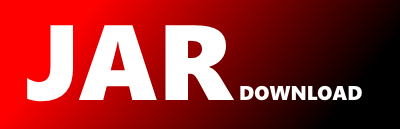
net.leanix.dropkit.api.Payload Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-dropkit Show documentation
Show all versions of leanix-dropkit Show documentation
Base functionality for leanIX dropwizard-based services
package net.leanix.dropkit.api;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.dropwizard.jackson.Jackson;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Represents a generic map-like payload class.
*
*
*/
@JsonInclude(JsonInclude.Include.ALWAYS)
public class Payload {
private static final ObjectMapper MAPPER = Jackson.newObjectMapper();
private final Map fields = new HashMap<>();
public static Payload fromObject(Object o) {
if (o == null) {
return new Payload();
}
String encoded;
try {
encoded = MAPPER.writeValueAsString(o);
} catch (JsonProcessingException ex) {
Logger.getLogger(Payload.class.getName()).log(Level.WARNING, "Transformation from object failed.", ex);
return new Payload();
}
return fromString(encoded);
}
public static Payload fromString(String encoded) {
try {
return MAPPER.readValue(encoded, Payload.class);
} catch (IOException ex) {
Logger.getLogger(Payload.class.getName()).log(Level.WARNING, "Deserialization from string failed", ex);
return new Payload();
}
}
@Override
public String toString() {
try {
return MAPPER.writeValueAsString(toMap());
} catch (JsonProcessingException ex) {
Logger.getLogger(Payload.class.getName()).log(Level.WARNING, "Serialization to string failed", ex);
return null;
}
}
/**
* Returns the internal map.
*
* @return
*/
public Map toMap() {
return fields;
}
/**
* Setter.
*
* @param key
* @param value
*/
@JsonAnySetter
public void setKey(String key, Object value) {
fields.put(key, value);
}
/**
* Getter.
*
* @param key
* @return
*/
@JsonAnyGetter
public Object getKey(String key) {
if (!fields.containsKey(key)) {
return null;
}
return fields.get(key);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy