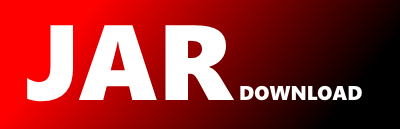
net.kut3.messaging.kafka.client.ProducerImpl Maven / Gradle / Ivy
/*
* Copyright 2019 Kut3Net.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.kut3.messaging.kafka.client;
import ch.qos.logback.classic.Level;
import java.util.Date;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import net.kut3.messaging.Message;
import net.kut3.messaging.ProcessResultCode;
import net.kut3.messaging.ProduceResult;
import net.kut3.messaging.Producer;
import net.kut3.messaging.client.ClientFactory;
import net.kut3.messaging.kafka.Component;
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerRecord;
import org.slf4j.LoggerFactory;
/**
*
*/
class ProducerImpl implements Producer, Component {
private final String name;
private final KafkaProducer producer;
private final String topic;
private final OnErrorHandler onErrorHandler;
/**
*
* @param name Name of the producer
* @param props Kafka producer properties
* @param topic Topic to produce message to
* @param onErrorHandler Error handler
*/
ProducerImpl(String name, Map props,
String topic,
OnErrorHandler onErrorHandler) {
this.name = name;
this.producer = new KafkaProducer<>(props);
this.topic = topic;
this.onErrorHandler = onErrorHandler;
}
@Override
public String name() {
return this.name;
}
@Override
public ProduceResult produce(Message message) {
if (null == this.onErrorHandler) {
this.producer.send(new ProducerRecord(this.topic,
message.property(KEY), message.bodyAsString()));
} else {
this.producer.send(new ProducerRecord(this.topic,
message.property(KEY), message.bodyAsString()),
(r, e) -> {
if (!r.hasOffset() || null != e) {
this.onErrorHandler.handle(this.topic, message, e);
}
}
);
}
return new ProduceResult().code(ProcessResultCode.OK);
}
@Override
public void close() {
System.out.println("closing");
this.producer.close();
}
public static void main(String[] args) throws InterruptedException {
ClientFactory clientFactory = new KafkaClientFactory();
String producerName = "kafka-client-0.3.0-01";
String servers = "10.1.1.99:9092,10.1.1.99:9093,10.1.1.98:9094";
String topic = "dev.Merchant";
((ch.qos.logback.classic.Logger) LoggerFactory.getLogger("org.apache.kafka"))
.setLevel(Level.ERROR);
LoggerFactory.getLogger(topic).info("Begin");
Producer producer = clientFactory.newProducer(new SimpleProducerProperties(producerName,
servers, topic,
(t, m, ex) -> {
LoggerFactory.getLogger(t).error(m.toString());
LoggerFactory.getLogger(t).error("shit", ex);
}));
for (int i = 0; i < 1000; i++) {
try {
System.out.println(new Date());
System.out.println(producer.produce(new KafkaMessage("key" + i, "value" + i)));
System.out.println(new Date());
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException ex) {
}
}
producer.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy