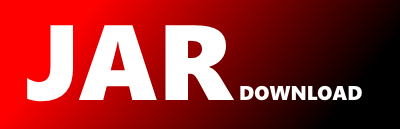
kieker.model.analysismodel.trace.OperationCall Maven / Gradle / Ivy
/**
*/
package kieker.model.analysismodel.trace;
import java.time.Duration;
import java.time.Instant;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EObject;
import kieker.model.analysismodel.deployment.DeployedOperation;
/**
*
* A representation of the model object 'Operation Call'.
*
*
*
* The following features are supported:
*
*
* - {@link kieker.model.analysismodel.trace.OperationCall#getOperation Operation}
* - {@link kieker.model.analysismodel.trace.OperationCall#getParent Parent}
* - {@link kieker.model.analysismodel.trace.OperationCall#getChildren Children}
* - {@link kieker.model.analysismodel.trace.OperationCall#getDuration Duration}
* - {@link kieker.model.analysismodel.trace.OperationCall#getStart Start}
* - {@link kieker.model.analysismodel.trace.OperationCall#getDurRatioToParent Dur Ratio To Parent}
* - {@link kieker.model.analysismodel.trace.OperationCall#getDurRatioToRootParent Dur Ratio To Root Parent}
* - {@link kieker.model.analysismodel.trace.OperationCall#getStackDepth Stack Depth}
* - {@link kieker.model.analysismodel.trace.OperationCall#getOrderIndex Order Index}
* - {@link kieker.model.analysismodel.trace.OperationCall#isFailed Failed}
* - {@link kieker.model.analysismodel.trace.OperationCall#getFailedCause Failed Cause}
*
*
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall()
* @model
* @generated
*/
public interface OperationCall extends EObject {
/**
* Returns the value of the 'Operation' reference.
*
*
*
* @return the value of the 'Operation' reference.
* @see #setOperation(DeployedOperation)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_Operation()
* @model
* @generated
*/
DeployedOperation getOperation();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getOperation Operation}' reference.
*
*
*
* @param value
* the new value of the 'Operation' reference.
* @see #getOperation()
* @generated
*/
void setOperation(DeployedOperation value);
/**
* Returns the value of the 'Parent' reference.
* It is bidirectional and its opposite is '{@link kieker.model.analysismodel.trace.OperationCall#getChildren Children}'.
*
*
*
* @return the value of the 'Parent' reference.
* @see #setParent(OperationCall)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_Parent()
* @see kieker.model.analysismodel.trace.OperationCall#getChildren
* @model opposite="children"
* @generated
*/
OperationCall getParent();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getParent Parent}' reference.
*
*
*
* @param value
* the new value of the 'Parent' reference.
* @see #getParent()
* @generated
*/
void setParent(OperationCall value);
/**
* Returns the value of the 'Children' reference list.
* The list contents are of type {@link kieker.model.analysismodel.trace.OperationCall}.
* It is bidirectional and its opposite is '{@link kieker.model.analysismodel.trace.OperationCall#getParent Parent}'.
*
*
*
* @return the value of the 'Children' reference list.
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_Children()
* @see kieker.model.analysismodel.trace.OperationCall#getParent
* @model opposite="parent"
* @generated
*/
EList getChildren();
/**
* Returns the value of the 'Duration' attribute.
*
*
*
* @return the value of the 'Duration' attribute.
* @see #setDuration(Duration)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_Duration()
* @model dataType="kieker.model.analysismodel.Duration"
* @generated
*/
Duration getDuration();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getDuration Duration}' attribute.
*
*
*
* @param value
* the new value of the 'Duration' attribute.
* @see #getDuration()
* @generated
*/
void setDuration(Duration value);
/**
* Returns the value of the 'Start' attribute.
*
*
*
* @return the value of the 'Start' attribute.
* @see #setStart(Instant)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_Start()
* @model dataType="kieker.model.analysismodel.Instant"
* @generated
*/
Instant getStart();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getStart Start}' attribute.
*
*
*
* @param value
* the new value of the 'Start' attribute.
* @see #getStart()
* @generated
*/
void setStart(Instant value);
/**
* Returns the value of the 'Dur Ratio To Parent' attribute.
*
*
*
* @return the value of the 'Dur Ratio To Parent' attribute.
* @see #setDurRatioToParent(float)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_DurRatioToParent()
* @model
* @generated
*/
float getDurRatioToParent();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getDurRatioToParent Dur Ratio To Parent}' attribute.
*
*
*
* @param value
* the new value of the 'Dur Ratio To Parent' attribute.
* @see #getDurRatioToParent()
* @generated
*/
void setDurRatioToParent(float value);
/**
* Returns the value of the 'Dur Ratio To Root Parent' attribute.
*
*
*
* @return the value of the 'Dur Ratio To Root Parent' attribute.
* @see #setDurRatioToRootParent(float)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_DurRatioToRootParent()
* @model
* @generated
*/
float getDurRatioToRootParent();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getDurRatioToRootParent Dur Ratio To Root Parent}' attribute.
*
*
*
* @param value
* the new value of the 'Dur Ratio To Root Parent' attribute.
* @see #getDurRatioToRootParent()
* @generated
*/
void setDurRatioToRootParent(float value);
/**
* Returns the value of the 'Stack Depth' attribute.
*
*
*
* @return the value of the 'Stack Depth' attribute.
* @see #setStackDepth(int)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_StackDepth()
* @model
* @generated
*/
int getStackDepth();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getStackDepth Stack Depth}' attribute.
*
*
*
* @param value
* the new value of the 'Stack Depth' attribute.
* @see #getStackDepth()
* @generated
*/
void setStackDepth(int value);
/**
* Returns the value of the 'Order Index' attribute.
*
*
*
* @return the value of the 'Order Index' attribute.
* @see #setOrderIndex(int)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_OrderIndex()
* @model
* @generated
*/
int getOrderIndex();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getOrderIndex Order Index}' attribute.
*
*
*
* @param value
* the new value of the 'Order Index' attribute.
* @see #getOrderIndex()
* @generated
*/
void setOrderIndex(int value);
/**
* Returns the value of the 'Failed' attribute.
* The default value is "false"
.
*
*
*
* @return the value of the 'Failed' attribute.
* @see #setFailed(boolean)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_Failed()
* @model default="false"
* @generated
*/
boolean isFailed();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#isFailed Failed}' attribute.
*
*
*
* @param value
* the new value of the 'Failed' attribute.
* @see #isFailed()
* @generated
*/
void setFailed(boolean value);
/**
* Returns the value of the 'Failed Cause' attribute.
*
*
*
* @return the value of the 'Failed Cause' attribute.
* @see #setFailedCause(String)
* @see kieker.model.analysismodel.trace.TracePackage#getOperationCall_FailedCause()
* @model
* @generated
*/
String getFailedCause();
/**
* Sets the value of the '{@link kieker.model.analysismodel.trace.OperationCall#getFailedCause Failed Cause}' attribute.
*
*
*
* @param value
* the new value of the 'Failed Cause' attribute.
* @see #getFailedCause()
* @generated
*/
void setFailedCause(String value);
} // OperationCall
© 2015 - 2025 Weber Informatics LLC | Privacy Policy