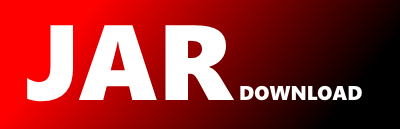
net.leanix.dropkit.etcd.MinimalEtcdClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-dropkit Show documentation
Show all versions of leanix-dropkit Show documentation
Base functionality for leanIX dropwizard-based services
package net.leanix.dropkit.etcd;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
import javax.ws.rs.client.Client;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.client.Entity;
import javax.ws.rs.client.Invocation;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.Form;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import org.glassfish.jersey.client.ClientConfig;
import org.glassfish.jersey.client.ClientProperties;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MinimalEtcdClientImpl implements MinimalEtcdClient {
private final Logger LOG = LoggerFactory.getLogger(MinimalEtcdClientImpl.class);
private final Client client;
private final URI etcdUri;
public MinimalEtcdClientImpl(URI etcdUri) {
this.etcdUri = etcdUri;
ClientConfig clientConfig = new ClientConfig();
clientConfig.property(ClientProperties.READ_TIMEOUT, 120 * 1000);
clientConfig.property(ClientProperties.CONNECT_TIMEOUT, 10 * 1000);
client = ClientBuilder.newClient(clientConfig);
}
@Override
public URI getEtcdUri() {
return etcdUri;
}
@Override
public String get(String key) throws EtcdException {
WebTarget webTarget = client.target(etcdUri)
.path("v2/keys")
.path(key);
Invocation.Builder invocationBuilder = webTarget.request(MediaType.APPLICATION_JSON);
try {
Response response = invocationBuilder.get();
if (response.getStatus() != Response.Status.OK.getStatusCode()
&& response.getStatus() != Response.Status.NOT_FOUND.getStatusCode()) {
throw new EtcdException(1, null, String.format("can not read key '%s' from etcd uri '%s'. Status code: %s",
key, etcdUri, response.getStatus()), 1, key, null);
}
EtcdResponse result = response.readEntity(EtcdResponse.class);
return result.errorCode == 100 ? null : result.getNode().getValue();
} catch (javax.ws.rs.ProcessingException e) {
throw new EtcdException(1, null, String.format("can not read key '%s' from etcd uri '%s'.", key, etcdUri), 1, key, e);
}
}
@Override
public boolean exists(String key) throws EtcdException {
WebTarget webTarget = client.target(etcdUri)
.path("v2/keys")
.path(key);
Invocation.Builder invocationBuilder = webTarget.request(MediaType.APPLICATION_JSON);
try {
Response response = invocationBuilder.get();
if (response.getStatus() != Response.Status.OK.getStatusCode()
&& response.getStatus() != Response.Status.NOT_FOUND.getStatusCode()) {
throw new EtcdException(1, null, String.format("can not read key '%s' from etcd uri '%s'. Status code: %s",
key, etcdUri, response.getStatus()), 1, key, null);
}
EtcdResponse result = response.readEntity(EtcdResponse.class);
if (result.errorCode != 100 && result.getNode().getKey() != null) {
return true;
}
return false;
} catch (javax.ws.rs.ProcessingException e) {
throw new EtcdException(1, null, String.format("can not read key '%s' from etcd uri '%s'.", key, etcdUri), 1, key, e);
}
}
@Override
public List list(String key) throws EtcdException {
WebTarget webTarget = client.target(etcdUri)
.path("v2/keys")
.path(key);
Invocation.Builder invocationBuilder = webTarget.request(MediaType.APPLICATION_JSON);
try {
Response response = invocationBuilder.get();
if (response.getStatus() != Response.Status.OK.getStatusCode()
&& response.getStatus() != Response.Status.NOT_FOUND.getStatusCode()) {
throw new EtcdException(1, null, String.format("can not read key '%s' from etcd uri '%s'. Status code: %s",
key, etcdUri, response.getStatus()), 1, key, null);
}
EtcdResponse result = response.readEntity(EtcdResponse.class);
List list = new ArrayList<>();
if (result.errorCode != 100 && result.getNode() != null && result.getNode().getNodes() != null) {
for (EtcdResponse.Node node : result.getNode().getNodes()) {
list.add(node.getKey());
}
}
return list;
} catch (javax.ws.rs.ProcessingException e) {
throw new EtcdException(1, null, String.format("can not read key '%s' from etcd uri '%s'.", key, etcdUri), 1, key, e);
}
}
@Override
public void set(String key, String value) throws EtcdException {
WebTarget webTarget = client.target(etcdUri)
.path("v2/keys")
.path(key);
Form form = new Form();
form.param("value", value);
Invocation.Builder invocationBuilder = webTarget.request(MediaType.APPLICATION_JSON_TYPE);
try {
Response response = invocationBuilder.put(Entity.entity(form, MediaType.APPLICATION_FORM_URLENCODED_TYPE));
if (response.getStatusInfo().getFamily() != Response.Status.Family.SUCCESSFUL
&& response.getStatus() != Response.Status.NOT_FOUND.getStatusCode()) {
LOG.warn("Error while putting value '{}' to key '{}' on etcd. Status code: {}", value, key, response.getStatus());
throw new EtcdException(1, response.getEntity().toString(), "received HTTP " + response.getStatus(), 1, key, null);
}
} catch (javax.ws.rs.ProcessingException e) {
LOG.warn("Error while putting '{}' key on etcd with uri '{}'", key, etcdUri);
throw new EtcdException(1, null, String.format("can not write key '%s' from etcd uri '%s'.", key, etcdUri), 1, key, e);
}
}
@Override
public void delete(String key, boolean recursive) throws EtcdException {
WebTarget webTarget = client.target(etcdUri)
.path("v2/keys")
.path(key);
if (recursive) {
webTarget = webTarget.queryParam("recursive", "true");
}
Invocation.Builder invocationBuilder = webTarget.request(MediaType.APPLICATION_JSON_TYPE);
try {
Response response = invocationBuilder.delete();
if (response.getStatusInfo().getFamily() != Response.Status.Family.SUCCESSFUL) {
throw new EtcdException(1, null, String.format("can not delete key '%s' from etcd uri '%s'. Status code: %s",
key, etcdUri, response.getStatus()), 1, key, null);
}
} catch (javax.ws.rs.ProcessingException e) {
throw new EtcdException(1, null, String.format("can not delete key '%s' from etcd uri '%s'.", key, etcdUri), 1, key, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy