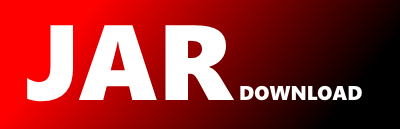
net.leanix.dropkit.persistence.pool.HikariDataSourceFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-dropkit-persistence Show documentation
Show all versions of leanix-dropkit-persistence Show documentation
Base functionality for persistence in leanIX dropwizard-based services
package net.leanix.dropkit.persistence.pool;
import com.codahale.metrics.MetricRegistry;
import com.zaxxer.hikari.HikariConfig;
import io.dropwizard.db.DataSourceFactory;
import io.dropwizard.db.ManagedDataSource;
import io.dropwizard.util.Duration;
import java.util.Map;
import javax.validation.constraints.NotNull;
import java.util.Properties;
import javax.validation.constraints.Null;
/**
* A factory for pooled {@link ManagedDataSource}s.
*
* Configuration Parameters:
*
*
* Name
* Default
* Description
*
*
* {@code driverClass}
* REQUIRED
* The full name of the JDBC driver class.
*
*
* {@code driverClass}
* REQUIRED
* The full name of the DataSource class.
*
*
* {@code url}
* REQUIRED
* The URL of the server.
*
*
* {@code user}
* REQUIRED
* The username used to connect to the server.
*
*
* {@code password}
* none
* The password used to connect to the server.
*
*
* {@code abandonWhenPercentageFull}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code alternateUsernamesAllowed}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code commitOnReturn}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code autoCommitByDefault}
* JDBC driver's default
* The default auto-commit state of the connections.
*
*
* {@code readOnlyByDefault}
* JDBC driver's default
* The default read-only state of the connections.
*
*
* {@code properties}
* none
* Any additional JDBC driver parameters.
*
*
* {@code defaultCatalog}
* none
* The default catalog to use for the connections.
*
*
* {@code defaultTransactionIsolation}
* JDBC driver default
*
* The default transaction isolation to use for the connections. Can be one of
* {@code none}, {@code default}, {@code read-uncommitted}, {@code read-committed},
* {@code repeatable-read}, or {@code serializable}.
*
*
*
* {@code useFairQueue}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code initialSize}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code minSize}
* 10
*
* The minimum size of the connection pool. Note, it's recommended to make this equal to the maximum pool size.
*
*
*
* {@code maxSize}
* 100
*
* The maximum size of the connection pool.
*
*
*
* {@code initializationQuery}
* none
*
* A custom query to be run when a connection is first created.
*
*
*
* {@code logAbandonedConnections}
* {@code false}
*
* This property is not supported with HikariCP.
*
*
*
* {@code logValidationErrors}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code maxConnectionAge}
* 30 Minutes
*
* Connections which have been open for longer than {@code maxConnectionAge} are
* closed when returned.
*
*
*
* {@code maxWaitForConnection}
* 30 seconds
*
* If a request for a connection is blocked for longer than this period, an exception
* will be thrown.
*
*
*
* {@code minIdleTime}
* 1 minute
*
* The minimum amount of time an connection must sit idle in the pool before it is
* eligible for eviction.
*
*
*
* {@code jdbc4Driver}
* {@code true}
*
* If true, will not use the validationQuery option below, if your driver is old, time to upgrade,
* or be sad and set this to false.
*
*
*
* {@code validationQuery}
* {@code SELECT 1}
*
* This feature is not recommended if you have a JDBC driver version 4 or newer.
*
* The SQL query that will be used to validate connections from this pool before
* returning them to the caller or pool. If specified, this query does not have to
* return any data, it just can't throw a SQLException.
*
*
*
* {@code validationQueryTimeout}
* 5000 ms
*
* This property is used to control how long HikariCP will wait to test for aliveness, minimum 1 second.
*
*
*
* {@code checkConnectionWhileIdle}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code checkConnectionOnBorrow}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code checkConnectionOnConnect}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code checkConnectionOnReturn}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code autoCommentsEnabled}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code evictionInterval}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
* {@code validationInterval}
* N/A
*
* This property is not supported with HikariCP.
*
*
*
*/
public final class HikariDataSourceFactory extends DataSourceFactory {
public boolean jdbc4Driver = false;
@NotNull
public String dataSourceClassName;
public String poolName;
@Null
private String driverClass = null;
private String url = null;
@Override
public ManagedDataSource build(MetricRegistry metricRegistry, String name) {
final Properties properties = new Properties();
for (Map.Entry property : this.getProperties().entrySet()) {
if (property.getKey().startsWith("dataSource."))
properties.setProperty(property.getKey(), property.getValue());
}
final HikariConfig config = new HikariConfig(properties);
// config.setJdbcUrl(null);
config.setUsername(getUser());
config.setPassword(getPassword());
if (getAutoCommitByDefault() != null)
config.setAutoCommit(getAutoCommitByDefault());
if (getReadOnlyByDefault() != null)
config.setReadOnly(getReadOnlyByDefault());
config.setCatalog(getDefaultCatalog());
String isolationLevel = null;
switch (getDefaultTransactionIsolation()) {
case NONE:
isolationLevel = "TRANSACTION_NONE";
break;
case READ_UNCOMMITTED:
isolationLevel = "TRANSACTION_READ_UNCOMMITTED";
break;
case READ_COMMITTED:
isolationLevel = "TRANSACTION_READ_COMMITTED";
break;
case REPEATABLE_READ:
isolationLevel = "TRANSACTION_REPEATABLE_READ";
break;
case SERIALIZABLE:
isolationLevel = "TRANSACTION_SERIALIZABLE";
break;
}
if (isolationLevel != null)
config.setTransactionIsolation(isolationLevel);
config.setMaximumPoolSize(getMaxSize());
config.setConnectionInitSql(getInitializationQuery());
config.setMaxLifetime(getMaxConnectionAge().orElse(Duration.minutes(30)).toMilliseconds());
config.setConnectionTimeout(getMaxWaitForConnection().toMilliseconds());
config.setValidationTimeout(Duration.seconds(1).toMilliseconds());
config.setMetricRegistry(metricRegistry);
config.setDataSourceClassName(dataSourceClassName);
if (this.poolName != null)
config.setPoolName(poolName);
return new ManagedHikariDataSource(config);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy