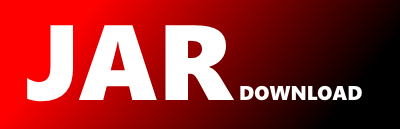
net.leanix.dropkit.util.TimeoutExecutor Maven / Gradle / Ivy
package net.leanix.dropkit.util;
import java.util.concurrent.Callable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class TimeoutExecutor {
private static final Logger LOG = LoggerFactory.getLogger(TimeoutExecutor.class);
private final long timeout;
private final long sleeping;
public TimeoutExecutor(long timeout, long sleeping) {
this.timeout = timeout;
this.sleeping = sleeping;
}
public boolean checkUntilConditionIsTrue(Callable callable) {
long end = System.currentTimeMillis() + timeout;
try {
while (System.currentTimeMillis() < end) {
if (callable.call() == true) {
return true;
}
Thread.sleep(sleeping);
}
LOG.warn("Skip condition testing due to timeout!");
} catch (InterruptedException e) {
LOG.warn("stop checking conditions", e);
} catch (Exception e) {
LOG.warn("Got exception during checking the condition.", e);
}
return false;
}
public T callUntilReturnIsNotNull(Callable callable) {
long end = System.currentTimeMillis() + timeout;
try {
while (System.currentTimeMillis() < end) {
T result = callable.call();
if (result != null) {
return result;
}
Thread.sleep(sleeping);
}
} catch (InterruptedException e) {
LOG.warn("stop checking conditions", e);
} catch (Exception e) {
LOG.warn("Got exception during checking the condition.", e);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy