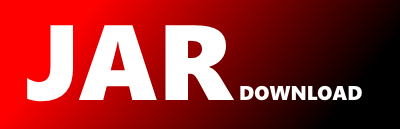
net.leanix.dropkit.AppHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-dropkit Show documentation
Show all versions of leanix-dropkit Show documentation
Base functionality for leanIX dropwizard-based services
package net.leanix.dropkit;
import net.leanix.dropkit.swagger.ApiDocsAssetServlet;
import com.codahale.metrics.MetricFilter;
import com.fasterxml.jackson.databind.util.ISO8601DateFormat;
import com.fasterxml.jackson.datatype.joda.JodaModule;
import com.google.common.base.Charsets;
import com.palominolabs.metrics.newrelic.AllEnabledMetricAttributeFilter;
import com.palominolabs.metrics.newrelic.NewRelicReporter;
import io.dropwizard.Configuration;
import io.dropwizard.assets.AssetsBundle;
import io.dropwizard.configuration.EnvironmentVariableSubstitutor;
import io.dropwizard.configuration.SubstitutingSourceProvider;
import io.dropwizard.setup.Bootstrap;
import io.dropwizard.setup.Environment;
import org.eclipse.jetty.servlets.CrossOriginFilter;
import java.util.EnumSet;
import java.util.concurrent.TimeUnit;
import javax.servlet.DispatcherType;
import javax.servlet.FilterRegistration;
/**
* Application initialisation helper.
*
*/
public class AppHelper {
/**
* Inits the use of iso8501 time format.
*
* @param bootstrap
*/
public void initializeISO8601(Bootstrap extends Configuration> bootstrap) {
bootstrap.getObjectMapper().registerModule(new JodaModule());
bootstrap.getObjectMapper().setDateFormat(new ISO8601DateFormat());
}
public void initialize(Bootstrap extends Configuration> bootstrap) {
// Enable variable substitution with environment variables
bootstrap.setConfigurationSourceProvider(
new SubstitutingSourceProvider(
bootstrap.getConfigurationSourceProvider(),
new EnvironmentVariableSubstitutor(false)));
this.initializeISO8601(bootstrap);
}
/**
* To be included in App.run() method
* @param config
* @param environment
*/
public void run(Configuration config, Environment environment) {
this.addCORSFilter(environment);
this.registerExceptionMappers(environment);
this.registerNewRelicReporter(environment);
}
/**
* To be included in App.run() method for serving swagger.json and swagger UI.
* @param config
* @param environment
*/
public void runSwagger(Configuration config, Environment environment) {
// add the servlet for static swagger UI assets (need to put leanix-swagger-ui into the classpath)
new AssetsBundle("/swagger", "/docs", "index.html", "swagger").run(environment);
// add the servlet serving the swagger definitions file (swagger.json)
// swagger.json must be generated at service build time (with kong chen plugin)
environment.servlets().addServlet(
"api-docs",
new ApiDocsAssetServlet("/swagger-assets", "/api-docs", "swagger.json", Charsets.UTF_8, "configureMeIfNeeded")
).addMapping("/api-docs/*");
}
/**
* Registers mappers for invalid-entity and business logic exception.
*
*
* @param environment
*/
public void registerExceptionMappers(Environment environment) {
//exception mappers
environment.jersey().register(new InvalidEntityMapper());
environment.jersey().register(new BusinessLogicExceptionMapper());
}
/**
* Registers new relic reporter
* @param environment
*/
public void registerNewRelicReporter(Environment environment)
{
NewRelicReporter reporter = new NewRelicReporter(
environment.metrics(),
"NewRelic reporter",
MetricFilter.ALL,
new AllEnabledMetricAttributeFilter(),
TimeUnit.SECONDS,
TimeUnit.MILLISECONDS,
""
);
reporter.start(1, TimeUnit.MINUTES);
}
/**
* Enables cross-origin requests.
*
*
* @param environment
*/
public void addCORSFilter(Environment environment) {
FilterRegistration.Dynamic filter = environment.servlets().addFilter("CORS", CrossOriginFilter.class);
filter.addMappingForUrlPatterns(EnumSet.allOf(DispatcherType.class), true, "/*");
filter.setInitParameter(CrossOriginFilter.ALLOWED_ORIGINS_PARAM, "*");
filter.setInitParameter(CrossOriginFilter.ACCESS_CONTROL_ALLOW_ORIGIN_HEADER, "*");
filter.setInitParameter(CrossOriginFilter.ALLOWED_HEADERS_PARAM, "accept,accept-encoding,accept-language,access-control-request-headers,access-control-request-method,api-key,authorization,cache-control,content-type,content-length,connection,host,pragma,referer,x-requested-with,origin,x-api-sync-mode,x-api-key,x-credentials");
filter.setInitParameter(CrossOriginFilter.ALLOWED_METHODS_PARAM, "GET,PUT,POST,DELETE,OPTIONS");
filter.setInitParameter(CrossOriginFilter.PREFLIGHT_MAX_AGE_PARAM, "5184000"); // 2 months
filter.setInitParameter(CrossOriginFilter.ALLOW_CREDENTIALS_PARAM, "true");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy