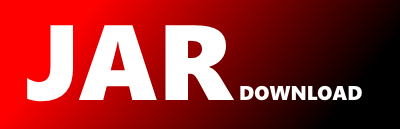
kieker.analysis.generic.sink.graph.dot.DotExportMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kieker Show documentation
Show all versions of kieker Show documentation
Kieker: Application Performance Monitoring and Dynamic Software Analysis
The newest version!
/***************************************************************************
* Copyright 2022 Kieker Project (http://kieker-monitoring.net)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
***************************************************************************/
package kieker.analysis.generic.sink.graph.dot;
import java.util.Collections;
import java.util.EnumMap;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import com.google.common.graph.MutableNetwork;
import kieker.analysis.generic.graph.IEdge;
import kieker.analysis.generic.graph.INode;
import kieker.analysis.generic.sink.graph.dot.attributes.DotClusterAttribute;
import kieker.analysis.generic.sink.graph.dot.attributes.DotEdgeAttribute;
import kieker.analysis.generic.sink.graph.dot.attributes.DotGraphAttribute;
import kieker.analysis.generic.sink.graph.dot.attributes.DotNodeAttribute;
/**
* This class specifies how attributes (for graphs, vertices and edges) are mapped
* to a dot graph.
*
* To create a {@link DotExportMapper} use the {@link DotExportMapper.Builder}.
*
* @param
* node type
* @param
* edge type
*
* @author Sören Henning
*
* @since 1.14
*/
public class DotExportMapper {
//
// BETTER Even if EnumMaps are very efficient in terms of lookup, etc. they require
// a constant memory space, which is the size of the enums list. Moreover, we only
// want to iterate over the map and do not perform any lookups etc. A more efficient
// way would be to use a List of Pairs of constant size.
//
// PMD: No concurrent access intended for the following attributes
protected final Map, String>> graphAttributes = new EnumMap<>(
DotGraphAttribute.class); // NOPMD (see above)
protected final Map, String>> defaultNodeAttributes = new EnumMap<>(
DotNodeAttribute.class); // NOPMD (see above)
protected final Map, String>> defaultEdgeAttributes = new EnumMap<>(
DotEdgeAttribute.class); // NOPMD (see above)
protected final Map> nodeAttributes = new EnumMap<>(DotNodeAttribute.class); // NOPMD (see above)
protected final Map> edgeAttributes = new EnumMap<>(DotEdgeAttribute.class); // NOPMD (see above)
protected final Map> clusterAttributes = new EnumMap<>(DotClusterAttribute.class); // NOPMD (see above)
protected DotExportMapper() {
// Create an empty export configuration
}
public Set, String>>> getGraphAttributes() {
return Collections.unmodifiableSet(this.graphAttributes.entrySet());
}
public Set, String>>> getDefaultNodeAttributes() {
return Collections.unmodifiableSet(this.defaultNodeAttributes.entrySet());
}
public Set, String>>> getDefaultEdgeAttributes() {
return Collections.unmodifiableSet(this.defaultEdgeAttributes.entrySet());
}
public Set>> getNodeAttributes() {
return Collections.unmodifiableSet(this.nodeAttributes.entrySet());
}
public Set>> getEdgeAttributes() {
return Collections.unmodifiableSet(this.edgeAttributes.entrySet());
}
public Set>> getClusterAttributes() {
return Collections.unmodifiableSet(this.clusterAttributes.entrySet());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy