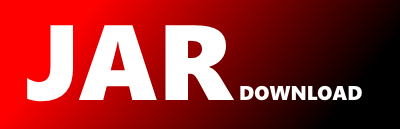
net.leanix.dropkit.amqp.ConnectionHolder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-dropkit Show documentation
Show all versions of leanix-dropkit Show documentation
Base functionality for leanIX dropwizard-based services
package net.leanix.dropkit.amqp;
import com.google.inject.Inject;
import com.google.inject.Singleton;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
import com.rabbitmq.client.ShutdownSignalException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
/**
* Holds and manages a connection to an AMQP server. When the connection drops,
* a new one may be created and put here.
*/
@Singleton
public class ConnectionHolder {
private final Logger log = LoggerFactory.getLogger(ConnectionHolder.class);
private final ConnectionFactory connectionFactory;
private volatile Connection connection;
@Inject
public ConnectionHolder(ConnectionFactory connectionFactory) {
this.connectionFactory = connectionFactory;
}
public Connection getConnection() throws IOException {
if (connection == null) {
synchronized (this) {
if (connection == null) {
log.info("creating a connection to AMQP server");
connection = connectionFactory.newConnection();
}
}
}
return connection;
}
public void closeConnection() {
if (connection == null) {
// no connection to close
return;
}
Connection oldConnection = connection;
connection = null;
log.info("closing connection");
try {
oldConnection.close();
} catch (IOException | ShutdownSignalException e) {
log.error("could not close connection, ignore", e);
}
}
public Channel createNewChannel() throws IOException {
if (connection == null) {
getConnection();
}
try {
log.debug("..createChannel");
return connection.createChannel();
} catch (IOException | ShutdownSignalException e) {
log.error("could not create channel from connection -- closing connection", e);
closeConnection();
throw e;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy