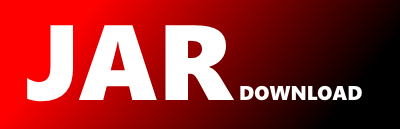
net.leanix.dropkit.persistence.TransactionHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-dropkit-persistence Show documentation
Show all versions of leanix-dropkit-persistence Show documentation
Base functionality for persistence in leanIX dropwizard-based services
package net.leanix.dropkit.persistence;
import com.google.inject.Inject;
import com.google.inject.Injector;
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.concurrent.Callable;
import java.util.concurrent.Executors;
/**
*
* Just a convenient class to simplify the execution of code within a hibernate transaction.
*
*
* @author ralfwehner
*
*/
public class TransactionHandler {
private static final Logger LOG = LoggerFactory.getLogger(TransactionHandler.class);
private SessionHandler sessionHandler;
@Inject
public TransactionHandler(SessionHandler sessionHandler) {
super();
this.sessionHandler = sessionHandler;
}
/**
* A constructor which is mainly useful in junit tests.
*
* @param injector
*/
public TransactionHandler(Injector injector) {
this(injector.getInstance(SessionHandler.class));
}
public K executeInTransaction(Callable callable) {
Session session = sessionHandler.openSession();
Transaction transaction = session.beginTransaction();
boolean success = false;
K result = null;
try {
result = callable.call();
success = true;
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
if (success) {
try {
transaction.commit();
} catch (Throwable t) {
LOG.error("can not commit transaction", t);
} finally {
// close session anyway to avoid db connection leaks
session.close();
}
} else {
try {
transaction.rollback();
} catch (Throwable t) {
LOG.error("can not rollback transaction", t);
} finally {
// if you make rollback, clear is needed
session.clear();
// close session anyway to avoid db connection leaks
session.close();
}
}
}
return result;
}
public void executeInTransaction(Runnable runnable) {
executeInTransaction(Executors.callable(runnable));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy