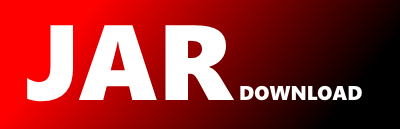
kieker.model.analysismodel.source.impl.SourcePackageImpl Maven / Gradle / Ivy
Show all versions of kieker Show documentation
/**
*/
package kieker.model.analysismodel.source.impl;
import java.util.Map;
import org.eclipse.emf.ecore.EAttribute;
import org.eclipse.emf.ecore.EClass;
import org.eclipse.emf.ecore.EPackage;
import org.eclipse.emf.ecore.EReference;
import org.eclipse.emf.ecore.impl.EPackageImpl;
import kieker.model.analysismodel.AnalysismodelPackage;
import kieker.model.analysismodel.assembly.AssemblyPackage;
import kieker.model.analysismodel.assembly.impl.AssemblyPackageImpl;
import kieker.model.analysismodel.deployment.DeploymentPackage;
import kieker.model.analysismodel.deployment.impl.DeploymentPackageImpl;
import kieker.model.analysismodel.execution.ExecutionPackage;
import kieker.model.analysismodel.execution.impl.ExecutionPackageImpl;
import kieker.model.analysismodel.impl.AnalysismodelPackageImpl;
import kieker.model.analysismodel.source.SourceFactory;
import kieker.model.analysismodel.source.SourceModel;
import kieker.model.analysismodel.source.SourcePackage;
import kieker.model.analysismodel.statistics.StatisticsPackage;
import kieker.model.analysismodel.statistics.impl.StatisticsPackageImpl;
import kieker.model.analysismodel.trace.TracePackage;
import kieker.model.analysismodel.trace.impl.TracePackageImpl;
import kieker.model.analysismodel.type.TypePackage;
import kieker.model.analysismodel.type.impl.TypePackageImpl;
/**
*
* An implementation of the model Package.
*
*
* @generated
*/
public class SourcePackageImpl extends EPackageImpl implements SourcePackage {
/**
*
*
*
* @generated
*/
private EClass sourceModelEClass = null;
/**
*
*
*
* @generated
*/
private EClass eObjectToSourceEntryEClass = null;
/**
* Creates an instance of the model Package, registered with
* {@link org.eclipse.emf.ecore.EPackage.Registry EPackage.Registry} by the package
* package URI value.
*
* Note: the correct way to create the package is via the static
* factory method {@link #init init()}, which also performs
* initialization of the package, or returns the registered package,
* if one already exists.
*
*
*
* @see org.eclipse.emf.ecore.EPackage.Registry
* @see kieker.model.analysismodel.source.SourcePackage#eNS_URI
* @see #init()
* @generated
*/
private SourcePackageImpl() {
super(eNS_URI, SourceFactory.eINSTANCE);
}
/**
*
*
*
* @generated
*/
private static boolean isInited = false;
/**
* Creates, registers, and initializes the Package for this model, and for any others upon which it depends.
*
*
* This method is used to initialize {@link SourcePackage#eINSTANCE} when that field is accessed.
* Clients should not invoke it directly. Instead, they should simply access that field to obtain the package.
*
*
*
* @see #eNS_URI
* @see #createPackageContents()
* @see #initializePackageContents()
* @generated
*/
public static SourcePackage init() {
if (isInited) {
return (SourcePackage) EPackage.Registry.INSTANCE.getEPackage(SourcePackage.eNS_URI);
}
// Obtain or create and register package
final Object registeredSourcePackage = EPackage.Registry.INSTANCE.get(eNS_URI);
final SourcePackageImpl theSourcePackage = registeredSourcePackage instanceof SourcePackageImpl ? (SourcePackageImpl) registeredSourcePackage
: new SourcePackageImpl();
isInited = true;
// Obtain or create and register interdependencies
Object registeredPackage = EPackage.Registry.INSTANCE.getEPackage(AnalysismodelPackage.eNS_URI);
final AnalysismodelPackageImpl theAnalysismodelPackage = (AnalysismodelPackageImpl) (registeredPackage instanceof AnalysismodelPackageImpl
? registeredPackage
: AnalysismodelPackage.eINSTANCE);
registeredPackage = EPackage.Registry.INSTANCE.getEPackage(StatisticsPackage.eNS_URI);
final StatisticsPackageImpl theStatisticsPackage = (StatisticsPackageImpl) (registeredPackage instanceof StatisticsPackageImpl ? registeredPackage
: StatisticsPackage.eINSTANCE);
registeredPackage = EPackage.Registry.INSTANCE.getEPackage(TypePackage.eNS_URI);
final TypePackageImpl theTypePackage = (TypePackageImpl) (registeredPackage instanceof TypePackageImpl ? registeredPackage : TypePackage.eINSTANCE);
registeredPackage = EPackage.Registry.INSTANCE.getEPackage(AssemblyPackage.eNS_URI);
final AssemblyPackageImpl theAssemblyPackage = (AssemblyPackageImpl) (registeredPackage instanceof AssemblyPackageImpl ? registeredPackage
: AssemblyPackage.eINSTANCE);
registeredPackage = EPackage.Registry.INSTANCE.getEPackage(DeploymentPackage.eNS_URI);
final DeploymentPackageImpl theDeploymentPackage = (DeploymentPackageImpl) (registeredPackage instanceof DeploymentPackageImpl ? registeredPackage
: DeploymentPackage.eINSTANCE);
registeredPackage = EPackage.Registry.INSTANCE.getEPackage(ExecutionPackage.eNS_URI);
final ExecutionPackageImpl theExecutionPackage = (ExecutionPackageImpl) (registeredPackage instanceof ExecutionPackageImpl ? registeredPackage
: ExecutionPackage.eINSTANCE);
registeredPackage = EPackage.Registry.INSTANCE.getEPackage(TracePackage.eNS_URI);
final TracePackageImpl theTracePackage = (TracePackageImpl) (registeredPackage instanceof TracePackageImpl ? registeredPackage : TracePackage.eINSTANCE);
// Create package meta-data objects
theSourcePackage.createPackageContents();
theAnalysismodelPackage.createPackageContents();
theStatisticsPackage.createPackageContents();
theTypePackage.createPackageContents();
theAssemblyPackage.createPackageContents();
theDeploymentPackage.createPackageContents();
theExecutionPackage.createPackageContents();
theTracePackage.createPackageContents();
// Initialize created meta-data
theSourcePackage.initializePackageContents();
theAnalysismodelPackage.initializePackageContents();
theStatisticsPackage.initializePackageContents();
theTypePackage.initializePackageContents();
theAssemblyPackage.initializePackageContents();
theDeploymentPackage.initializePackageContents();
theExecutionPackage.initializePackageContents();
theTracePackage.initializePackageContents();
// Mark meta-data to indicate it can't be changed
theSourcePackage.freeze();
// Update the registry and return the package
EPackage.Registry.INSTANCE.put(SourcePackage.eNS_URI, theSourcePackage);
return theSourcePackage;
}
/**
*
*
*
* @generated
*/
@Override
public EClass getSourceModel() {
return this.sourceModelEClass;
}
/**
*
*
*
* @generated
*/
@Override
public EReference getSourceModel_Sources() {
return (EReference) this.sourceModelEClass.getEStructuralFeatures().get(0);
}
/**
*
*
*
* @generated
*/
@Override
public EClass getEObjectToSourceEntry() {
return this.eObjectToSourceEntryEClass;
}
/**
*
*
*
* @generated
*/
@Override
public EReference getEObjectToSourceEntry_Key() {
return (EReference) this.eObjectToSourceEntryEClass.getEStructuralFeatures().get(0);
}
/**
*
*
*
* @generated
*/
@Override
public EAttribute getEObjectToSourceEntry_Value() {
return (EAttribute) this.eObjectToSourceEntryEClass.getEStructuralFeatures().get(1);
}
/**
*
*
*
* @generated
*/
@Override
public SourceFactory getSourceFactory() {
return (SourceFactory) this.getEFactoryInstance();
}
/**
*
*
*
* @generated
*/
private boolean isCreated = false;
/**
* Creates the meta-model objects for the package. This method is
* guarded to have no affect on any invocation but its first.
*
*
*
* @generated
*/
public void createPackageContents() {
if (this.isCreated) {
return;
}
this.isCreated = true;
// Create classes and their features
this.sourceModelEClass = this.createEClass(SOURCE_MODEL);
this.createEReference(this.sourceModelEClass, SOURCE_MODEL__SOURCES);
this.eObjectToSourceEntryEClass = this.createEClass(EOBJECT_TO_SOURCE_ENTRY);
this.createEReference(this.eObjectToSourceEntryEClass, EOBJECT_TO_SOURCE_ENTRY__KEY);
this.createEAttribute(this.eObjectToSourceEntryEClass, EOBJECT_TO_SOURCE_ENTRY__VALUE);
}
/**
*
*
*
* @generated
*/
private boolean isInitialized = false;
/**
* Complete the initialization of the package and its meta-model. This
* method is guarded to have no affect on any invocation but its first.
*
*
*
* @generated
*/
public void initializePackageContents() {
if (this.isInitialized) {
return;
}
this.isInitialized = true;
// Initialize package
this.setName(eNAME);
this.setNsPrefix(eNS_PREFIX);
this.setNsURI(eNS_URI);
// Create type parameters
// Set bounds for type parameters
// Add supertypes to classes
// Initialize classes, features, and operations; add parameters
this.initEClass(this.sourceModelEClass, SourceModel.class, "SourceModel", !IS_ABSTRACT, !IS_INTERFACE, IS_GENERATED_INSTANCE_CLASS);
this.initEReference(this.getSourceModel_Sources(), this.getEObjectToSourceEntry(), null, "sources", null, 0, -1, SourceModel.class, !IS_TRANSIENT,
!IS_VOLATILE,
IS_CHANGEABLE, IS_COMPOSITE, !IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
this.initEClass(this.eObjectToSourceEntryEClass, Map.Entry.class, "EObjectToSourceEntry", !IS_ABSTRACT, !IS_INTERFACE, !IS_GENERATED_INSTANCE_CLASS);
this.initEReference(this.getEObjectToSourceEntry_Key(), this.ecorePackage.getEObject(), null, "key", null, 0, 1, Map.Entry.class, !IS_TRANSIENT,
!IS_VOLATILE,
IS_CHANGEABLE, !IS_COMPOSITE, IS_RESOLVE_PROXIES, !IS_UNSETTABLE, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
this.initEAttribute(this.getEObjectToSourceEntry_Value(), this.ecorePackage.getEString(), "value", null, 0, -1, Map.Entry.class, !IS_TRANSIENT, !IS_VOLATILE,
IS_CHANGEABLE,
!IS_UNSETTABLE, !IS_ID, IS_UNIQUE, !IS_DERIVED, IS_ORDERED);
}
} // SourcePackageImpl