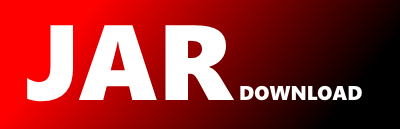
net.kut3.messaging.kafka.KafkaConsumerAdapter Maven / Gradle / Ivy
The newest version!
///*
// * Copyright 2019 Kut3Net.
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package net.kut3.messaging.kafka;
//
//import java.time.Duration;
//import java.util.Arrays;
//import java.util.Collection;
//import java.util.HashMap;
//import java.util.Map;
//import java.util.function.Consumer;
//import net.kut3.messaging.BatchConsumer;
//import org.apache.kafka.clients.consumer.ConsumerRecord;
//import org.apache.kafka.clients.consumer.ConsumerRecords;
//import net.kut3.messaging.Message;
//import static org.apache.kafka.clients.consumer.ConsumerConfig.*;
//
///**
// *
// * @param Type of key
// * @param Type of value
// */
//public abstract class KafkaConsumerAdapter {
//
// private final ConsumerBuilder builder;
//
// private KafkaConsumerAdapter(ConsumerBuilder builder) {
// this.builder = builder;
// }
//
// /**
// *
// * @param servers One or more host port pairs separated by commas.
// * @param groupId Name of the group this consumer belongs to.
// * @param clientId Name of this consumer.
// * @param topics Names of the consumed topics.
// * @return A new {@link ConsumerBuilder}
// */
// public static ConsumerBuilder newBuilder(String servers, String groupId,
// String clientId, Collection topics) {
//
// return new ConsumerBuilder(servers, groupId, clientId, topics);
// }
//
// /**
// *
// */
// public static final class ConsumerBuilder {
//
// private final Map props = new HashMap<>();
// private final Collection topics;
//
// private OffsetMode offsetMode = OffsetMode.LATEST;
//
// private ConsumerBuilder(String servers, String groupId, String clientId,
// Collection topics) {
//
// this.topics = topics;
//
// this.props.put(BOOTSTRAP_SERVERS_CONFIG, servers);
// this.props.put(GROUP_ID_CONFIG, groupId);
// this.props.put(CLIENT_ID_CONFIG, clientId);
//
// this.props.put(ENABLE_AUTO_COMMIT_CONFIG, "true");
// this.props.put(AUTO_COMMIT_INTERVAL_MS_CONFIG, "3000");
//
// this.props.put(KEY_DESERIALIZER_CLASS_CONFIG,
// "org.apache.kafka.common.serialization.StringDeserializer");
//
// this.props.put(VALUE_DESERIALIZER_CLASS_CONFIG,
// "org.apache.kafka.common.serialization.StringDeserializer");
// }
//
// /**
// * Default value is {@link OffsetMode#LATEST}.
// *
// * @return Indicate this consumer in which offset mode
// */
// public OffsetMode offsetMode() {
// return this.offsetMode;
// }
//
// /**
// *
// * @param value One of {@link OffsetMode} value. Default value is
// * {@link OffsetMode#LATEST}.
// * @return The current {@link ConsumerBuilder} instance
// */
// public ConsumerBuilder offsetMode(OffsetMode value) {
// this.offsetMode = value;
// return this;
// }
//
// /**
// *
// * @return a new the current {@link KafkaConsumerAdapter}
// */
// public KafkaConsumerAdapter build() {
// return new KafkaConsumerAdapter<>(this);
// }
// }
//
// /**
// *
// * @param messageProcessor The processor will process delivered message
// */
// public void start(Consumer messageProcessor) {
// this.builder.props.put(AUTO_OFFSET_RESET_CONFIG,
// this.builder.offsetMode().asString());
//
// org.apache.kafka.clients.consumer.KafkaConsumer consumer
// = new org.apache.kafka.clients.consumer.KafkaConsumer<>(this.builder.props);
//
// consumer.subscribe(this.builder.topics);
//
// while (true) {
// ConsumerRecords records
// = consumer.poll(Duration.ofMillis(10000));
//
// for (ConsumerRecord record : records) {
// messageProcessor.accept(new KafkaMessageAdapter(record));
// }
// }
// }
//
// /**
// *
// * @param messageProcessor The processor will process delivered message
// */
// public void start(BatchConsumer messageProcessor) {
// this.builder.props.put(AUTO_OFFSET_RESET_CONFIG,
// this.builder.offsetMode().asString());
//
// org.apache.kafka.clients.consumer.KafkaConsumer consumer
// = this.initConsumer(this.builder.props);
//
// consumer.subscribe(this.builder.topics);
//
// while (true) {
// ConsumerRecords records
// = consumer.poll(Duration.ofMillis(10000));
//
// if (records.isEmpty()) {
// System.out.println("No records pooled after 10000ms");
// continue;
// }
//
// for (ConsumerRecord record : records) {
// messageProcessor.addToBatch(new KafkaMessageAdapter(record));
// }
//
// messageProcessor.processBatch();
// }
// }
//
// @Override
// public String toString() {
// return "{topics=" + this.builder.topics
// + "}";
// }
//
// /**
// *
// * @param args Input parameters
// */
// public static void main(String[] args) {
// KafkaConsumerAdapter consumer = KafkaConsumerAdapter
// .newBuilder("10.1.1.99:9092,10.1.1.99:9093,10.1.1.98:9094",
// "group-01",
// "consumer-001",
// Arrays.asList("my-topic"))
// .offsetMode(OffsetMode.EARLIEST)
// .build();
//
// consumer.start(m -> System.out.println(m));
// }
//
// /**
// *
// * @param consumerProperties Properties of consumer to initialize
// * @return A appropriate
// * {@link org.apache.kafka.clients.consumer.KafkaConsumer} instance.
// */
// protected abstract org.apache.kafka.clients.consumer.KafkaConsumer
// initConsumer(Map consumerProperties);
//}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy