANCE.getVectorMeasurement_Values();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.IntMeasurementImpl Int Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.IntMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getIntMeasurement()
* @generated
*/
EClass INT_MEASUREMENT = eINSTANCE.getIntMeasurement();
/**
* The meta object literal for the 'Value' attribute feature.
*
*
*
* @generated
*/
EAttribute INT_MEASUREMENT__VALUE = eINSTANCE.getIntMeasurement_Value();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.LongMeasurementImpl Long Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.LongMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getLongMeasurement()
* @generated
*/
EClass LONG_MEASUREMENT = eINSTANCE.getLongMeasurement();
/**
* The meta object literal for the 'Value' attribute feature.
*
*
*
* @generated
*/
EAttribute LONG_MEASUREMENT__VALUE = eINSTANCE.getLongMeasurement_Value();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.FloatMeasurementImpl Float Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.FloatMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getFloatMeasurement()
* @generated
*/
EClass FLOAT_MEASUREMENT = eINSTANCE.getFloatMeasurement();
/**
* The meta object literal for the 'Value' attribute feature.
*
*
*
* @generated
*/
EAttribute FLOAT_MEASUREMENT__VALUE = eINSTANCE.getFloatMeasurement_Value();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.DoubleMeasurementImpl Double Measurement}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.DoubleMeasurementImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getDoubleMeasurement()
* @generated
*/
EClass DOUBLE_MEASUREMENT = eINSTANCE.getDoubleMeasurement();
/**
* The meta object literal for the 'Value' attribute feature.
*
*
*
* @generated
*/
EAttribute DOUBLE_MEASUREMENT__VALUE = eINSTANCE.getDoubleMeasurement_Value();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.UnitImpl Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.UnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getUnit()
* @generated
*/
EClass UNIT = eINSTANCE.getUnit();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.ComposedUnitImpl Composed Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.ComposedUnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getComposedUnit()
* @generated
*/
EClass COMPOSED_UNIT = eINSTANCE.getComposedUnit();
/**
* The meta object literal for the 'Units' containment reference list feature.
*
*
*
* @generated
*/
EReference COMPOSED_UNIT__UNITS = eINSTANCE.getComposedUnit_Units();
/**
* The meta object literal for the 'Exponent' attribute feature.
*
*
*
* @generated
*/
EAttribute COMPOSED_UNIT__EXPONENT = eINSTANCE.getComposedUnit_Exponent();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.SimpleUnitImpl Simple Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.SimpleUnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getSimpleUnit()
* @generated
*/
EClass SIMPLE_UNIT = eINSTANCE.getSimpleUnit();
/**
* The meta object literal for the 'Prefix' attribute feature.
*
*
*
* @generated
*/
EAttribute SIMPLE_UNIT__PREFIX = eINSTANCE.getSimpleUnit_Prefix();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.SIUnitImpl SI Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.SIUnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getSIUnit()
* @generated
*/
EClass SI_UNIT = eINSTANCE.getSIUnit();
/**
* The meta object literal for the 'Unit Type' attribute feature.
*
*
*
* @generated
*/
EAttribute SI_UNIT__UNIT_TYPE = eINSTANCE.getSIUnit_UnitType();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.CustomUnitImpl Custom Unit}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.CustomUnitImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getCustomUnit()
* @generated
*/
EClass CUSTOM_UNIT = eINSTANCE.getCustomUnit();
/**
* The meta object literal for the 'Name' attribute feature.
*
*
*
* @generated
*/
EAttribute CUSTOM_UNIT__NAME = eINSTANCE.getCustomUnit_Name();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.ESIUnitType ESI Unit Type}' enum.
*
*
*
* @see kieker.model.analysismodel.statistics.ESIUnitType
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getESIUnitType()
* @generated
*/
EEnum ESI_UNIT_TYPE = eINSTANCE.getESIUnitType();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.EPrefix EPrefix}' enum.
*
*
*
* @see kieker.model.analysismodel.statistics.EPrefix
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getEPrefix()
* @generated
*/
EEnum EPREFIX = eINSTANCE.getEPrefix();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.StatisticsModelImpl Model}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.StatisticsModelImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getStatisticsModel()
* @generated
*/
EClass STATISTICS_MODEL = eINSTANCE.getStatisticsModel();
/**
* The meta object literal for the 'Statistics' map feature.
*
*
*
* @generated
*/
EReference STATISTICS_MODEL__STATISTICS = eINSTANCE.getStatisticsModel_Statistics();
/**
* The meta object literal for the 'Units' containment reference list feature.
*
*
*
* @generated
*/
EReference STATISTICS_MODEL__UNITS = eINSTANCE.getStatisticsModel_Units();
/**
* The meta object literal for the '{@link kieker.model.analysismodel.statistics.impl.EObjectToStatisticsMapEntryImpl EObject To Statistics Map
* Entry}' class.
*
*
*
* @see kieker.model.analysismodel.statistics.impl.EObjectToStatisticsMapEntryImpl
* @see kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl#getEObjectToStatisticsMapEntry()
* @generated
*/
EClass EOBJECT_TO_STATISTICS_MAP_ENTRY = eINSTANCE.getEObjectToStatisticsMapEntry();
/**
* The meta object literal for the 'Value' containment reference feature.
*
*
*
* @generated
*/
EReference EOBJECT_TO_STATISTICS_MAP_ENTRY__VALUE = eINSTANCE.getEObjectToStatisticsMapEntry_Value();
/**
* The meta object literal for the 'Key' reference feature.
*
*
*
* @generated
*/
EReference EOBJECT_TO_STATISTICS_MAP_ENTRY__KEY = eINSTANCE.getEObjectToStatisticsMapEntry_Key();
}
} // StatisticsPackage
© 2015 - 2024 Weber Informatics LLC | Privacy Policy